Information is present in the screenshot and below. Based on that need help in solving the code for this problem in python or java. The time complexity has to be as less as possible (nlogn or n at best, no n^2). Hint: Use inversion index nlogn approach Output Format The output consists of one line containing an integer indicating the number of quantum blasts per second that Butz must fire at Swapper to prevent him from sorting the books in the worst-case scenario (best case for Swapper). If this number is not a whole number, just to prevent Swapper from ever succeeding, round up to the nearest whole number. If it is impossible for Butz to stop Swapper from restoring order to the shelf, print "Butz loses!" without quotes. Sample Input 0 4 3 4 1 3 2 Sample Output 0 2 Sample Input 1 5 3 2 4 0 3 1 Sample Output 1 1 Sample Input 2 6 5 5 0 1 2 4 3 Sample Output 2 1 The actual code in Python def solve(books,n,s): # TODO: Compute and print the answer here pass def main(): n, s = list(map(int,input().strip().split(" "))) books = list(map(int,input().strip().split(" "))) solve(books,n,s) if __name__ == "__main__": main() The actual code in java import java.io.BufferedReader; import java.io.InputStreamReader; public class c { public static void main(String[] args) throws Exception { BufferedReader br = new BufferedReader(new InputStreamReader(System.in)); StringBuilder sb = new StringBuilder(); String[] parts = br.readLine().trim().split(" "); int n = Integer.parseInt(parts[0]); int s = Integer.parseInt(parts[1]); int[] books = new int[n]; parts = br.readLine().trim().split(" "); for(int i = 0; i < n; i++) { books[i] = Integer.parseInt(parts[i]); } solve(books,n,s); } public static void solve(int[] books, int n, int s){ // TODO: Compute and print answer here } }
Information is present in the screenshot and below. Based on that need help in solving the code for this problem in python or java. The time complexity has to be as less as possible (nlogn or n at best, no n^2).
Hint: Use inversion index nlogn approach
Output Format
The output consists of one line containing an integer indicating the number of quantum blasts per second that Butz must fire at Swapper to prevent him from sorting the books in the worst-case scenario (best case for Swapper). If this number is not a whole number, just to prevent Swapper from ever succeeding, round up to the nearest whole number. If it is impossible for Butz to stop Swapper from restoring order to the shelf, print "Butz loses!" without quotes.
Sample Input 0
4 1 3 2
Sample Output 0
Sample Input 1
2 4 0 3 1
Sample Output 1
Sample Input 2
5 0 1 2 4 3
Sample Output 2
The actual code in Python
def solve(books,n,s): def main(): if __name__ == "__main__": |
The actual code in java
import java.io.BufferedReader; public class c { for(int i = 0; i < n; i++) { public static void solve(int[] books, int n, int s){ |



Step by step
Solved in 4 steps

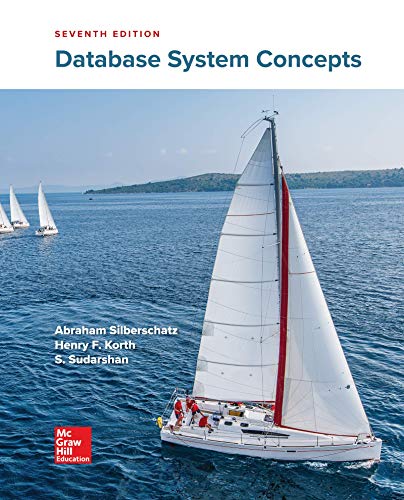
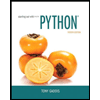
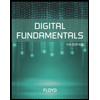
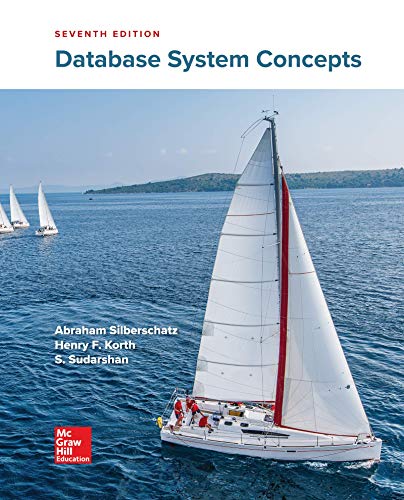
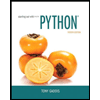
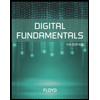
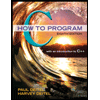
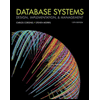
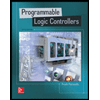