main.cc file #include #include "car.h" int main() { // =================== YOUR CODE HERE =================== // 1. Create a Car object called `c1` using the default // constructor. // Call its Print member function. // ====================================================== std::cout << "\n"; // =================== YOUR CODE HERE =================== // 2. Create a `VehicleId` object with the following info: // Model: Honda, ID: 3, License plate: 7B319X4 // Create a `Car` object `c2` using the constructor that // accepts a `VehicleId` and pass in the `VehicleId` // object you just made. // Call its Print member function. // ====================================================== std::cout << "\n"; // =================== YOUR CODE HERE =================== // 3. Create a `Date` object with the following info: // Day: 4, Month: 11, Year: 2018 // Create a `Car` object `c3` using the constructor that // accepts a `Date` object and pass in the `Date` object // you just made. // Call its Print member function. // ====================================================== std::cout << "\n"; // =================== YOUR CODE HERE =================== // 4. Create a `Car` object `c4` using the constructor that // accepts a `VehicleId` and `Date` object and pass in // the `VehicleId` and `Date` objects you created in // steps 2 and 3 above. // Call its Print member function. // ====================================================== // 5. Create an instance of `VehicleId` using the default constructor. // 6. Create an instance of `Date` using the default constructor. // 7. Call the `SetId` member function on `c4` and pass // in the VehicleId you just created. // 8. Call the `SetReleaseDate` member function on `c4` // and pass in the VehicleId you just created. // 9. Finally, call the print member function for `c4`. return 0; } date.h file class Date { public: Date() : Date(1, 1, 2022) {} Date(int day, int month, int year) : day_(day), month_(month), year_(year) {} int Day() const { return day_; } void SetDay(int day) { day_ = day; } int Month() const { return month_; } void SetMonth(int month) { month_ = month; } int Year() const { return year_; } void SeyYear(int year) { year_ = year; } private: int day_; int month_; int year_; }; car.cc file #include "car.h" #include // ========================= YOUR CODE HERE ========================= // This implementation file (car.cc) is where you should implement // the member functions declared in the header (car.h), only // if you didn't implement them inline within car.h. // // Remember to specify the name of the class with :: in this format: // MyClassName::MyFunction() { // ... // } // to tell the compiler that each function belongs to the Car class. // =================================================================== car.h file #include "date.h" #include "vehicleid.h" // ======================= YOUR CODE HERE ======================= // Write the Car class here, containing two member variables: // id_ to store the vehicle identity info and release_date_ // to store the car's release date. // // Then, define the following constructor overloads: // 1. A default constructor, left empty. The id_ and release_date // will be initialized to their default values automatically. // 2. A non-default constructor, which accepts a VehicleId object. // 3. A non-default constructor, which accepts a Date object. // 4. A non-default constructor, which accepts a VehicleId and Date // object (in that order). // // Finally, define the following member functions: // 1. The accessor (getter) function for the id_. // 2. The mutator (setter) function for the id_. // 3. The accessor (getter) function for the release_date_. // 4. The mutator (setter) function for the release_date_. // 5. The Print member function. // // Note: mark functions that do not modify the member variables // as const, by writing `const` after the parameter list. // Pass objects by const reference, e.g. for the Date object // passed in as input to SetReleaseDate. // =============================================================== vehicleid.h file #include class VehicleId { public: VehicleId() : VehicleId("Tesla", 121, "TUFFY121L") {} VehicleId(const std::string &model, int vin, const std::string &license_plate) : model_(model), vin_(vin), license_plate_(license_plate) {} int Vin() const { return vin_; } void SetVin(int vin) { vin_ = vin; } std::string Model() const { return model_; } void SetModel(const std::string &model) { model_ = model; } std::string LicensePlate() const { return license_plate_; } void SetLicensePlate(const std::string &license_plate) { license_plate_ = license_plate; } private: // A vehicle identification number (VIN) int vin_; std::string model_; std::string license_plate_; };
main.cc file
#include <iostream>
#include "car.h"
int main() {
// =================== YOUR CODE HERE ===================
// 1. Create a Car object called `c1` using the default
// constructor.
// Call its Print member function.
// ======================================================
std::cout << "\n";
// =================== YOUR CODE HERE ===================
// 2. Create a `VehicleId` object with the following info:
// Model: Honda, ID: 3, License plate: 7B319X4
// Create a `Car` object `c2` using the constructor that
// accepts a `VehicleId` and pass in the `VehicleId`
// object you just made.
// Call its Print member function.
// ======================================================
std::cout << "\n";
// =================== YOUR CODE HERE ===================
// 3. Create a `Date` object with the following info:
// Day: 4, Month: 11, Year: 2018
// Create a `Car` object `c3` using the constructor that
// accepts a `Date` object and pass in the `Date` object
// you just made.
// Call its Print member function.
// ======================================================
std::cout << "\n";
// =================== YOUR CODE HERE ===================
// 4. Create a `Car` object `c4` using the constructor that
// accepts a `VehicleId` and `Date` object and pass in
// the `VehicleId` and `Date` objects you created in
// steps 2 and 3 above.
// Call its Print member function.
// ======================================================
// 5. Create an instance of `VehicleId` using the default constructor.
// 6. Create an instance of `Date` using the default constructor.
// 7. Call the `SetId` member function on `c4` and pass
// in the VehicleId you just created.
// 8. Call the `SetReleaseDate` member function on `c4`
// and pass in the VehicleId you just created.
// 9. Finally, call the print member function for `c4`.
return 0;
}
date.h file
class Date {
public:
Date() : Date(1, 1, 2022) {}
Date(int day, int month, int year) : day_(day), month_(month), year_(year) {}
int Day() const { return day_; }
void SetDay(int day) { day_ = day; }
int Month() const { return month_; }
void SetMonth(int month) { month_ = month; }
int Year() const { return year_; }
void SeyYear(int year) { year_ = year; }
private:
int day_;
int month_;
int year_;
};
car.cc file
#include "car.h"
#include <iostream>
// ========================= YOUR CODE HERE =========================
// This implementation file (car.cc) is where you should implement
// the member functions declared in the header (car.h), only
// if you didn't implement them inline within car.h.
//
// Remember to specify the name of the class with :: in this format:
// <return type> MyClassName::MyFunction() {
// ...
// }
// to tell the compiler that each function belongs to the Car class.
// ===================================================================
car.h file
#include "date.h"
#include "vehicleid.h"
// ======================= YOUR CODE HERE =======================
// Write the Car class here, containing two member variables:
// id_ to store the vehicle identity info and release_date_
// to store the car's release date.
//
// Then, define the following constructor overloads:
// 1. A default constructor, left empty. The id_ and release_date
// will be initialized to their default values automatically.
// 2. A non-default constructor, which accepts a VehicleId object.
// 3. A non-default constructor, which accepts a Date object.
// 4. A non-default constructor, which accepts a VehicleId and Date
// object (in that order).
//
// Finally, define the following member functions:
// 1. The accessor (getter) function for the id_.
// 2. The mutator (setter) function for the id_.
// 3. The accessor (getter) function for the release_date_.
// 4. The mutator (setter) function for the release_date_.
// 5. The Print member function.
//
// Note: mark functions that do not modify the member variables
// as const, by writing `const` after the parameter list.
// Pass objects by const reference, e.g. for the Date object
// passed in as input to SetReleaseDate.
// ===============================================================
vehicleid.h file
#include <string>
class VehicleId {
public:
VehicleId() : VehicleId("Tesla", 121, "TUFFY121L") {}
VehicleId(const std::string &model, int vin, const std::string &license_plate)
: model_(model), vin_(vin), license_plate_(license_plate) {}
int Vin() const { return vin_; }
void SetVin(int vin) { vin_ = vin; }
std::string Model() const { return model_; }
void SetModel(const std::string &model) { model_ = model; }
std::string LicensePlate() const { return license_plate_; }
void SetLicensePlate(const std::string &license_plate) {
license_plate_ = license_plate;
}
private:
// A vehicle identification number (VIN)
int vin_;
std::string model_;
std::string license_plate_;
};



Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

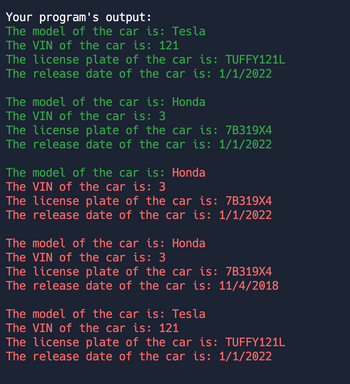
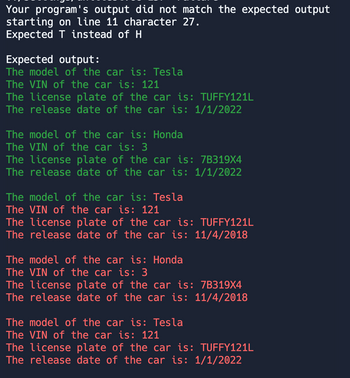
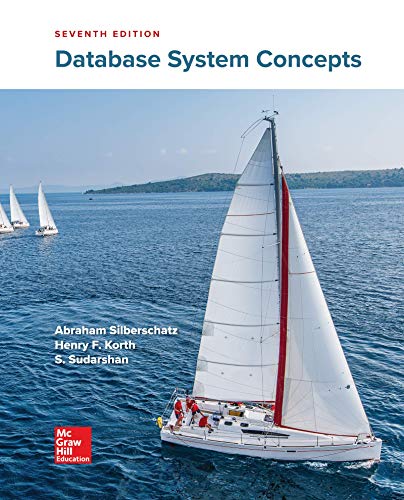
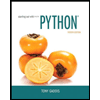
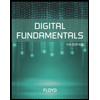
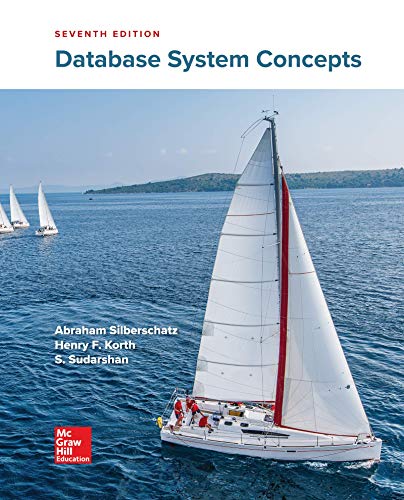
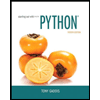
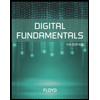
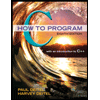
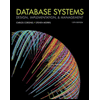
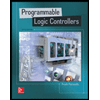