(Java) How can I implement a binary search tree in a program to store data, and use the delete method to trim the tree? The program should have a 'populate' button that obtains a string from the user, creates a sorted binary search tree of characters, and prints the tree using the DisplayTree class. It should also print the characters on one line in sorted order by traversing the tree in inorder fashion. The program should also have a 'Trim Tree' button that obtains a second line of input to delete characters from the tree, trimming the tree accordingly. It should ignore characters that are not in the tree, and only delete one character for each occurrence in the second line of input. When all characters from the second line have been deleted from the tree, the program should print the remaining characters in the tree using the DisplayTree class. The output should be labeled appropriately, and no spaces or commas should be used between tree elements in the inorder traversal. Here is the base code given to me: package problem_set_30; import static javax.swing.JOptionPane.*; public class Pro3001{ public static void main(String[] args) { new Environment101().setVisible(true); }} class Environment101 extends View { // BinaryTree101< ?? > charTree = ??; @Override public void buttonOneAction() { String lineInput = showInputDialog("Enter a line of input to insert:","marauder"); //************************************** // Create a binary search tree from // the input string. //************************************** // o // o output.setText( "Tree after input.\n" ); // output.append( new DisplayTree( charTree.getRoot()).toString() ); // o // o } @Override public void buttonTwoAction() { String lineInput = showInputDialog("Enter a line of input to remove:","rummage"); //************************************** // Trim the binary search tree using // the input string. //************************************** // o // o output.setText( "Tree after trimming.\n" ); // output.append( new DisplayTree( charTree.getRoot()).toString() ); // o // o } @Override public void setParams() { buttonOne.setText("Create Tree"); buttonTwo.setText("Trim Tree"); } } class BinaryTree101> { private TreeNode root = null; public void delete(T key) { root = delete(root, key); } private TreeNode delete(TreeNode current, T item) { if(current == null) return null; if( item.equals(current.getData()) ) { if(current.getLeft() == null && current.getRight() == null) return null; if (current.getRight() == null) return current.getLeft(); if (current.getLeft() == null) return current.getRight(); TreeNode follow = current; TreeNode travel = current.getRight(); while(travel.getLeft() != null) { follow = travel; travel = travel.getLeft(); } travel.setLeft(current.getLeft()); if(current.getRight() != travel) { follow.setLeft(travel.getRight()); travel.setRight(current.getRight()); } return travel; } if( item.compareTo(current.getData()) <= 0 ) return current.setLeft( delete(current.getLeft(),item) ); else return current.setRight( delete(current.getRight(),item) ); } public boolean searchTree(T key) { return searchTree(root, key); } private boolean searchTree(TreeNode current, T key) { if (current == null) return false; else if ( key.equals( current.getData() ) ) return true; else if ( key.compareTo(current.getData()) <= 0 ) return searchTree(current.getLeft(),key); else return searchTree(current.getRight(),key); } public void add(T data) { root = add( root, new TreeNode<>( data, null, null ) ); } private TreeNode add(TreeNode current, TreeNode temp) { if( current == null ) return temp; else if ( temp.getData().compareTo(current.getData()) <= 0 ) return current.setLeft( add(current.getLeft(), temp) ); else return current.setRight ( add(current.getRight(), temp) ); } public TreeNode getRoot() { return root; } }
(Java) How can I implement a binary search tree in a program to store data, and use the delete method to trim the tree? The program should have a 'populate' button that obtains a string from the user, creates a sorted binary search tree of characters, and prints the tree using the DisplayTree class. It should also print the characters on one line in sorted order by traversing the tree in inorder fashion. The program should also have a 'Trim Tree' button that obtains a second line of input to delete characters from the tree, trimming the tree accordingly. It should ignore characters that are not in the tree, and only delete one character for each occurrence in the second line of input. When all characters from the second line have been deleted from the tree, the program should print the remaining characters in the tree using the DisplayTree class. The output should be labeled appropriately, and no spaces or commas should be used between tree elements in the inorder traversal.
Here is the base code given to me:

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

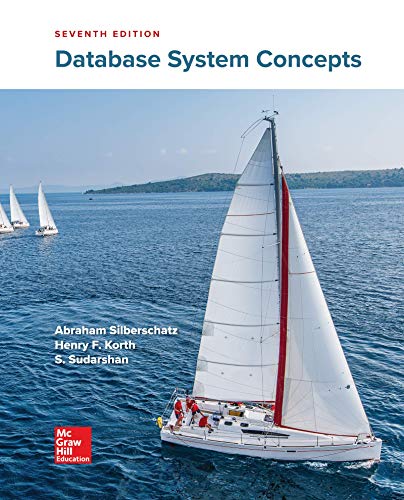
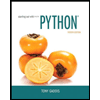
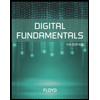
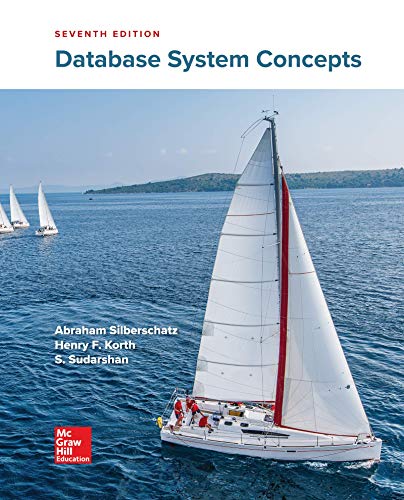
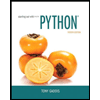
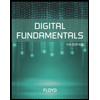
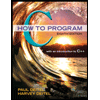
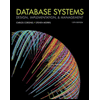
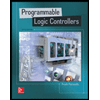