Methods (Ch6) - Review 1. (The MyRoot method) Below is a manual implementation of the Math.sqrt() method in Java. There are two methods, method #1 which calculates the square root for positive integers, and method #2, which calculates the square root of positive doubles (also works for integers). public class SquareRoot { public static void main(String[] args) { } // implement a loop of your choice here // Method that calculates the square root of integer variables public static double myRoot(int number) { double root; root=number/2; double root old; do { root old root; root (root_old+number/root_old)/2; } while (Math.abs(root_old-root)>1.8E-6); return root; } // Method that calculates the square root of double variables public static double myRoot(double number) { double root; root number/2; double root_old; do { root old root; root (root_old+number/root_old)/2; while (Math.abs (root_old-root)>1.0E-6); return root; } } Program-it-Yourself: In the main method, create a program that prints out a table of numbers that is two columns wide and 20 rows long. For each row, the left column should be a regular number, and the right column should be that number's square root. Starting from the last two digits of your Kean ID, and adding one to that number for every row, create the table using any loop of your choice. Example: Table for Kean ID XXXX20 Output: 20 4.47 21 4.58 22 4.69 40 6.32 1-1. Both methods above have the same name. Why is it important to give methods that implement the same logic the same name? (1 point) 1-2. Which loop will do the job best when you know when to start, when to finish and what to do after every repetition? Describe it and its syntax (1 point) 1-3. Implement the Program-It-Yourself problem with the loop you chose in 1-2. (1 point) 1-4. What the method(s) was/were called by your loop? Why? (1 point) 1-5. Rewrite the code you implemented for the Program-It-Yourself problem, but now, use the Math.sqrt() method instead. (1 point)
Methods (Ch6) - Review 1. (The MyRoot method) Below is a manual implementation of the Math.sqrt() method in Java. There are two methods, method #1 which calculates the square root for positive integers, and method #2, which calculates the square root of positive doubles (also works for integers). public class SquareRoot { public static void main(String[] args) { } // implement a loop of your choice here // Method that calculates the square root of integer variables public static double myRoot(int number) { double root; root=number/2; double root old; do { root old root; root (root_old+number/root_old)/2; } while (Math.abs(root_old-root)>1.8E-6); return root; } // Method that calculates the square root of double variables public static double myRoot(double number) { double root; root number/2; double root_old; do { root old root; root (root_old+number/root_old)/2; while (Math.abs (root_old-root)>1.0E-6); return root; } } Program-it-Yourself: In the main method, create a program that prints out a table of numbers that is two columns wide and 20 rows long. For each row, the left column should be a regular number, and the right column should be that number's square root. Starting from the last two digits of your Kean ID, and adding one to that number for every row, create the table using any loop of your choice. Example: Table for Kean ID XXXX20 Output: 20 4.47 21 4.58 22 4.69 40 6.32 1-1. Both methods above have the same name. Why is it important to give methods that implement the same logic the same name? (1 point) 1-2. Which loop will do the job best when you know when to start, when to finish and what to do after every repetition? Describe it and its syntax (1 point) 1-3. Implement the Program-It-Yourself problem with the loop you chose in 1-2. (1 point) 1-4. What the method(s) was/were called by your loop? Why? (1 point) 1-5. Rewrite the code you implemented for the Program-It-Yourself problem, but now, use the Math.sqrt() method instead. (1 point)
Chapter8: Arrays
Section: Chapter Questions
Problem 20RQ
Related questions
Question
![Methods (Ch6) - Review
1. (The MyRoot method) Below is a manual implementation of the Math.sqrt() method in Java.
There are two methods, method #1 which calculates the square root for positive integers, and
method #2, which calculates the square root of positive doubles (also works for integers).
public class SquareRoot {
public static void main(String[] args) {
}
// implement a loop of your choice here
// Method that calculates the square root of integer variables
public static double myRoot(int number) {
double root;
root=number/2;
double root old;
do {
root old root;
root (root_old+number/root_old)/2;
} while (Math.abs(root_old-root)>1.8E-6);
return root;
}
// Method that calculates the square root of double variables
public static double myRoot(double number) {
double root;
root number/2;
double root_old;
do {
root old root;
root (root_old+number/root_old)/2;
while (Math.abs (root_old-root)>1.0E-6);
return root;
}
}
Program-it-Yourself: In the main method, create a program that prints out a table of
numbers that is two columns wide and 20 rows long. For each row, the left column should be
a regular number, and the right column should be that number's square root. Starting from
the last two digits of your Kean ID, and adding one to that number for every row, create the
table using any loop of your choice.
Example: Table for Kean ID XXXX20
Output:
20 4.47
21 4.58
22 4.69
40 6.32](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1f1cff89-d0c3-401a-ba77-d9b5f81fcda4%2F8d3d3547-b922-4266-a0d2-81cbdddb16da%2Fhe5j2gg_processed.png&w=3840&q=75)
Transcribed Image Text:Methods (Ch6) - Review
1. (The MyRoot method) Below is a manual implementation of the Math.sqrt() method in Java.
There are two methods, method #1 which calculates the square root for positive integers, and
method #2, which calculates the square root of positive doubles (also works for integers).
public class SquareRoot {
public static void main(String[] args) {
}
// implement a loop of your choice here
// Method that calculates the square root of integer variables
public static double myRoot(int number) {
double root;
root=number/2;
double root old;
do {
root old root;
root (root_old+number/root_old)/2;
} while (Math.abs(root_old-root)>1.8E-6);
return root;
}
// Method that calculates the square root of double variables
public static double myRoot(double number) {
double root;
root number/2;
double root_old;
do {
root old root;
root (root_old+number/root_old)/2;
while (Math.abs (root_old-root)>1.0E-6);
return root;
}
}
Program-it-Yourself: In the main method, create a program that prints out a table of
numbers that is two columns wide and 20 rows long. For each row, the left column should be
a regular number, and the right column should be that number's square root. Starting from
the last two digits of your Kean ID, and adding one to that number for every row, create the
table using any loop of your choice.
Example: Table for Kean ID XXXX20
Output:
20 4.47
21 4.58
22 4.69
40 6.32

Transcribed Image Text:1-1. Both methods above have the same name. Why is it important to give methods
that implement the same logic the same name? (1 point)
1-2. Which loop will do the job best when you know when to start, when to finish and
what to do after every repetition? Describe it and its syntax (1 point)
1-3. Implement the Program-It-Yourself problem with the loop you chose in 1-2. (1 point)
1-4. What the method(s) was/were called by your loop? Why? (1 point)
1-5. Rewrite the code you implemented for the Program-It-Yourself problem, but now, use
the Math.sqrt() method instead. (1 point)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
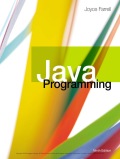
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
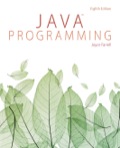
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
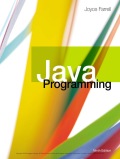
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
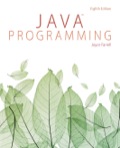
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
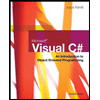
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
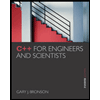
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
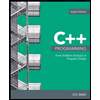
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning