Java: Derive the class ExtClock from the class Clock by adding a data member to store the time zone. Add necessary methods and constructors to make the class functional. Also write the definitions of the methods and constructors. Here is the code: public class Clock { private int hr; private int min; private int sec; public Clock() { setTime(0, 0, 0); } public Clock(int hours, int minutes, int seconds) { setTime(hours, minutes, seconds); } public void setTime(int hours, int minutes, int seconds) { if (0 <= hours && hours < 24) hr = hours; else hr = 0; if (0 <= minutes && minutes < 60) min = minutes; else min = 0; if(0 <= seconds && seconds < 60) sec = seconds; else sec = 0; } public int getHours() { return hr; } public int getMinutes() { return min; } public int getSeconds() { return sec; } public void printTime() { if (hr < 10) System.out.print("0"); System.out.print(hr + ":"); if (min < 10) System.out.print("0"); System.out.print(min + ":"); if (sec < 10) System.out.print("0"); System.out.print(sec); } public void incrementSeconds() { sec++; if (sec > 59) { sec = 0; incrementMinutes(); } } public void incrementMinutes() { min++; if (min > 59) { min = 0; incrementHours(); } } public void incrementHours() { hr++; if(hr > 23) hr = 0; } public boolean equals(Clock otherClock) { return (hr == otherClock.hr && min == otherClock.min && sec == otherClock.sec); } public void makeCopy(Clock otherClock) { hr = otherClock.hr; min = otherClock.min; sec = otherClock.sec; } public Clock getCopy() { Clock temp = new Clock(); temp.hr = hr; temp.min = min; temp.sec = sec; return temp; } }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Java: Derive the class ExtClock from the class Clock by adding a data member to store the time zone. Add necessary methods and constructors to make the class functional. Also write the definitions of the methods and constructors.
Here is the code:
public class Clock
{
private int hr;
private int min;
private int sec;
public Clock()
{
setTime(0, 0, 0);
}
public Clock(int hours, int minutes, int seconds)
{
setTime(hours, minutes, seconds);
}
public void setTime(int hours, int minutes, int seconds)
{
if (0 <= hours && hours < 24)
hr = hours;
else
hr = 0;
if (0 <= minutes && minutes < 60)
min = minutes;
else
min = 0;
if(0 <= seconds && seconds < 60)
sec = seconds;
else
sec = 0;
}
public int getHours()
{
return hr;
}
public int getMinutes()
{
return min;
}
public int getSeconds()
{
return sec;
}
public void printTime()
{
if (hr < 10)
System.out.print("0");
System.out.print(hr + ":");
if (min < 10)
System.out.print("0");
System.out.print(min + ":");
if (sec < 10)
System.out.print("0");
System.out.print(sec);
}
public void incrementSeconds()
{
sec++;
if (sec > 59)
{
sec = 0;
incrementMinutes();
}
}
public void incrementMinutes()
{
min++;
if (min > 59)
{
min = 0;
incrementHours();
}
}
public void incrementHours()
{
hr++;
if(hr > 23)
hr = 0;
}
public boolean equals(Clock otherClock)
{
return (hr == otherClock.hr && min == otherClock.min && sec == otherClock.sec);
}
public void makeCopy(Clock otherClock)
{
hr = otherClock.hr;
min = otherClock.min;
sec = otherClock.sec;
}
public Clock getCopy()
{
Clock temp = new Clock();
temp.hr = hr;
temp.min = min;
temp.sec = sec;
return temp;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

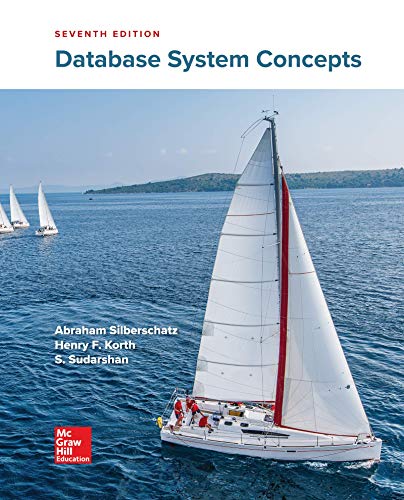
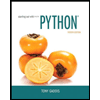
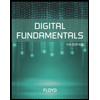
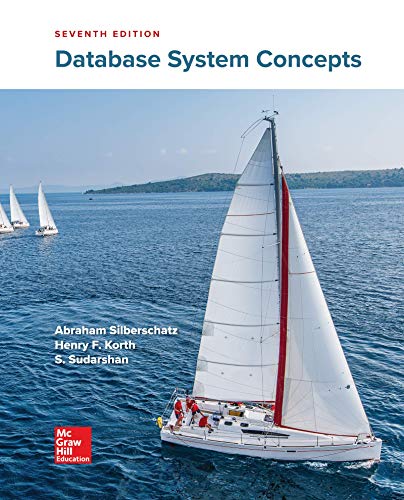
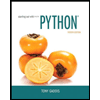
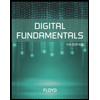
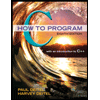
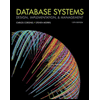
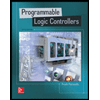