Instructions: 1. Write a java code for the above class diagram for “Car”. 2. MAX_Weight must be a public class constant which is set to 6.4 3. The data member “counter” must be a class member and initialized to zero 4. Use “counter” to assign a unique id for each class instance ( counter++ ) by the constructor 5. The constructor is used to initialize all the instance variables by receiving the values from the user. Don’t change the parameter’s names. 6. “convertMeterIntoMile” and “convertMileIntoMeter” are class methods: a. convertMeterIntoMile: convert the reading into miles by dividing the receiving value by 1.62 b. convertMileIntoMeter: convert the reading into meter by multiplying the receiving value by 1.62 7. The method “computeFuelEconomy” must be overloaded and return a dummy calculation 8. In the main method: a. Create an array of objects to hold five objects of class Car. b. Add them to the array. Note that the code for class Person is included but you need to import it from package “people” c. By using for-loop, check if any of the objects exceeds MAX_Weight or not, and print “heavy car” or light char”, accordingly. d. Use both class methods to convert 100 km into miles and 200 miles into kilometers. e. Using the first object in the array, call all of the computeFuelEconomy methods. 9. Add your code to a package, called “assignment1” 10. Include a clear screenshot of your code and the output.
Instructions:
1. Write a java code for the above class diagram for “Car”.
2. MAX_Weight must be a public class constant which is set to 6.4
3. The data member “counter” must be a class member and initialized to zero
4. Use “counter” to assign a unique id for each class instance ( counter++ ) by the constructor
5. The constructor is used to initialize all the instance variables by receiving the values from the
user. Don’t change the parameter’s names.
6. “convertMeterIntoMile” and “convertMileIntoMeter” are class methods:
a. convertMeterIntoMile: convert the reading into miles by dividing the receiving value by 1.62
b. convertMileIntoMeter: convert the reading into meter by multiplying the receiving value by 1.62
7. The method “computeFuelEconomy” must be overloaded and return a dummy calculation
8. In the main method:
a. Create an array of objects to hold five objects of class Car.
b. Add them to the array. Note that the code for class Person is included but you need to import it from package “people”
c. By using for-loop, check if any of the objects exceeds MAX_Weight or not, and print “heavy car” or light char”, accordingly.
d. Use both class methods to convert 100 km into miles and 200 miles into kilometers.
e. Using the first object in the array, call all of the computeFuelEconomy methods.
9. Add your code to a package, called “assignment1”
10. Include a clear screenshot of your code and the output.
package people;
public class Person{ private String name; private int age;
public Person() { name = "no name"; age = -1;
}
public Person(String name, int age) { this.name = name;
this.age = age; }
public String getName() { return name;
}
public void setName(String n) {
name = n; }
public void setAge(int a) { age = a;
}
public int getAge() { return age;
} }


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

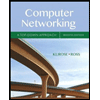
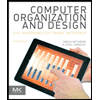
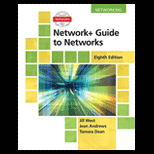
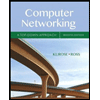
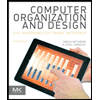
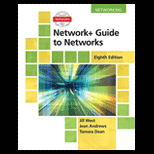
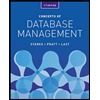
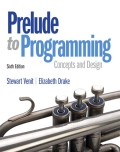
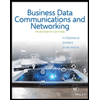