JAVA PROGRAM
import java.io.*;
import java.util.*;
public class ArrayListOperations {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
while(true) {
System.out.print("Please enter the file name or type QUIT to exit:\n");
String filename = scanner.nextLine();
if(filename.equalsIgnoreCase("QUIT")) {
break;
}
File file = new File(filename);
if(!file.exists()) {
System.out.println("File: " + filename + " does not exist.");
continue;
}
ArrayList<ArrayList<Double>> data = loadFromFile(filename);
for(int i = 0; i < data.size(); i++) {
System.out.printf("Row %d Length: %d, Subtotal: %.3f\n", i, data.get(i).size(), getRowSubtotal(data, i));
}
for(int j = 0; j < getMaxColumnLength(data); j++) {
System.out.printf("Column %d Height: %d, Subtotal: %.3f\n", j, getColumnHeight(data, j), getColSubtotal(data, j));
}
System.out.printf("Array Elements: %d, Total: %.3f\n", getTotalElements(data), getTotal(data));
break;
}
}
public static ArrayList<ArrayList<Double>> loadFromFile(String filename) {
ArrayList<ArrayList<Double>> data = new ArrayList<>();
try {
Scanner fileScanner = new Scanner(new File(filename));
while(fileScanner.hasNextLine()) {
String[] parts = fileScanner.nextLine().split(" ");
ArrayList<Double> row = new ArrayList<>();
for(String part : parts) {
row.add(Double.parseDouble(part));
}
data.add(row);
}
} catch(Exception e) {
e.printStackTrace();
}
return data;
}
public static double getRowSubtotal(ArrayList<ArrayList<Double>> data, int rowIndex) {
double sum = 0.0;
for(double d : data.get(rowIndex)) {
sum += d;
}
return sum;
}
public static double getColSubtotal(ArrayList<ArrayList<Double>> data, int colIndex) {
double sum = 0.0;
for(ArrayList<Double> row : data) {
if(row.size() > colIndex) {
sum += row.get(colIndex);
}
}
return sum;
}
public static double getTotal(ArrayList<ArrayList<Double>> data) {
double sum = 0.0;
for(ArrayList<Double> row : data) {
for(double d : row) {
sum += d;
}
}
return sum;
}
public static int getMaxColumnLength(ArrayList<ArrayList<Double>> data) {
int max = 0;
for(ArrayList<Double> row : data) {
if(row.size() > max) {
max = row.size();
}
}
return max;
}
public static int getColumnHeight(ArrayList<ArrayList<Double>> data, int colIndex) {
int count = 0;
for(ArrayList<Double> row : data) {
if(row.size() > colIndex) {
count++;
}
}
return count;
}
public static int getTotalElements(ArrayList<ArrayList<Double>> data) {
int count = 0;
for(ArrayList<Double> row : data) {
count += row.size();
}
return count;
}
}
Test Case 1
quitENTER
Test Case 2
QUITENTER
Test Case 5
badfile.txtENTER
File: badfile.txt does not exist.\n
Please enter the file name again or type QUIT to exit:\n
quitENTER
Test Case 6
badfile.txtENTER
File: badfile.txt does not exist.\n
Please enter the file name again or type QUIT to exit:\n
QUITENTER
Test Case 11
badfile.txtENTER
File: badfile.txt does not exist.\n
Please enter the file name again or type QUIT to exit:\n
badfile2.txtENTER
File: badfile2.txt does not exist.\n
Please enter the file name again or type QUIT to exit:\n
input3.txtENTER
Row 0 Length: 6, Subtotal: 2025.100\n
Row 1 Length: 6, Subtotal: 3604.000\n
Row 2 Length: 6, Subtotal: 1628.660\n
Column 0 Height: 3, Subtotal: 1568.770\n
Column 1 Height: 3, Subtotal: 1801.420\n
Column 2 Height: 3, Subtotal: 258.690\n
Column 3 Height: 3, Subtotal: 1568.770\n
Column 4 Height: 3, Subtotal: 1801.420\n
Column 5 Height: 3, Subtotal: 258.690\n
Array Elements: 18, Total: 7257.760\n

![Test Case 5 Failed Show what's missing
Please enter the file name or type QUIT to exit: \n
badfile.txt ENTER
File: badfile.txt does not exist.\n
Please enter the file name or type QUIT to exit: \n
quit ENTER
Test Case 6
Failed
Please enter the file name or type QUIT to exit: \n
badfile.txt ENTER
Show what's missing
File: badfile.txt does not exist.\n
Please enter the file name or type QUIT to exit: \n
QUIT ENTER
Test Case 7 Failed Show what's missing
Please enter the file name or type QUIT to exit: \n
badfile.txt ENTER
File: badfile.txt does not exist.\n
Please enter the file name or type QUIT to exit: \n
QuIt ENTER
Test Case 10 Failed
Show what's missing D
Please enter the file name or type QUIT to exit: \n
badfile.txt ENTER
Test Case 11
File: badfile.txt does not exist.\n
Please enter the file name or type QUIT to exit: \n
input2.txt ENTER
Row 8 Length: 3, Subtotal: 1012.550 \n
Row 1 Length: 3, Subtotal: 1802.000 \n
Row 2 Length: 3, Subtotal: 814.330\n
Column
Height: 3, Subtotal: 1568.770 \n
Column 1 Height: 3, Subtotal: 1801.420 \n
Column 2 Height: 3, Subtotal: 258.690 \n
Array Elements: 9, Total: 3628.880 \n
Failed Show what's missing
Please enter the file name or type QUIT to exit: \n
badfile.txt ENTER
File: badfile.txt does not exist.\n
Please enter the file name or type QUIT to exit: \n
badfile2.txt ENTER
File: badfile2.txt does not exist. \n
Please enter the file name or type QUIT to exit: \n
input3.txt ENTER
Row 8 Length: 6, Subtotal: 2025.100 \n
Row 1 Length: 6, Subtotal: 3604.000 \n
Row 2 Length: 6, Subtotal: 1628.660\n
Column
Height: 3, Subtotal: 1568.770 \n
Column 1 Height: 3, Subtotal: 1801.420 \n
Column 2 Height: 3, Subtotal: 258.690 \n
Column 3 Height: 3, Subtotal: 1568.770 \n
Column 4 Height: 3, Subtotal: 1801.420|\n]
Column 5 Height: 3, Subtotal: 258.690 \n
Array Elements: 18, Total: 7257.760 \n
Test Case 5 Failed
Show what's missing
Please enter the file name or type QUIT to exit: \n]
badfile.txt ENTER
File: badfile.txt does not exist.\n
Please enter the file name again or type QUIT to exit: \n
quit ENTER
Test Case 6 Failed Show what's missing
Please enter the file name or type QUIT to exit: \n
badfile.txt ENTER
File: badfile.txt does not exist.\n
Please enter the file name again or type QUIT to exit: \n
QUIT ENTER
Test Case 7 Failed Show what's missing
Please enter the file name or type QUIT to exit: \n
badfile.txt ENTER
File: badfile.txt does not exist.\n
Please enter the file name again or type QUIT to exit: \n
QuIt ENTER
Test Case 10 Failed Show what's missing
Please enter the file name or type QUIT to exit: \n
badfile.txt ENTER
File: badfile.txt does not exist.\n
Please enter the file name again or type QUIT to exit: \n
input2.txt ENTER
RowLength: 3, Subtotal: 1012.550\n
Row 1 Length: 3, Subtotal: 1802.000 \n
Row 2 Length: 3, Subtotal: 814.330 \n
Column Height: 3, Subtotal: 1568.770 \n
Column 1 Height: 3, Subtotal: 1801.420 \n
Column 2 Height: 3, Subtotal: 258.690\n
Array Elements: 9, Total: 3628.880 \n
Test Case 11 Failed Show what's missing
Please enter the file name or type QUIT to exit: \n
badfile.txt ENTER
File: badfile.txt does not exist.\n
Please enter the file name again or type QUIT to exit: \n
badfile2.txt ENTER
File: badfile2.txt does not exist.\n
Please enter the file name again or type QUIT to exit: \n
input3.txt ENTER
Row 0 Length: 6, Subtotal: 2025.100 \n
Row 1 Length: 6, Subtotal: 3604.000 \n
Row 2 Length: 6, Subtotal: 1628.660 \n
Column Height: 3, Subtotal: 1568.770 \n
Column 1 Height: 3, Subtotal: 1801.420 \n
Column 2 Height: 3, Subtotal: 258.690\n
Column 3 Height: 3, Subtotal: 1568.770 \n
Column 4 Height: 3, Subtotal: 1801.420 \n
Column 5 Height: 3, Subtotal: 258.690\n
Array Elements: 18, Total: 7257.760\n](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F936391a3-65fb-491a-ae21-62c7d9d10962%2Fd73f1c6b-7d72-4a93-ac90-7c2ab0bfa84c%2Fjahazun_processed.png&w=3840&q=75)

Step by step
Solved in 3 steps with 1 images

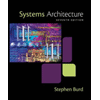
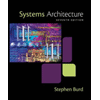