Java Programming: Below is the shank.java file which is the main method file along with shank.txt. The main goal is to make sure to use this method: (List lines = Files.readAllLines(Paths.get(filename));) to read the text file. Show the full code with the text file being read in the output. There must be no error at all. package mypack; import java.io.IOException; import java.nio.charset.Charset; import java.nio.charset.StandardCharsets; import java.nio.file.Files; import java.nio.file.Path; import java.nio.file.Paths; import java.util.List; public class Shank { public static void main(String[] args) { Path filePath = Paths.get("shank.txt"); Charset charset = StandardCharsets.UTF_8; if (args.length != 1) { System.out.println("Error: Exactly one argument is required."); System.exit(0); } String filename = args[0]; try { List lines = Files.readAllLines(Paths.get(filename)); for (String line : lines) { try { Lexer lexer = new Lexer(line); List tokens = lexer.lex(line) for (Token token : tokens) { System.out.println(token); } } catch (Exception e) { System.out.println("Exception: " + e.getMessage()); } } } catch (IOException e) { System.out.println("Error: Could not read file '" + filename + "'."); } } } Shank.txt Fibonoacci (Iterative) define add (num1,num2:integer var sum : integer) variable counter : integer Finonacci(N) int N = 10; while counter < N define start () variables num1,num2,num3 : integer add num1,num2,var num3 {num1 and num2 are added together to get num3} num1 = num2; num2 = num3; counter = counter + 1; GCD (Recursive) define add (int a,int b : gcd) if b = 0 sum = a sum gcd(b, a % b) GCD (Iterative) define add (inta, intb : gcd) if a = 0 sum = b if b = 0 sum = a while counter a != b if a > b a = a - b; else b = b - a; sum = a; variables a,b : integer a = 60 b = 96 subtract a,b
Java
package mypack;
import java.io.IOException;
import java.nio.charset.Charset;
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.List;
public class Shank {
public static void main(String[] args) {
Path filePath = Paths.get("shank.txt");
Charset charset = StandardCharsets.UTF_8;
if (args.length != 1) {
System.out.println("Error: Exactly one argument is required.");
System.exit(0);
}
String filename = args[0];
try {
List<String> lines = Files.readAllLines(Paths.get(filename));
for (String line : lines) {
try {
Lexer lexer = new Lexer(line);
List<Token> tokens = lexer.lex(line)
for (Token token : tokens) {
System.out.println(token);
}
} catch (Exception e) {
System.out.println("Exception: " + e.getMessage());
}
}
} catch (IOException e) {
System.out.println("Error: Could not read file '" + filename + "'.");
}
}
}
Shank.txt
Fibonoacci (Iterative)
define add (num1,num2:integer var sum : integer)
variable counter : integer
Finonacci(N)
int N = 10;
while counter < N
define start ()
variables num1,num2,num3 : integer
add num1,num2,var num3
{num1 and num2 are added together to get num3}
num1 = num2;
num2 = num3;
counter = counter + 1;
GCD (Recursive)
define add (int a,int b : gcd)
if b = 0
sum = a
sum gcd(b, a % b)
GCD (Iterative)
define add (inta, intb : gcd)
if a = 0
sum = b
if b = 0
sum = a
while counter a != b
if a > b
a = a - b;
else
b = b - a;
sum = a;
variables a,b : integer
a = 60
b = 96
subtract a,b

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

How do you pass the file name as a command-line argument? Show that in the code with the screenshot of the output of the text file being read in the terminal.
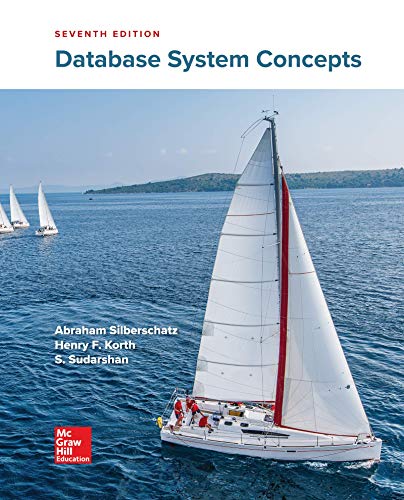
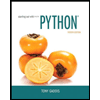
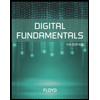
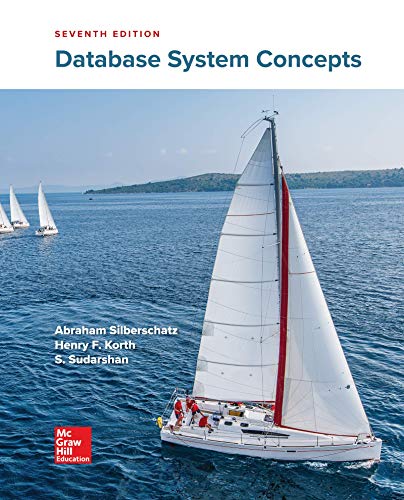
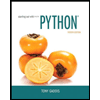
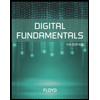
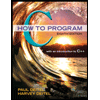
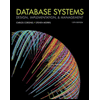
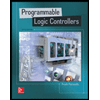