Main.java DrivingCost.java 1- import java.util.Scanner; 3 public class DrivingCost 4-{ 5 6 public static void main(String[] args) 7-{ 8 Scanner scan = new Scanner(System.in); 9 double drivingDistance; 10 double fuel Efficiency; 11 double priceperGallon; 12 double drivingcost; 13 14 System.out.print("Enter the driving distance: "); 15 driving Distance scan.nextDouble(); 16 17 System.out.print("Enter miles per gallon: "); 18 fuelEfficiency - scan.nextDouble(); 19 20 System.out.print("Enter price per gallon: "); 21 pricePerGallon - scan.nextDouble(); 22 23 drivingcost-drivingDistance / 25.5 pricePerGallon; 24 drivingcost-drivingcost 100; Ln: 1, Cot: 12 Run Share Command Line Arguments Enter the driving distance: 900.5 Enter miles per gallon: 25.5 Enter price per gallon: 3.55 The cost of driving is $125.36
Main.java DrivingCost.java 1- import java.util.Scanner; 3 public class DrivingCost 4-{ 5 6 public static void main(String[] args) 7-{ 8 Scanner scan = new Scanner(System.in); 9 double drivingDistance; 10 double fuel Efficiency; 11 double priceperGallon; 12 double drivingcost; 13 14 System.out.print("Enter the driving distance: "); 15 driving Distance scan.nextDouble(); 16 17 System.out.print("Enter miles per gallon: "); 18 fuelEfficiency - scan.nextDouble(); 19 20 System.out.print("Enter price per gallon: "); 21 pricePerGallon - scan.nextDouble(); 22 23 drivingcost-drivingDistance / 25.5 pricePerGallon; 24 drivingcost-drivingcost 100; Ln: 1, Cot: 12 Run Share Command Line Arguments Enter the driving distance: 900.5 Enter miles per gallon: 25.5 Enter price per gallon: 3.55 The cost of driving is $125.36
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
i need help with a flow chart for this program
![## Java Program to Calculate Driving Cost
This example demonstrates a simple Java program that calculates the cost of driving based on user inputs. The provided code asks the user to input the driving distance, fuel efficiency, and the price per gallon of fuel. Then, it calculates and outputs the cost of the trip.
### Code Explanation
Here is the breakdown of the Java program:
1. **Import the Scanner Class**:
```java
import java.util.Scanner;
```
2. **Define the Class DrivingCost**:
```java
public class DrivingCost
{
```
3. **Main Method**:
```java
public static void main(String[] args)
{
```
4. **Variable Declaration and User Input**:
- **Initialize Scanner**: This object will be used to capture user inputs.
```java
Scanner scan = new Scanner(System.in);
```
- **Driving Distance**: Prompts the user to input the driving distance and stores the value.
```java
System.out.print("Enter the driving distance: ");
double drivingDistance = scan.nextDouble();
```
- **Fuel Efficiency**: Prompts the user to input the fuel efficiency in miles per gallon and stores the value.
```java
System.out.print("Enter miles per gallon: ");
double fuelEfficiency = scan.nextDouble();
```
- **Price Per Gallon**: Prompts the user to input the price per gallon of fuel and stores the value.
```java
System.out.print("Enter price per gallon: ");
double pricePerGallon = scan.nextDouble();
```
5. **Calculation**:
- Calculates the driving cost using the formula:
\[
\text{drivingcost} = \left( \frac{\text{drivingDistance}}{\text{fuelEfficiency}} \times \text{pricePerGallon} \right)
\]
```java
double drivingcost = (drivingDistance / fuelEfficiency) * pricePerGallon;
```
6. **Output the Result**:
- Outputs the calculated driving cost.
```java
System.out.printf("The cost of driving is $%.2f\n", drivingcost);
```
7. **Close the Class and Main Method**:
```java
}
}
```
### Full Program Code
```java](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc4b4111f-0cb3-46a3-bff1-74a667fd5dd6%2F7ed95523-237d-43b8-9ed2-664f61316737%2Fzwhrrbq_processed.png&w=3840&q=75)
Transcribed Image Text:## Java Program to Calculate Driving Cost
This example demonstrates a simple Java program that calculates the cost of driving based on user inputs. The provided code asks the user to input the driving distance, fuel efficiency, and the price per gallon of fuel. Then, it calculates and outputs the cost of the trip.
### Code Explanation
Here is the breakdown of the Java program:
1. **Import the Scanner Class**:
```java
import java.util.Scanner;
```
2. **Define the Class DrivingCost**:
```java
public class DrivingCost
{
```
3. **Main Method**:
```java
public static void main(String[] args)
{
```
4. **Variable Declaration and User Input**:
- **Initialize Scanner**: This object will be used to capture user inputs.
```java
Scanner scan = new Scanner(System.in);
```
- **Driving Distance**: Prompts the user to input the driving distance and stores the value.
```java
System.out.print("Enter the driving distance: ");
double drivingDistance = scan.nextDouble();
```
- **Fuel Efficiency**: Prompts the user to input the fuel efficiency in miles per gallon and stores the value.
```java
System.out.print("Enter miles per gallon: ");
double fuelEfficiency = scan.nextDouble();
```
- **Price Per Gallon**: Prompts the user to input the price per gallon of fuel and stores the value.
```java
System.out.print("Enter price per gallon: ");
double pricePerGallon = scan.nextDouble();
```
5. **Calculation**:
- Calculates the driving cost using the formula:
\[
\text{drivingcost} = \left( \frac{\text{drivingDistance}}{\text{fuelEfficiency}} \times \text{pricePerGallon} \right)
\]
```java
double drivingcost = (drivingDistance / fuelEfficiency) * pricePerGallon;
```
6. **Output the Result**:
- Outputs the calculated driving cost.
```java
System.out.printf("The cost of driving is $%.2f\n", drivingcost);
```
7. **Close the Class and Main Method**:
```java
}
}
```
### Full Program Code
```java
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
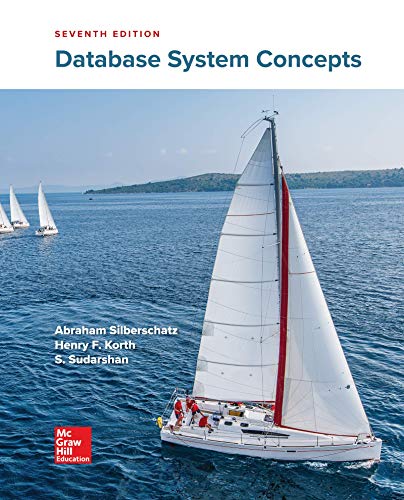
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
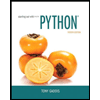
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
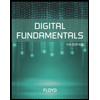
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
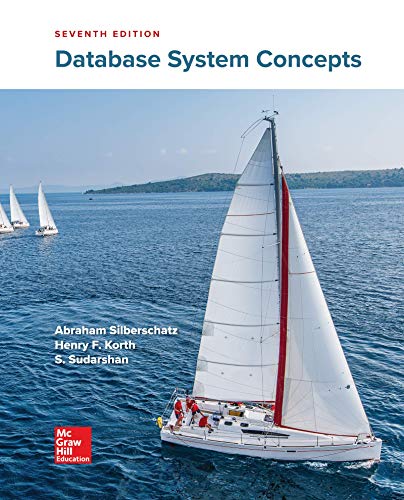
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
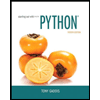
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
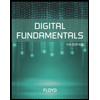
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
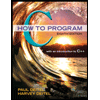
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
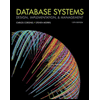
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
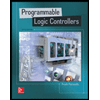
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education