In this task, we're going to be creating an Email Simulator using OOP. Follow the instruction and fill in the rest of logic to fulfil the below program requirements. • Open the file called email.py. Create an Email class and initialise a constructor that takes in three arguments: o email_address - the email address of the sender (for Inbox emails) or recipient (for Outbox emails). o subject_line - the subject of the email. o email_contents - the content of the email. The email class should contain two instance-level class variables with default values: o has been read-initialised to False. o is_spam-initialised to False. • The Email class should also contain the following class methods to edit the values of the email objects: o mark_as_read which should change has been read to True. o mark_as_spam which should change is_spam to True. Initialise the following empty lists to store, and access, the email objects: (note that you can have a list of objects) o Inbox o Outbox Create the following functions to add functionality to your email simulator: o populate_inbox() - a function which creates an email object with the email address, subject line and contents, and stores it in the Inbox list. Take note: At program start-up, this function should be used to populate your Inbox with three sample emails for further use in your program. Note that this function does not need to be included as a menu option for the user.
In this task, we're going to be creating an Email Simulator using OOP. Follow the instruction and fill in the rest of logic to fulfil the below program requirements. • Open the file called email.py. Create an Email class and initialise a constructor that takes in three arguments: o email_address - the email address of the sender (for Inbox emails) or recipient (for Outbox emails). o subject_line - the subject of the email. o email_contents - the content of the email. The email class should contain two instance-level class variables with default values: o has been read-initialised to False. o is_spam-initialised to False. • The Email class should also contain the following class methods to edit the values of the email objects: o mark_as_read which should change has been read to True. o mark_as_spam which should change is_spam to True. Initialise the following empty lists to store, and access, the email objects: (note that you can have a list of objects) o Inbox o Outbox Create the following functions to add functionality to your email simulator: o populate_inbox() - a function which creates an email object with the email address, subject line and contents, and stores it in the Inbox list. Take note: At program start-up, this function should be used to populate your Inbox with three sample emails for further use in your program. Note that this function does not need to be included as a menu option for the user.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question

Transcribed Image Text:<
HHyperion Dev
Hyperion
●
o list_emails () - a function that loops through the Inbox and prints
the email's subject_line, along with a corresponding number. For
example, if there are three emails in the Inbox:
O
1
2
Welcome to Hyperion Dev!
Great work on the bootcamp!
Your excellent marks!
This function can be used to list the messages when the user
chooses to read, mark as spam, and delete an email.
o read_email() - a function that displays a selected email, together
with the email_address, subject_line, and email_contents, and then
sets its has been_read instance variable to True.
For this, allow the user to input an index i.e. read_email(i) prints the
email stored at position i in the list. Following the example above, an
index of 0 will print the email with the subject line "Welcome to
Hyperion Dev!".
Copyright © 2021 Hyperion Dev. All rights reserved.
o mark_spam() - a function which, using the same indexing method as
above, sets its mark_as_spam instance variable to True.
O delete_email() - a function which, using the same indexing method
as above, deletes an email from the inbox.
o send_email() - a function which creates a new email object and
"sends" it to the Outbox.
Your task is to build out the Class, Methods, Lists, and functions to get
everything working! Fill in the rest of the logic for what should happen
when the user chooses to:
1. Read an email
2. Mark an email as spam
3. Delete an email
4. View unread emails
5. View spam emails
6. Send an email
7. Quit application
Note that menu option 5 and 6 does not require a function. Access
the corresponding class variables to retrieve the subject_line only.
Tip: The enumerate() function will be handy for this task.
● Keep the readability of print outputs in mind and take initiative to show
SE L1T28 - Introduction to OOP I - Classes.pdf
100% +
חר
12 of 14
3 of 4
hts reserved.
Q loop
1 of 1
X
>

Transcribed Image Text:<
Hyperion Dev
HHyperion
Compulsory Task 1
In this task, we're going to be creating an Email Simulator using OOP. Follow
the instruction and fill in the rest of logic to fulfil the below program
requirements.
● Open the file called email.py.
Create an Email class and initialise a constructor that takes in three
arguments:
O
email address the email address of the sender (for Inbox emails) or
recipient (for Outbox emails).
O subject_line - the subject of the email.
O email_contents - the content of the email.
●
● The email class should contain two instance-level class variables with
default values:
o has been_read - initialised to False.
O is_spam-initialised to False.
Copyright © 2021 Hyperion Dev. All rights reserved.
● The Email class should also contain the following class methods to
edit the values of the email objects:
O mark_as_read which should change has_been_read to True.
O mark_as_spam which should change is_spam to True.
Initialise the following empty lists to store, and access, the email objects:
(note that you can have a list of objects)
O Inbox
O Outbox
Create the following functions to add functionality to your email simulator:
O populate_inbox() - a function which creates an email object with the
email address, subject line and contents, and stores it in the Inbox list.
Take note: At program start-up, this function should be used to
populate your Inbox with three sample emails for further use in your
program. Note that this function does not need to be included as a
menu option for the user.
SE L1T28 - Introduction to OOP I - Classes.pdf
100% +
חר
11 of 14
3 of 4
hts reserved.
Q loop
1 of 1
X
>
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 11 images

Recommended textbooks for you
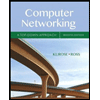
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
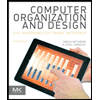
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
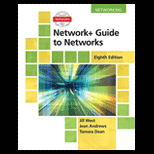
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
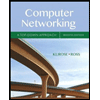
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
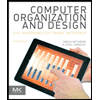
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
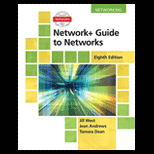
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
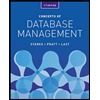
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
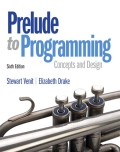
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
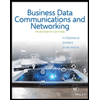
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY