1. Complete the class code below and include a method named "search" that takes two String.parameters, a string to search and the other a target string to search for in the first string. The search method will return an integer representing the number of times the search string was found. The string being searched is a super-string formed by joining all lines of a text file, so we might expect to get multiple hits with our method. HINT: walk the String in a loop and use .substring(start, end) to examine a target-sized segment of the input string for equality to your target word. public class WordSearch { public static void main(String[] args) { String fileName = "story.txt"; String target = "help"; Scanner fileln = new Scanner(new File(fileName)); String story = ""; while(fileln.hasNextLine()) { story += fileln.nextLine(); // Build a super-String } System.out.printIn("(" + target + ") found " + search(story, target) +" times"); } // method code here } I/ end of WordSearch class
1. Complete the class code below and include a method named "search" that takes two String.parameters, a string to search and the other a target string to search for in the first string. The search method will return an integer representing the number of times the search string was found. The string being searched is a super-string formed by joining all lines of a text file, so we might expect to get multiple hits with our method. HINT: walk the String in a loop and use .substring(start, end) to examine a target-sized segment of the input string for equality to your target word. public class WordSearch { public static void main(String[] args) { String fileName = "story.txt"; String target = "help"; Scanner fileln = new Scanner(new File(fileName)); String story = ""; while(fileln.hasNextLine()) { story += fileln.nextLine(); // Build a super-String } System.out.printIn("(" + target + ") found " + search(story, target) +" times"); } // method code here } I/ end of WordSearch class
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![### Java Word Search Exercise
1. **Task Description:**
- Complete the class code below and include a **method named "search"** that takes two String parameters: a string to search through and a target string to search for in the first string.
- The search method will return an integer representing the number of times the search string was found.
- The string being searched is a super-string formed by joining all lines of a text file, so multiple occurrences of the target string are expected.
- **Hint:** Walk the String in a loop and use `<string>.substring(start, end)` to examine a segment of the input string that is sized to match the target word.
2. **Code Skeleton:**
```java
public class WordSearch {
public static void main(String[] args) {
String fileName = "story.txt";
String target = "help";
Scanner fileIn = new Scanner(new File(fileName));
String story = "";
while(fileIn.hasNextLine()) {
story += fileIn.nextLine(); // Build a super-String
}
System.out.println("(" + target + ") found " + search(story, target) + " times");
}
// method code here
} // end of WordSearch class
```
3. **Explanation:**
- **Purpose:** This exercise involves completing a Java program that reads from a text file, concatenates its contents into a single large string (super-string), and then searches for occurrences of a target substring within this super-string.
- **Key Components:**
- **Scanner:** Used for reading the text file line-by-line.
- **String Concatenation:** Each line from the file is appended to a `String` to form the super-string.
- **Search Method:** Needs to be implemented to count how many times the `target` string occurs within the `story`.
4. **Expected Outcome:**
- The program will output how many times the string "help" appears in the concatenated text from "story.txt".](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F9a95d37a-6d2c-4845-99e3-2b94485914c1%2Fd9e8d279-c8cc-4bab-ac41-6b68971b6a51%2F7j58sa8j_processed.png&w=3840&q=75)
Transcribed Image Text:### Java Word Search Exercise
1. **Task Description:**
- Complete the class code below and include a **method named "search"** that takes two String parameters: a string to search through and a target string to search for in the first string.
- The search method will return an integer representing the number of times the search string was found.
- The string being searched is a super-string formed by joining all lines of a text file, so multiple occurrences of the target string are expected.
- **Hint:** Walk the String in a loop and use `<string>.substring(start, end)` to examine a segment of the input string that is sized to match the target word.
2. **Code Skeleton:**
```java
public class WordSearch {
public static void main(String[] args) {
String fileName = "story.txt";
String target = "help";
Scanner fileIn = new Scanner(new File(fileName));
String story = "";
while(fileIn.hasNextLine()) {
story += fileIn.nextLine(); // Build a super-String
}
System.out.println("(" + target + ") found " + search(story, target) + " times");
}
// method code here
} // end of WordSearch class
```
3. **Explanation:**
- **Purpose:** This exercise involves completing a Java program that reads from a text file, concatenates its contents into a single large string (super-string), and then searches for occurrences of a target substring within this super-string.
- **Key Components:**
- **Scanner:** Used for reading the text file line-by-line.
- **String Concatenation:** Each line from the file is appended to a `String` to form the super-string.
- **Search Method:** Needs to be implemented to count how many times the `target` string occurs within the `story`.
4. **Expected Outcome:**
- The program will output how many times the string "help" appears in the concatenated text from "story.txt".
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
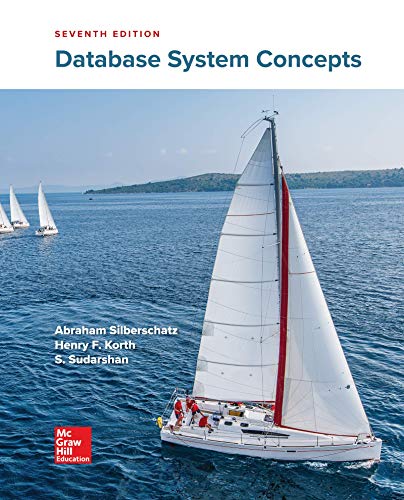
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
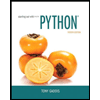
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
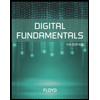
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
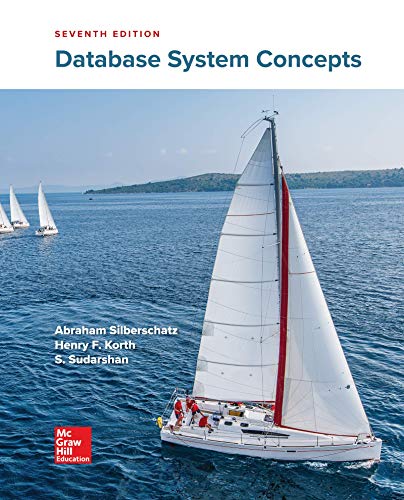
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
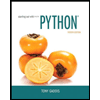
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
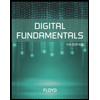
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
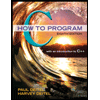
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
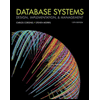
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
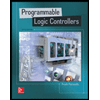
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education