Modify the code below to create a "Yahtzee Dice game" program that is contained in 5 files: Dice_game.cpp
Modify the code below to create a "Yahtzee Dice game" program that is contained in 5 files:
- Dice_game.cpp
- Dice.cpp
- Die.cpp
- Dice.h
- Die.h
ou must include these features:
- Try-Throw-Catch
- Use .h and .cpp files for classes
- Must be your own code written now
code to be modified:
Dice_Game.cpp
#include <iostream>
#include "Die.h"
using namespace std;
// a struct for game variables
struct GameState
{
int turn = 1;
int score = 0;
int score_this_turn = 0;
bool turn_over = false;
bool game_over = false;
Die die;
};
// declare functions
void display_rules();
void play_game(GameState&);
void take_turn(GameState&);
void roll_die(GameState&);
void hold_turn(GameState&);
int main()
{
display_rules();
GameState game;
play_game(game);
}
// define functions
void display_rules()
{
cout << "Dice Game Rules: \n"
<< "\n"
<< "* See how many turns it takes you to get to 20.\n"
<< "* Turn ends when you hold or roll a 1.\n"
<< "* If you roll a 1, you lose all points for the turn.\n"
<< "* If you hold, you save all points for the turn.\n\n";
}
void play_game(GameState& game)
{
while (!game.game_over)
{
take_turn(game);
}
cout << "Game over!\n";
}
void take_turn(GameState& game)
{
cout << "TURN " << game.turn << endl;
game.turn_over = false;
while (!game.turn_over)
{
char choice;
cout << "Roll or hold? (r/h): ";
cin >> choice;
if (choice == 'r')
roll_die(game);
else if (choice == 'h')
hold_turn(game);
else
cout << "Invalid choice. Try again.\n";
}
}
void roll_die(GameState& game)
{
game.die.roll();
cout << "Die: " << game.die.getValue() << endl;
if (game.die.getValue() == 1)
{
game.score_this_turn = 0;
game.turn += 1;
game.turn_over = true;
cout << "Turn over. No score.\n\n";
}
else
{
game.score_this_turn += game.die.getValue();
}
}
void hold_turn(GameState& game)
{
game.score += game.score_this_turn;
game.turn_over = true;
cout << "Score for turn: " << game.score_this_turn << endl;
cout << "Total score: " << game.score << "\n\n";
game.score_this_turn = 0;
if (game.score >= 20)
{
game.game_over = true;
cout << "You finished in " << game.turn << " turns!\n\n";
}
else
{
game.turn += 1;
}
}
Dice.cpp
#include "Dice.h"
Dice::Dice() {}
void Dice::add_die(Die die)
{
dice.push_back(die);
}
void Dice::roll_all()
{
for (Die& die : dice)
{
die.roll();
}
}
std::
{
return dice;
}
Die.cpp
#include <cstdlib>
#include <ctime>
#include "Die.h"
Die::Die()
{
srand(time(NULL)); // seed the rand() function
value = 1;
}
void Die::roll()
{
value = rand() % 6; // value is >= 0 and <= 5
++value; // value is >= 1 and <= 6
}
int Die::getValue()
{
return value;
}
Dice.h
ifndef DICE_H
#define DICE_H
#include <vector>
#include "Die.h"
class Dice
{
private:
std::vector<Die> dice;
public:
Dice();
void add_die(Die die);
void roll_all();
std::vector<Die> get_dice() const;
};
#endif // DICE_H
Die.h
#ifndef DIE_H
#define DIE_H
class Die
{
private:
int value;
public:
Die();
void roll();
int getValue();
};
#endif

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

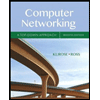
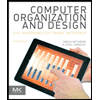
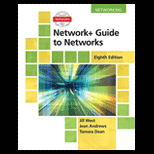
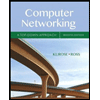
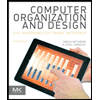
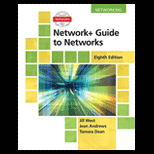
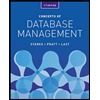
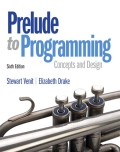
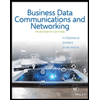