C++ program complete the code here tasks. main.cc file #include int main() { // =================== YOUR CODE HERE =================== // 1. Create a vector Pet objects called `pets`. // Don't forget to #include and "pet.h" // ====================================================== std::string name; std::string breed_name; std::string species; std::string color; double weight = 0.0; do { std::cout << "Please enter the pet's name (q to quit): "; std::getline(std::cin, name); if (name != "q") { std::cout << "Please enter the pet's species: "; std::getline(std::cin, species); std::cout << "Please enter the pet's breed: "; std::getline(std::cin, breed_name); std::cout << "Please enter the pet's color: "; std::getline(std::cin, color); std::cout << "Please enter the pet's weight (lbs): "; std::cin >> weight; std::cin.ignore(); // =================== YOUR CODE HERE =================== // 2. Create a Pet object using the input from the user // Store the newly-created Pet object into the vector. // ====================================================== } } while (name != "q"); std::cout << "Printing Pets:\n"; // =================== YOUR CODE HERE =================== // 3. Print information about each pet in the `pets` // vector by writing a loop to access each Pet object. // ====================================================== return 0; } pet.cc file #include "pet.h" #include #include // ========================= YOUR CODE HERE ========================= // This implementation file (pet.cc) is where you should implement // the member functions declared in the header (pet.h), only // if you didn't implement them inline within pet.h. // // Remember to specify the name of the class with :: in this format: // MyClassName::MyFunction() { // ... // } // to tell the compiler that each function belongs to the Pet class. // =================================================================== breed.h file #include // ======================= YOUR CODE HERE ======================= // Write the Breed class here. Refer to the README for the member // variables, constructors, and member functions needed. // // Note: you may define all functions inline in this file. // =============================================================== pet.h file #include #include "breed.h" // ======================= YOUR CODE HERE ======================= // Write the Pet class here. Refer to the README for the member // variables, constructors, and member functions needed. // // Note: mark functions that do not modify the member variables // as const, by writing `const` after the parameter list. // Pass objects by const reference when appropriate. // Remember that std::string is an object! // =============================================================== The sample output: Please enter the pet's name (q to quit): AirBud Please enter the pet's type: Dog Please enter the pet's breed: Golden Retriever Please enter the pet's color: Blonde Please enter the pet's weight (lbs): 44.5 Please enter the pet's name (q to quit): Cookie Please enter the pet's type: Dog Please enter the pet's breed: English Bulldog Please enter the pet's color: Brown & White Please enter the pet's weight (lbs): 31.2 Please enter the pet's name (q to quit): q Printing Pets: Pet 1 Name: AirBud Species: Dog Breed: Golden Retriever Color: Blonde Weight: 44.5 lbs Pet 2 Name: Cookie Species: Dog Breed: English Bulldog Color: Brown & White Weight: 31.2 lbs
C++
main.cc file
#include <iostream>
int main() {
// =================== YOUR CODE HERE ===================
// 1. Create a
// Don't forget to #include <vector> and "pet.h"
// ======================================================
std::string name;
std::string breed_name;
std::string species;
std::string color;
double weight = 0.0;
do {
std::cout << "Please enter the pet's name (q to quit): ";
std::getline(std::cin, name);
if (name != "q") {
std::cout << "Please enter the pet's species: ";
std::getline(std::cin, species);
std::cout << "Please enter the pet's breed: ";
std::getline(std::cin, breed_name);
std::cout << "Please enter the pet's color: ";
std::getline(std::cin, color);
std::cout << "Please enter the pet's weight (lbs): ";
std::cin >> weight;
std::cin.ignore();
// =================== YOUR CODE HERE ===================
// 2. Create a Pet object using the input from the user
// Store the newly-created Pet object into the vector.
// ======================================================
}
} while (name != "q");
std::cout << "Printing Pets:\n";
// =================== YOUR CODE HERE ===================
// 3. Print information about each pet in the `pets`
// vector by writing a loop to access each Pet object.
// ======================================================
return 0;
}
pet.cc file
#include "pet.h"
#include <iomanip>
#include <iostream>
// ========================= YOUR CODE HERE =========================
// This implementation file (pet.cc) is where you should implement
// the member functions declared in the header (pet.h), only
// if you didn't implement them inline within pet.h.
//
// Remember to specify the name of the class with :: in this format:
// <return type> MyClassName::MyFunction() {
// ...
// }
// to tell the compiler that each function belongs to the Pet class.
// ===================================================================
breed.h file
#include <string>
// ======================= YOUR CODE HERE =======================
// Write the Breed class here. Refer to the README for the member
// variables, constructors, and member functions needed.
//
// Note: you may define all functions inline in this file.
// ===============================================================
pet.h file
#include <string>
#include "breed.h"
// ======================= YOUR CODE HERE =======================
// Write the Pet class here. Refer to the README for the member
// variables, constructors, and member functions needed.
//
// Note: mark functions that do not modify the member variables
// as const, by writing `const` after the parameter list.
// Pass objects by const reference when appropriate.
// Remember that std::string is an object!
// ===============================================================
The sample output:
Please enter the pet's name (q to quit): AirBud
Please enter the pet's type: Dog
Please enter the pet's breed: Golden Retriever
Please enter the pet's color: Blonde
Please enter the pet's weight (lbs): 44.5
Please enter the pet's name (q to quit): Cookie
Please enter the pet's type: Dog
Please enter the pet's breed: English Bulldog
Please enter the pet's color: Brown & White
Please enter the pet's weight (lbs): 31.2
Please enter the pet's name (q to quit): q
Printing Pets:
Pet 1
Name: AirBud
Species: Dog
Breed: Golden Retriever
Color: Blonde
Weight: 44.5 lbs
Pet 2
Name: Cookie
Species: Dog
Breed: English Bulldog
Color: Brown & White
Weight: 31.2 lbs



Trending now
This is a popular solution!
Step by step
Solved in 3 steps

why is there a breed.cc file when there should be 4 files included. I would only like there to be 4 files inlcuded in order for the
solution is missing main.cc file and pet.cc file
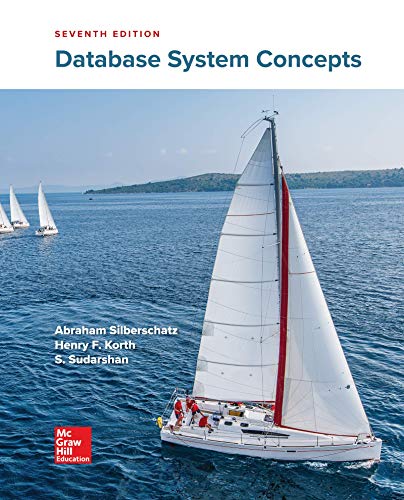
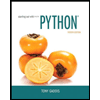
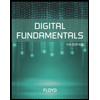
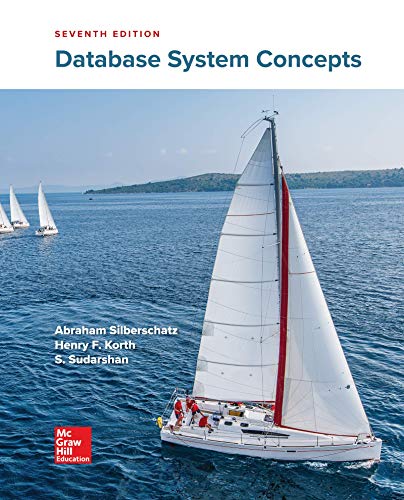
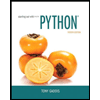
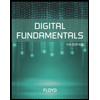
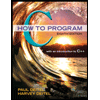
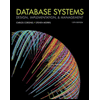
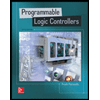