#include <stdio.h> #include <stdlib.h> #include <unistd.h> int global_var = 42; // int* function(int *a) { int local_var = 10; // *a = *a + local_var; int *local_pointer = (int *)malloc (size of (int) * 64); // Allocated array with 64 integers return local_pinter; } int main() { int local_main[1024*1024*1024*1024*1024] = {0}; // initialize an array and set all items as 0 int *heap_var = (int *)malloc(size of(int) * 16); // Allocated array with 16 integers *heap_var = 100; function(heap_var); printf(“the value is %d\n”, *heap_var); free(heap_var); // release the memory return 0; } 1) draw the memory layout of the created process, which should include text, data, heap and stack [2 marks]. 2) Indicate which section the variables are allocated [2 marks]: global_var local_var, local_pointer local_main heap_var, *heap_var (the data that it points to) 3) Does this code have memory leaking (heap memory is not released)? [2 marks] 4) The local_main take tiny memory space on stack, and it can succeed to run (suppose the stack size is 1GB on OS). True or False? [2 marks]
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
int global_var = 42; //
int* function(int *a) {
int local_var = 10; //
*a = *a + local_var;
int *local_pointer = (int *)malloc (size of (int) * 64); // Allocated array with 64 integers
return local_pinter;
}
int main() {
int local_main[1024*1024*1024*1024*1024] = {0}; // initialize an array and set all items as 0
int *heap_var = (int *)malloc(size of(int) * 16); // Allocated array with 16 integers
*heap_var = 100;
function(heap_var);
printf(“the value is %d\n”, *heap_var);
free(heap_var); // release the memory
return 0;
}
1) draw the memory layout of the created process, which should include text, data, heap and stack [2 marks].
2) Indicate which section the variables are allocated [2 marks]:
- global_var
- local_var, local_pointer
- local_main
- heap_var, *heap_var (the data that it points to)
3) Does this code have memory leaking (heap memory is not released)? [2 marks]
4) The local_main take tiny memory space on stack, and it can succeed to run (suppose the stack size is 1GB on OS). True or False? [2 marks]

Step by step
Solved in 2 steps with 1 images

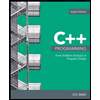
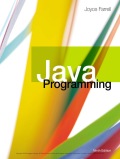
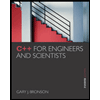
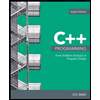
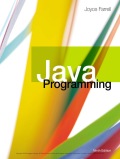
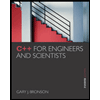
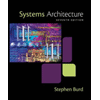
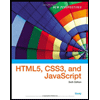