In this lab you are asked to complete the provided code so that it: Accepts integers as input from the user and appends them to a singly linked list (until the user enters -1) Then shifts all the elements in the singly-linked list to the right, so the tail becomes the head, while the previous ** head** shifts to the position of head.next Then print the modified singly-linked-list print "Empty!" if the linked list is empty
In this lab you are asked to complete the provided code so that it:
- Accepts integers as input from the user and appends them to a singly linked list (until the user enters -1)
- Then shifts all the elements in the singly-linked list to the right, so the tail becomes the head, while the previous ** head** shifts to the position of head.next
- Then print the modified singly-linked-list
- print "Empty!" if the linked list is empty
class Node:
def __init__(self, initial_data):
self.data = initial_data
self.next = None
def __str__(self):
return str(self.data)
class LinkedList:
def __init__(self):
self.head = None
self.tail = None
def append(self, new_node):
if self.head == None:
self.head = new_node
self.tail = new_node
else:
self.tail.next = new_node
self.tail = new_node
def prepend(self, new_node):
if self.head == None:
self.head = new_node
self.tail = new_node
else:
new_node.next = self.head
self.head = new_node
def remove_after(self, current_node):
# Special case, remove head
if (current_node == None) and (self.head != None):
succeeding_node = self.head.next
self.head = succeeding_node
if succeeding_node == None: # Remove last item
self.tail = None
elif current_node.next != None:
succeeding_node = current_node.next.next
current_node.next = succeeding_node
if succeeding_node == None: # Remove tail
self.tail = current_node
def printList(self):
if(self.head == None):
print('Empty!')
else:
node = self.head
while(node is not None):
print(node.data),
node = node.next
def shift(self):
#** your code goes here **
return self
if __name__ == '__main__':
#** Your code goes here **



Step by step
Solved in 2 steps

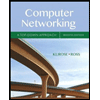
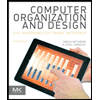
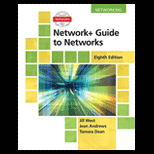
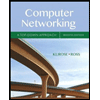
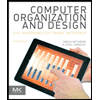
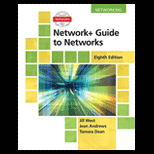
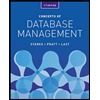
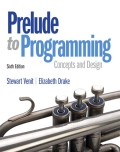
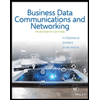