include #include #include const int MAX_NAME_SIZE = 50; void swap(char **x, char *
Can you assist me in finding the error in my code. My output does not equal the expected output as shown in the image. My code is written in C and is as follows
#include <stdlib.h>
#include <stdio.h>
#include <string.h>
const int MAX_NAME_SIZE = 50;
void swap(char **x, char **y)
{
char *temp;
temp = *x;
*x = *y;
*y = temp;
}
void AllPermutations(char**permList, int permSize, char**nameList, int nameSize){
if(permSize == nameSize - 1){
for(int i = 0; i < nameSize; i++)
{
if(i==nameSize-1)
printf("%s", nameList[i]);
else
printf("%s, ", nameList[i]);
}
printf("\n");
}
else{
for(int i = permSize; i < nameSize; i++){
swap(nameList + permSize, nameList+i);
AllPermutations(permList, permSize+1, nameList, nameSize);
swap(nameList+permSize, nameList+i);
}
}
}
int main(void) {
int size;
int i = 0;
char name[MAX_NAME_SIZE];
scanf("%d", &size);
char *nameList[size];
char *permList[size];
for (i = 0; i < size; ++i) {
nameList[i] = (char *)malloc(MAX_NAME_SIZE);
scanf("%s", name);
strcpy(nameList[i], name);
}
AllPermutations(permList, 0, nameList, size);
// Free dynamically allocated memory
for (i = 0; i < size; ++i) {
free(nameList[i]);
}
return 0;
}


Step by step
Solved in 3 steps with 2 images

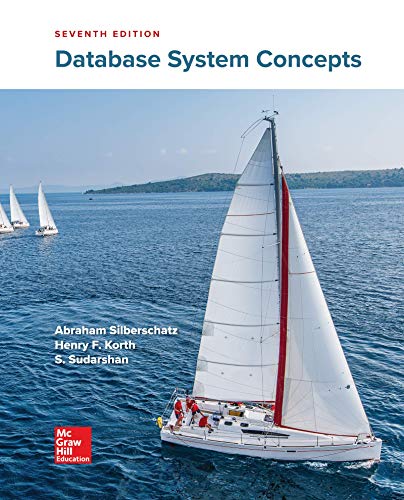
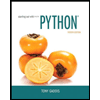
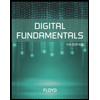
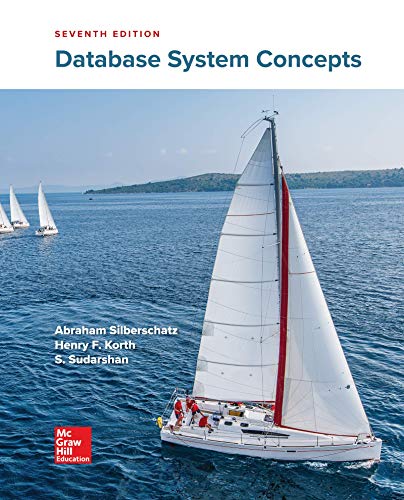
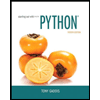
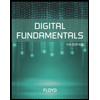
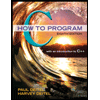
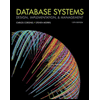
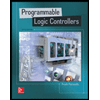