×3 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 struct node *newNode malloc(sizeof(struct node)); newNode -> target; newNode-> NULL; //if head is NULL, it is an empty list if (*headptr == NULL) *headPtr = newNode; target node => new node; node=>next = NULL; target node=>next != NULL;
×3 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 struct node *newNode malloc(sizeof(struct node)); newNode -> target; newNode-> NULL; //if head is NULL, it is an empty list if (*headptr == NULL) *headPtr = newNode; target node => new node; node=>next = NULL; target node=>next != NULL;
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![The image displays a section of error messages from a C++ compiler output. Below is the transcription of the text:
```
10 50 C:\Users\lr1821655\OneDrive\Dropbox\Screenshots\One... [Error] invalid application of 'sizeof' to incomplete type 'node'
12 9 C:\Users\lr1821655\OneDrive\Dropbox\Screenshots\One... [Error] invalid use of incomplete type 'struct node'
3 0 C:\Users\lr1821655\OneDrive\Dropbox\Screenshots\One... In file included from C:\Users\lr1821655\OneDrive\Dropbox\Screenshots\OneDrive\Documents\Assignment 5P1\llcpImp.cpp
9 11 C:\Users\lr1821655\OneDrive\Dropbox\Screenshots\One... [Error] forward declaration of 'struct node'
12 11 C:\Users\lr1821655\OneDrive\Dropbox\Screenshots\One... [Error] expected unqualified-id before '=' token
```
### Explanation of the Errors:
1. **Invalid application of 'sizeof' to incomplete type 'node':**
- This error indicates that the `sizeof` operator is being used on a type `node` which hasn't been completely defined yet. Incomplete types are declared but not fully defined; hence, their size cannot be determined.
2. **Invalid use of incomplete type 'struct node':**
- This suggests that there is an attempt to use the `struct node` in a way that requires knowledge of its full definition, but the compiler only sees a forward declaration.
3. **In file included from ... llcpImp.cpp:**
- Indicates the location of the file from which the error messages originate. Understanding this helps in tracking the source of errors in complex projects with multiple file inclusions.
4. **Forward declaration of 'struct node':**
- Implies that a `struct node` is declared but not defined completely at the point of use. A forward declaration specifies the existence of a type without giving details about its contents, typically used to handle circular dependencies.
5. **Expected unqualified-id before '=' token:**
- This error usually occurs when there is a syntax error, often caused by unexpected tokens or an improper declaration/initialization format.
These errors are typical in](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F3632b9ca-0e22-48c8-943d-c9e08fc0f04c%2Fabdebf57-ceae-4da5-a9b2-318124685a09%2Fbprjsvt_processed.png&w=3840&q=75)
Transcribed Image Text:The image displays a section of error messages from a C++ compiler output. Below is the transcription of the text:
```
10 50 C:\Users\lr1821655\OneDrive\Dropbox\Screenshots\One... [Error] invalid application of 'sizeof' to incomplete type 'node'
12 9 C:\Users\lr1821655\OneDrive\Dropbox\Screenshots\One... [Error] invalid use of incomplete type 'struct node'
3 0 C:\Users\lr1821655\OneDrive\Dropbox\Screenshots\One... In file included from C:\Users\lr1821655\OneDrive\Dropbox\Screenshots\OneDrive\Documents\Assignment 5P1\llcpImp.cpp
9 11 C:\Users\lr1821655\OneDrive\Dropbox\Screenshots\One... [Error] forward declaration of 'struct node'
12 11 C:\Users\lr1821655\OneDrive\Dropbox\Screenshots\One... [Error] expected unqualified-id before '=' token
```
### Explanation of the Errors:
1. **Invalid application of 'sizeof' to incomplete type 'node':**
- This error indicates that the `sizeof` operator is being used on a type `node` which hasn't been completely defined yet. Incomplete types are declared but not fully defined; hence, their size cannot be determined.
2. **Invalid use of incomplete type 'struct node':**
- This suggests that there is an attempt to use the `struct node` in a way that requires knowledge of its full definition, but the compiler only sees a forward declaration.
3. **In file included from ... llcpImp.cpp:**
- Indicates the location of the file from which the error messages originate. Understanding this helps in tracking the source of errors in complex projects with multiple file inclusions.
4. **Forward declaration of 'struct node':**
- Implies that a `struct node` is declared but not defined completely at the point of use. A forward declaration specifies the existence of a type without giving details about its contents, typically used to handle circular dependencies.
5. **Expected unqualified-id before '=' token:**
- This error usually occurs when there is a syntax error, often caused by unexpected tokens or an improper declaration/initialization format.
These errors are typical in

Transcribed Image Text:```c
struct node *newNode = malloc(sizeof(struct node));
newNode -> target;
newNode-> = NULL;
//if head is NULL, it is an empty list
if(*headptr == NULL)
*headPtr = newNode;
target node => new node;
node->next = NULL;
target node->next != NULL;
```
### Explanation
This code snippet demonstrates the initialization and insertion of a new node in a linked list. Here’s what each part does:
1. **Node Allocation:**
```c
struct node *newNode = malloc(sizeof(struct node));
```
- Allocates memory for a new node.
2. **Node Assignment:**
```c
newNode -> target;
newNode-> = NULL;
```
- Assigns target values to the new node and initializes it to point to NULL, indicating that it doesn’t yet link to another node.
3. **Handling Empty List:**
```c
if(*headptr == NULL)
```
- Checks if the head pointer is NULL. If it is, the list is empty.
4. **Insertion:**
```c
*headPtr = newNode;
target node => new node;
node->next = NULL;
target node->next != NULL;
```
- If the list is empty, the newly created node becomes the head.
- Adjustments to link the nodes accordingly, ensuring proper list connections.
This code is commonly used for managing dynamic data in C using linked lists, allowing for efficient data manipulation and storage.
Expert Solution

Step 1
This is C++ program dealing with linkedlist data structure implementing with struct.
A thorough in-depth knowledge of struct UDT, pointer concept and malloc () is required to understand and debugging the given code.
Step by step
Solved in 3 steps

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
It say that line 11 scope was not define
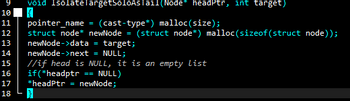
Transcribed Image Text:9
10
11
12
13
14
15
16
17
18
void IsolateTargetSoloAsTail(Node* headPtr, int target)
pointer_name
(cast-type*) malloc(size);
struct node* newNode = (struct node*) malloc(sizeof(struct node));
newNode->data
= target;
newNode->next = NULL;
//if head is NULL, it is an empty List
if (*headptr == NULL)
*headPtr = newNode;
=
![11
11
11
11
1 C:\Users\r1821655\OneDrive\Dropbox\Screenshots\One...
17 C:\Users\r1821655\OneDrive\Dropbox\Screenshots\One...
22 C:\Users\r1821655\OneDrive\Dropbox\Screenshots\One...
27 C:\Users\r1821655\OneDrive\Dropbox\Screenshots\One...
12 64 C:\Users\r1821655\OneDrive\Dropbox\Screenshots\One...
13 8 C:\Users\r1821655\OneDrive\Dropbox\Screenshots\One...
0 C:\Users\r1821655\OneDrive\Dropbox\Screenshots\...
C:\Users\r1821655\OneDrive\Dropbox\Screenshots\One...
3
11
8 C:\Users\r1821655\OneDrive\Dropbox\Screenshots\One...
14
[Error] 'pointer_name' was not declared in this scope
[Error] 'cast' was not declared in this scope
[Error] 'type' was not declared in this scope
[Error] expected primary-expression before ')' token
[Error] invalid application of 'sizeof' to incomplete type 'node'
[Error] invalid use of incomplete type 'struct node'
In file included from
[Error] forward declaration of 'struct node'
[Error] invalid use of incomplete type 'struct node'
C:\Users\r1821655\OneDrive\Dropbox\Screenshots\OneDrive\Documents\Assignment 5P1\llcplmp.cpp](https://content.bartleby.com/qna-images/question/3632b9ca-0e22-48c8-943d-c9e08fc0f04c/96652e64-e06b-43f5-a866-f05c68a13465/7nqi8og_thumbnail.png)
Transcribed Image Text:11
11
11
11
1 C:\Users\r1821655\OneDrive\Dropbox\Screenshots\One...
17 C:\Users\r1821655\OneDrive\Dropbox\Screenshots\One...
22 C:\Users\r1821655\OneDrive\Dropbox\Screenshots\One...
27 C:\Users\r1821655\OneDrive\Dropbox\Screenshots\One...
12 64 C:\Users\r1821655\OneDrive\Dropbox\Screenshots\One...
13 8 C:\Users\r1821655\OneDrive\Dropbox\Screenshots\One...
0 C:\Users\r1821655\OneDrive\Dropbox\Screenshots\...
C:\Users\r1821655\OneDrive\Dropbox\Screenshots\One...
3
11
8 C:\Users\r1821655\OneDrive\Dropbox\Screenshots\One...
14
[Error] 'pointer_name' was not declared in this scope
[Error] 'cast' was not declared in this scope
[Error] 'type' was not declared in this scope
[Error] expected primary-expression before ')' token
[Error] invalid application of 'sizeof' to incomplete type 'node'
[Error] invalid use of incomplete type 'struct node'
In file included from
[Error] forward declaration of 'struct node'
[Error] invalid use of incomplete type 'struct node'
C:\Users\r1821655\OneDrive\Dropbox\Screenshots\OneDrive\Documents\Assignment 5P1\llcplmp.cpp
Solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
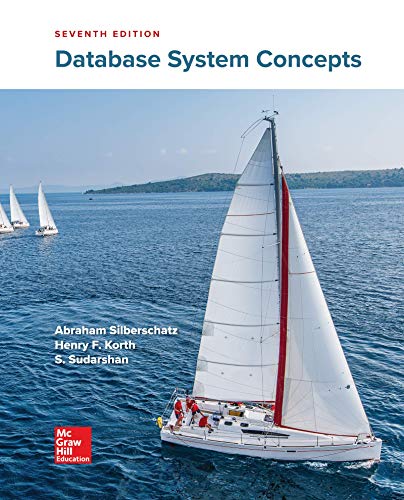
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
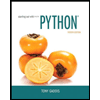
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
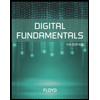
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
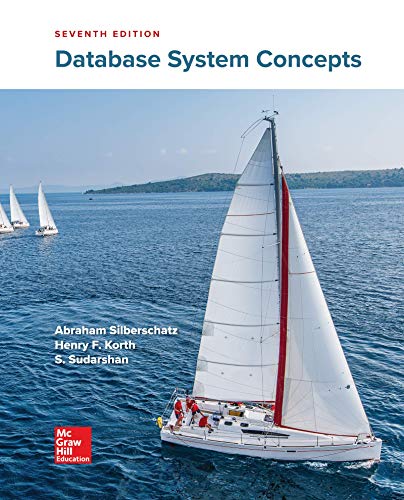
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
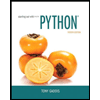
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
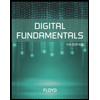
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
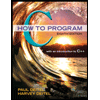
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
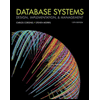
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
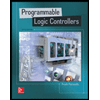
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education