Many user-created passwords are simple and easy to guess. Write a program that takes a simple password from the user input and makes it stronger by calling the new_password() function. The program should print the requested output that shows the new password returned from the function. Write a function new_password() that takes an input string, and returns a new password with the characters of the input replaced using the key below and with the "*" appended to the start and end of the password. i becomes 1 a becomes @ m becomes M B becomes 8 s becomes $ Example Input: mypassword Output: Your new password is *Myp@$$word* To verify that the unit tests will be passing, check that your function returns the correct value (i.e., a new password with the characters of the input replaced). assert new_password("mypassword") == "*Myp@$$word*"
Learning Goals
In this lab, you will:
- Write a function that matches the specifications
- Write a program that calls the required function and provides the requested output.
- Use the for OBJ in CONTAINER loop to go over characters in the string
- Use string concatenation to create a new string from provided parts
- Use if/else statement to change the concatenating objects depending on a condition
Instructions
Many user-created passwords are simple and easy to guess. Write a program that takes a simple password from the user input and makes it stronger by calling the new_password() function. The program should print the requested output that shows the new password returned from the function.
Write a function new_password() that takes an input string, and returns a new password with the characters of the input replaced using the key below and with the "*" appended to the start and end of the password.
- i becomes 1
- a becomes @
- m becomes M
- B becomes 8
- s becomes $
Example
Input:
mypassword
Output:
Your new password is *Myp@$$word*
To verify that the unit tests will be passing, check that your function returns the correct value (i.e., a new password with the characters of the input replaced).
assert new_password("mypassword") == "*Myp@$$word*"
Hints
- Review Figure 6.5.2 to see how to iterate over characters
- Use the PythonTutor to visualize what your code is doing
- Python strings are immutable, so use the string concatenation that creates a new string. You can create a new empty string and add characters to it to build a new password.
- Make sure you are clear about what the program does and what the function is supposed to do.
- Remember to properly define your function - the unit tests are checking the correctness of its return value.
Please use PYTHON Like this:
def ...
if __name__ == "__main__":
word = input()
''' Type your code here. '''


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

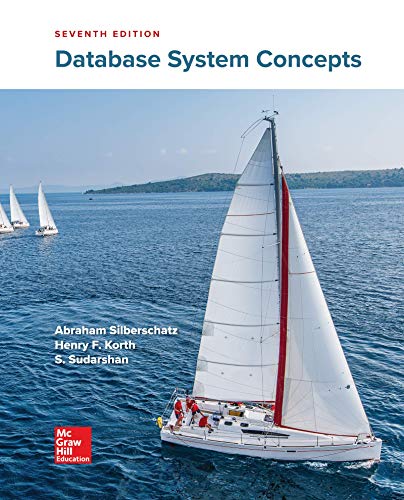
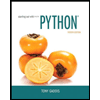
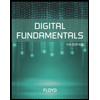
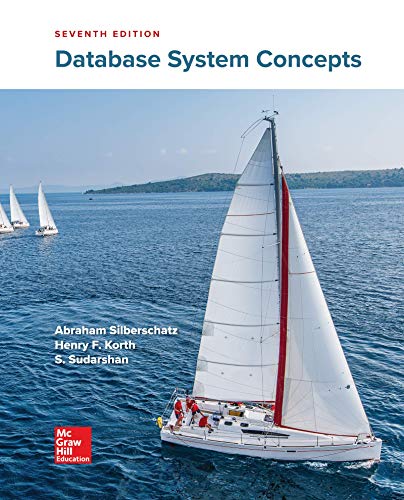
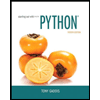
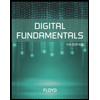
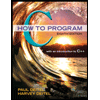
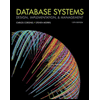
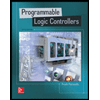