Lab 6.3 Download SavingAccount.py (See below). Overload the following operator:’ ==: return True when all attributes of two objects are equal > : return True if the balance of the account on the left is great than the balance of the account on the right Write a script that create 2 accounts. Then test the == and > operator. """ File: bank.py This module defines the SavingsAccount. """ class SavingsAccount(object): """This class represents a savings account with the owner's name, PIN, and balance.""" RATE = 0.02 def __init__(self, name, pin, balance = 0.0): self._name = name self._pin = pin self._balance = balance def __str__(self): result = 'Name: ' + self._name + '\n' result += 'PIN: ' + self._pin + '\n' result += 'Balance: ' + str(self._balance) return result def getBalance(self): return self._balance def getName(self): return self._name def getPin(self): return self._pin def deposit(self, amount): """Deposits the given amount.""" self._balance += amount return self._balance def withdraw(self, amount): """Withdraws the given amount. Returns None if successful, or an error message if unsuccessful.""" if amount < 0: return 'Amount must be >= 0' elif self._balance < amount: return 'Insufficient funds' else: self._balance -= amount return None def computeInterest(self): """Computes, deposits, and returns the interest.""" interest = self._balance * SavingsAccount.RATE self.deposit(interest) return interest
Lab 6.3 Download SavingAccount.py (See below). Overload the following operator:’
==: return True when all attributes of two objects are equal
> : return True if the balance of the account on the left is great than the balance
of the account on the right
Write a script that create 2 accounts. Then test the == and > operator.
"""
File: bank.py
This module defines the SavingsAccount.
"""
class SavingsAccount(object):
"""This class represents a savings account
with the owner's name, PIN, and balance."""
RATE = 0.02
def __init__(self, name, pin, balance = 0.0):
self._name = name
self._pin = pin
self._balance = balance
def __str__(self):
result = 'Name: ' + self._name + '\n'
result += 'PIN: ' + self._pin + '\n'
result += 'Balance: ' + str(self._balance)
return result
def getBalance(self):
return self._balance
def getName(self):
return self._name
def getPin(self):
return self._pin
def deposit(self, amount):
"""Deposits the given amount."""
self._balance += amount
return self._balance
def withdraw(self, amount):
"""Withdraws the given amount.
Returns None if successful, or an
error message if unsuccessful."""
if amount < 0:
return 'Amount must be >= 0'
elif self._balance < amount:
return 'Insufficient funds'
else:
self._balance -= amount
return None
def computeInterest(self):
"""Computes, deposits, and returns the interest."""
interest = self._balance * SavingsAccount.RATE
self.deposit(interest)
return interest

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

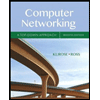
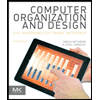
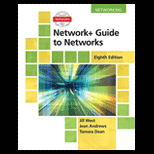
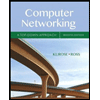
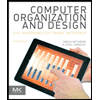
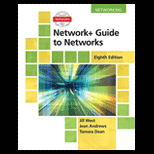
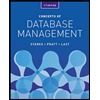
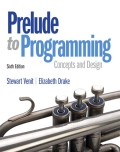
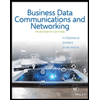