Is anything wrong
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
*
* Create two new files:
* src/spi.c
* include/spi.h
*
* In "spi.c" declare and implement a function spi_init() that will initialise SPI0 in
* unbuffered mode, such that data can be written to the shift register that controls
* the 7-segment display, and which enables the SPI interrupt via the IE bit in INTCTRL.
* You might also like to enable DISP EN as an output and drive it high so the display
* is enabled.
*
* Next, declare and implement a function spi_write(uint8_t b) which will write the
* byte b out via the SPI interface.
*
* Finally, declare and implement an ISR that will handle the SPI interrupt enabled
* above, and which will drive a rising edge onto the DISP LATCH net. You might need
* to add some code to spi_init() if you didn't already enable all the required pins
* as outputs.
*
This is my spi.c
This is my spi.c
#include <avr/io.h>
#include <avr/interrupt.h>
#include "spi.h"
#define DISP_EN_PIN PIN0_bm
#define DISP_LATCH_PIN PIN2_bm
void spi_init(void) {
PORTA.DIRSET = DISP_EN_PIN | DISP_LATCH_PIN; // Enable DISP_EN and DISP_LATCH as output
PORTA.OUTSET = DISP_EN_PIN; // Drive DISP_EN high to enable display
// SPI master mode, unbuffered, MSB first, CPOL=0, CPHA=0, 8-bit data, interrupt enabled
SPI0.CTRLA = SPI_MASTER_bm | SPI_DORD_bm | SPI_MODE_0_gc | SPI_ENABLE_bm | CPUINT_LVL1VEC_gm;
SPI0.CTRLB = SPI_BUFEN_bm;
SPI0.INTCTRL = CPUINT_LVL1VEC_gm;
}
void spi_write(uint8_t b) {
SPI0.DATA = b;
}
ISR(SPI0_RXC_vect) {
// Rising edge on DISP LATCH
PORTC.OUTCLR = PIN0_bm;
PORTC.OUTSET = PIN0_bm;
}
This is spi.h
Is anything wrong
This is spi.h
#ifndef SPI_H
#define SPI_H
void spi_init(void);
void spi_write(uint8_t b);
#endif
Is anything wrong
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
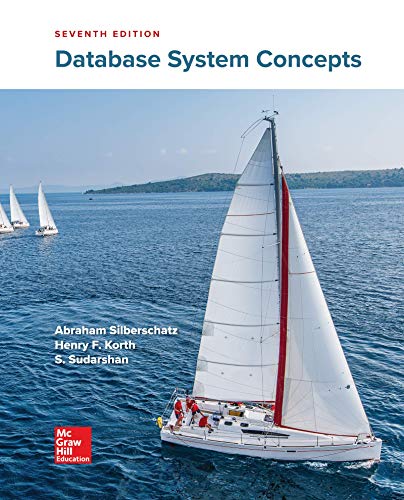
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
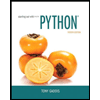
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
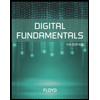
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
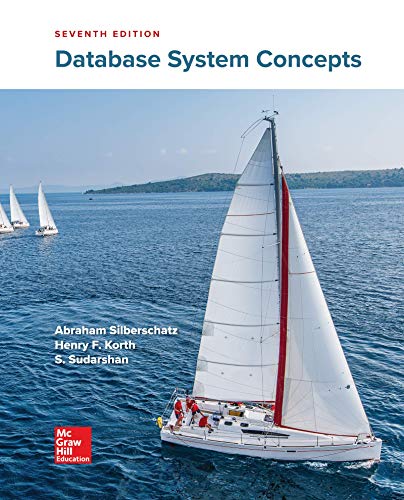
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
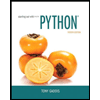
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
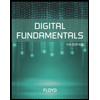
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
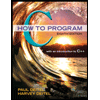
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
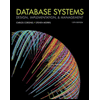
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
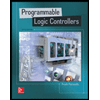
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education