Main method for reading in two square matrices, multiplying them and printing * the result.
C
parallelise it using Pthreads. Your new program should be able to use any number of threads (input as a command line argument ./p_mat_mul when running your program) and provide exactly the same output.
#include <stdio.h>
#include <stdlib.h>
/*
* Main method for reading in two square matrices, multiplying them and printing
* the result.
*
* WARNING: There are several bugs in this code - your task is to find and fix
* them!
*/
int main(int argc, char **argv) {
int N;
printf("Enter matrix dimension: ");
scanf("%d", &N);
int *A = malloc(N * N);
int *B = malloc(N * N);
int *C = malloc(N * N);
printf("Enter elements of the first matrix\n");
for (int i = 0; i < N; i++) {
for (int j = 0; j < N; j++) {
scanf("%d", &A[i * N + j]);
}
}
printf("Enter elements of the second matrix\n");
for (int i = 0; i <N; i++) {
for (int j = 0; j < N; j++) {
scanf("%d", &B[i * N + j]);
}
}
printf("Multiplying matrices...\n");
for (int i = 0; i < N; i++) {
for (int j = 0; j < N; j++) {
C[i* N + j] = 0;
for (int k = 0; k < N; k++) {
C[i * N + j] += A[i * N + k] * B[k * N + j];
}
}
}
printf("Final matrix:\n");
for (int i = 0; i < N; i++) {
for (int j = 0; j < N; j++) {
printf("%d\t", C[i + j]);
}
printf("\n");
}
free(A);
free(B);
free(C);
return 0;
}

Step by step
Solved in 2 steps with 2 images

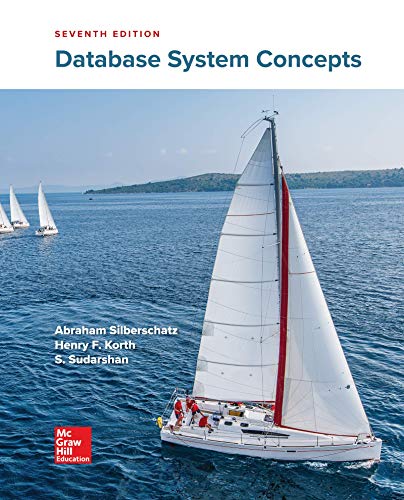
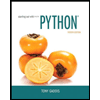
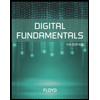
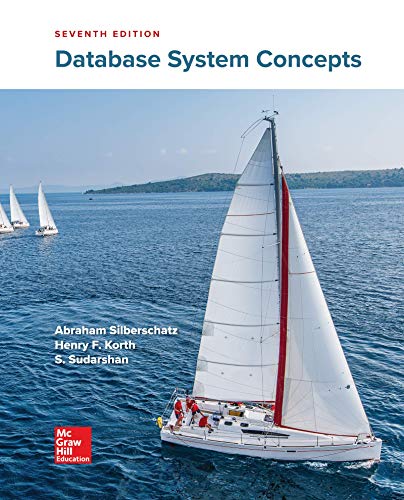
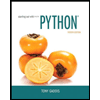
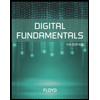
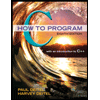
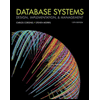
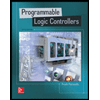