JAVA: There are three errors with the program below (issues which would prevent the program from compiling). State what the errors are and why they occur. import java.util.Scanner; import java.io.FileOutputStream; import java.io.FileInputStream; import java.io.IOException; public class MyClass extends AnotherClass, ThirdClass { private int i; private String s; public MyClass(String s) { this.s = s; i = s.length(); } public abstract int doSomething(); public void readString() throws IOException { FileInputStream inFile = new FileInputStream("Text.txt"); Scanner scan = new Scanner(inFile); s = scan.next(); i = s.length(); inFile.close(); scan.close(); } public void writeString() throws IOException { FileOutputStream outFile = new FileOutputStream("Text.txt"); PrintWriter pw = new PrintWriter(outFile); pw.println(s); outFile.close(); } }
JAVA:
There are three errors with the program below (issues which would prevent the program from compiling). State what the errors are and why they occur.
import java.util.Scanner;
import java.io.FileOutputStream;
import java.io.FileInputStream;
import java.io.IOException;
public class MyClass extends AnotherClass, ThirdClass {
private int i;
private String s;
public MyClass(String s) {
this.s = s;
i = s.length();
}
public abstract int doSomething();
public void readString() throws IOException {
FileInputStream inFile = new FileInputStream("Text.txt");
Scanner scan = new Scanner(inFile);
s = scan.next();
i = s.length();
inFile.close();
scan.close();
}
public void writeString() throws IOException {
FileOutputStream outFile = new FileOutputStream("Text.txt");
PrintWriter pw = new PrintWriter(outFile);
pw.println(s);
outFile.close();
}
}


Step by step
Solved in 3 steps

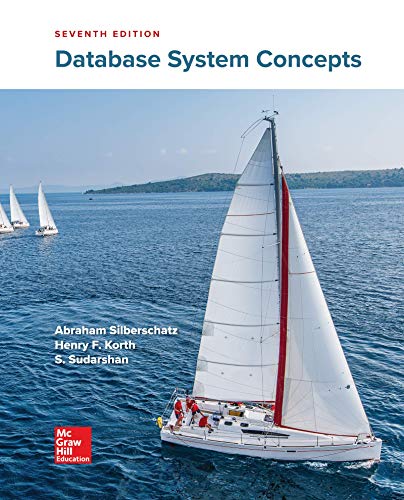
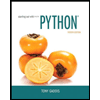
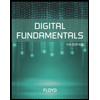
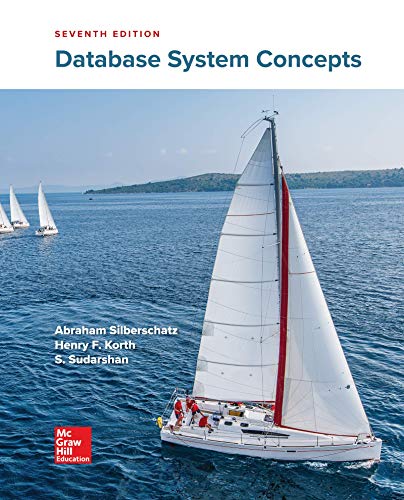
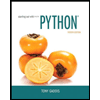
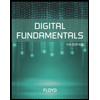
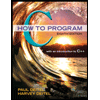
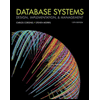
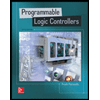