Make an animated program in where a circle moves across the screen. The circle starts out on (0,0) and moves right one square at a time. Upon reaching the end of a row, the circle will move to the first square of the next row. When the circle reaches the end of the last row, it is transferred back to the initial (0,0) position. When the user presses space, the circle should change into an apple. When the user presses space again it should change back. Use the Snakeuserinterface. Call ui.place(x,y,ui.SNAKE) to place a circle at position (x,y). Use ui.FOOD instead of ui.SNAKE to draw an apple. Please complete this in Python 3. We have to use Snakeuserinterface. This is the format we should use: import snakelib width = 0 # initialized in play_animation height = 0 # initialized in play_snake ui = None # initialized in play_animation SPEED = 20 keep_running = True def draw(): ui.clear() # fill in draw code here ui.show() def play_animation(init_ui): global width, height, ui, keep_running ui = init_ui width, height = ui.board_size() draw() while keep_running: event = ui.get_event() if event.name == "alarm": draw() # make sure you handle the quit event like below, # or the test might get stuck in an infinite loop if event.name == "quit": keep_running = False if __name__ == "__main__": # do this if running this module directly # (not when importing it for the tests) ui = snakelib.SnakeUserInterface(10, 10) ui.set_animation_speed(SPEED) play_animation(ui)
Make an animated program in where a circle moves across the screen. The circle starts out on (0,0) and moves right one square at a time. Upon reaching the end of a row, the circle will move to the first square of the next row. When the circle reaches the end of the last row, it is transferred back to the initial (0,0) position. When the user presses space, the circle should change into an apple. When the user presses space again it should change back. Use the Snakeuserinterface. Call ui.place(x,y,ui.SNAKE) to place a circle at position (x,y). Use ui.FOOD instead of ui.SNAKE to draw an apple.
Please complete this in Python 3. We have to use Snakeuserinterface.
This is the format we should use:
import snakelib width = 0 # initialized in play_animation height = 0 # initialized in play_snake ui = None # initialized in play_animation SPEED = 20 keep_running = True def draw(): ui.clear() # fill in draw code here ui.show() def play_animation(init_ui): global width, height, ui, keep_running ui = init_ui width, height = ui.board_size() draw() while keep_running: event = ui.get_event() if event.name == "alarm": draw() # make sure you handle the quit event like below, # or the test might get stuck in an infinite loop if event.name == "quit": keep_running = False if __name__ == "__main__": # do this if running this module directly # (not when importing it for the tests) ui = snakelib.SnakeUserInterface(10, 10) ui.set_animation_speed(SPEED) play_animation(ui)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

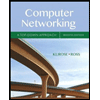
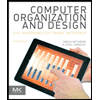
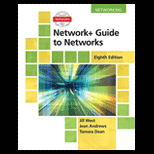
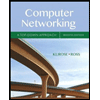
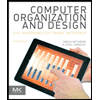
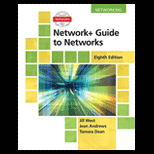
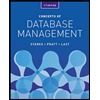
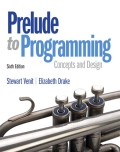
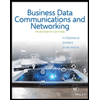