Make a flowchart based from these code /* The program is all about string manipulation wherein the user will enter a string. The program will format the string to upper case -> lower case letters alternately, disregarding the spaces. For example, the user input is masbate. The output must be MaSbAtE. */ #include #include #include #include using namespace std; void manipulateString(string myInput) { ofstream outFile; int mySpaces[5]; int myCount = 0; // make string in lowercase for (int i = 0; i < myInput.length(); i++) { myInput[i] = tolower(myInput[i]); } // count the number of spaces in the string and get the index of each of those spaces for (int i = 0; i < myInput.length(); i++) { if (myInput[i] == ' ') { mySpaces[myCount] = i; myCount++; } } // erase the spaces in the string for (int i = 0; i < myInput.length(); i++) { if (myInput[i] == ' ') myInput.erase(i, 1); } // formatting the string to upper case -> lower case letters alternately for (int i = 0; i < myInput.length(); i++) { if (i % 2 == 0) myInput[i] = toupper(myInput[i]); } // insert the spaces using the indexes from the first for loop above for (int i = 0; i < myCount; i++) myInput.insert(mySpaces[i], " "); cout << "New String Value: " << myInput << endl; outFile.open("string.txt", std::ios_base::app); outFile << myInput << endl; outFile.close(); } int main () { string myInput; cout << "Enter a string: "; getline(cin, myInput); manipulateString(myInput); // call manipulateString void function }
Make a flowchart based from these code
/*
The program is all about string manipulation wherein the user will enter a string. The program will format
the string to upper case -> lower case letters alternately, disregarding the spaces. For example, the user input is
masbate. The output must be MaSbAtE.
*/
#include <iostream>
#include <string>
#include <cctype>
#include <fstream>
using namespace std;
void manipulateString(string myInput) {
ofstream outFile;
int mySpaces[5];
int myCount = 0;
// make string in lowercase
for (int i = 0; i < myInput.length(); i++) {
myInput[i] = tolower(myInput[i]);
}
// count the number of spaces in the string and get the index of each of those spaces
for (int i = 0; i < myInput.length(); i++) {
if (myInput[i] == ' ') {
mySpaces[myCount] = i;
myCount++;
}
}
// erase the spaces in the string
for (int i = 0; i < myInput.length(); i++) {
if (myInput[i] == ' ')
myInput.erase(i, 1);
}
// formatting the string to upper case -> lower case letters alternately
for (int i = 0; i < myInput.length(); i++) {
if (i % 2 == 0)
myInput[i] = toupper(myInput[i]);
}
// insert the spaces using the indexes from the first for loop above
for (int i = 0; i < myCount; i++)
myInput.insert(mySpaces[i], " ");
cout << "New String Value: " << myInput << endl;
outFile.open("string.txt", std::ios_base::app);
outFile << myInput << endl;
outFile.close();
}
int main () {
string myInput;
cout << "Enter a string: ";
getline(cin, myInput);
manipulateString(myInput); // call manipulateString void function
}

Step by step
Solved in 2 steps with 2 images

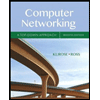
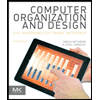
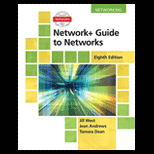
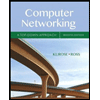
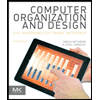
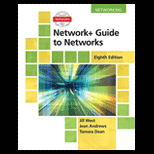
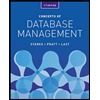
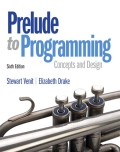
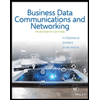