java Given Array : ARRAY[] = [11, 21, 33, 40, 50] Write a full and complete recursive implementation of the Binary Search Algorithm such that you find your target element 21 in O(log2(n)) time complexity overall and O(1) space complexity. Here, n is the length of the list of input integers (array). We will not be considering any memory used by recursion.
java
Given Array :
ARRAY[] = [11, 21, 33, 40, 50]
Write a full and complete recursive implementation of the Binary Search
Algorithm such that you find your target element 21 in O(log2(n)) time
complexity overall and O(1) space complexity. Here, n is the length of
the list of input integers (array). We will not be considering any memory
used by recursion.

Task :- Write a Java code to implement binary search.
Java code :-
import java.io.*;
class Main {
public static int binarySearch(int[] arr, int low, int high, int target) {
if(low > high)
return -1;
int mid = (low+high)/2;
if(arr[mid]==target)
return mid;
if(arr[mid] > target)
// search in left half
return binarySearch(arr,low,mid-1,target);
else
// search in right half
return binarySearch(arr,mid+1,high,target);
}
public static void main(String[] args) {
int[] arr = {11,21,33,40,50};
int index = binarySearch(arr,0,arr.length-1,21);
if(index==-1)
System.out.println("target is not found in given array.");
else
System.out.println("target is found at index " + index);
}
}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

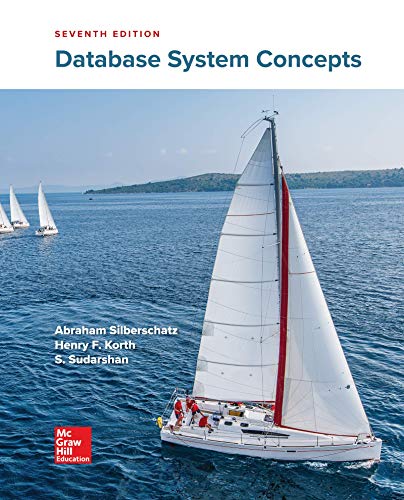
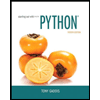
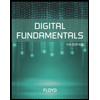
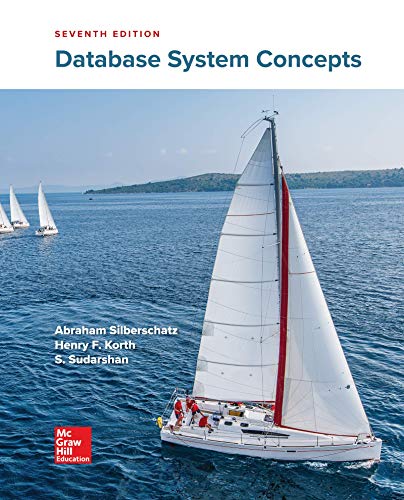
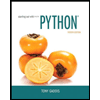
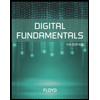
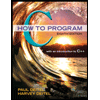
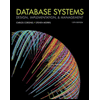
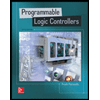