In Python write an address book program using object oriented design, you would need at least two things: An “address book” class. A class that will store information about a “person”. Using knowledge of classes, and thinking constructively about the task assigned with object-oriented design in mind. Requirements: A class named `Person`. Class constructor requires first, middle and last name of your person. Class method `set_email` must exist to set the person’s e-mail address, e.g.` "kirk@starfleet.org".` Class method `get_info` that returns a string formed from a person’s first, middle, last names and e-mail address, in this exact form: `"James Tiberius Kirk ".` Class method `__str__ that returns the same string as the `get_info` method. A class named `AddressBook`. It must do the following: No class constructor arguments are required, though you should consider some internal data structures for initialization. Class method `add_person` which allows you to add a new `Person` object to your address book. Class method `get_people` that will return a list of the `Person` objects you stored in your address book. Your main() function does nothing, and should be: def main(): pass
In Python write an address book program using object oriented design, you would need at least two things:
-
An “address book” class.
-
A class that will store information about a “person”.
Using knowledge of classes, and thinking constructively about the task assigned with object-oriented design in mind.
Requirements:
-
A class named `Person`.
-
Class constructor requires first, middle and last name of your person.
-
Class method `set_email` must exist to set the person’s e-mail address, e.g.` "kirk@starfleet.org".`
-
Class method `get_info` that returns a string formed from a person’s first, middle, last names and e-mail address, in this exact form: `"James Tiberius Kirk <kirk@starfleet.org>".`
-
Class method `__str__ that returns the same string as the `get_info` method.
-
-
A class named `AddressBook`. It must do the following:
-
No class constructor arguments are required, though you should consider some internal data structures for initialization.
-
Class method `add_person` which allows you to add a new `Person` object to your address book.
-
Class method `get_people` that will return a list of the `Person` objects you stored in your address book.
-
- Your main() function does nothing, and should be:
- def main():
- pass

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

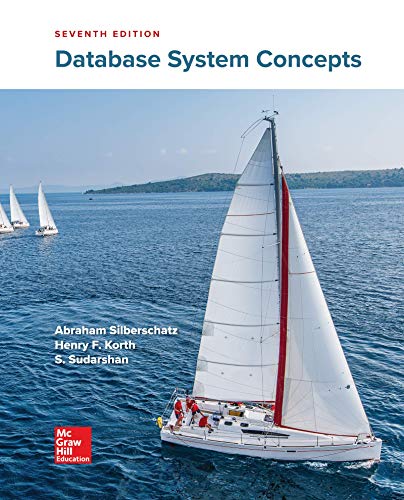
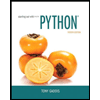
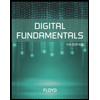
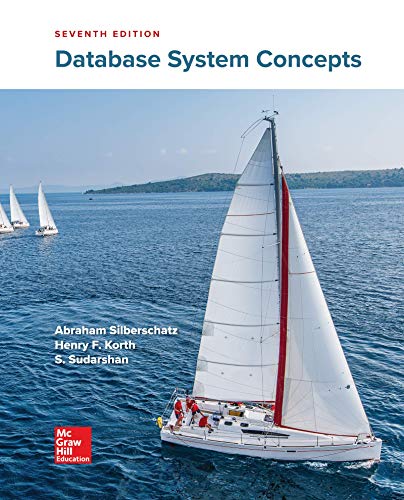
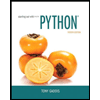
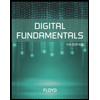
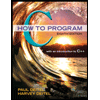
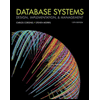
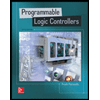