In the code editor, you are provided with an initial code that asks the user for an integer input and passes this to a function call of the digitMon() function. The digitMon() function is a recursive function which has the following description: Return type - int Name - digitMon Parameters - one integer Description - this function is a recursive function that computes the DigitMon of the passed integer. The digitMon() function is already partially implemented. Your task is to fill in the blanks to make it work.
In C Language please..
6. DigitMon
by CodeChum Admin
When I was a kid, I used to play Digimon.
Now that I'm a programmer and I have this weird passion of digits, I want to combine them both to create the ultimate program: DigitMon!
This DigitMon program would take an integer input and would output the sum of all the digits of the number. For example, if the input is 243, the output would be 9 because 2 + 4 + 3 = 9. In this case, we say that the DigitMon of 243 is 9.
Instructions:
- In the code editor, you are provided with an initial code that asks the user for an integer input and passes this to a function call of the digitMon() function.
-
The digitMon() function is a recursive function which has the following description:
- Return type - int
- Name - digitMon
- Parameters - one integer
- Description - this function is a recursive function that computes the DigitMon of the passed integer.
- The digitMon() function is already partially implemented. Your task is to fill in the blanks to make it work.
Input
1. Integer to be processed
Source Code:
#include<stdio.h>
int digitMon(int);
int main(void) {
int n;
printf("Enter n: ");
scanf("%d", &n);
printf("DigitMon of %d is %d", n, digitMon(n));
return 0;
}
int digitMon(int n) {
// TODO: Fill in the blanks
if(n == __) {
return __;
} else {
return (n % 10) + __;
}
}
Output

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

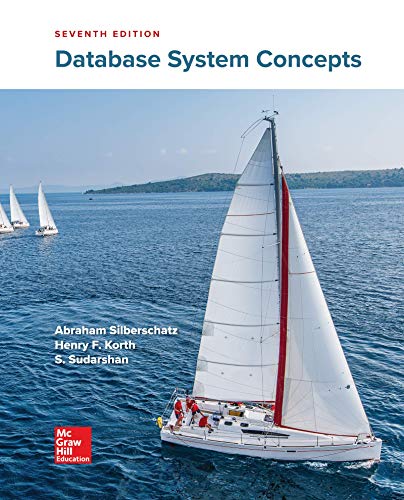
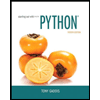
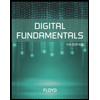
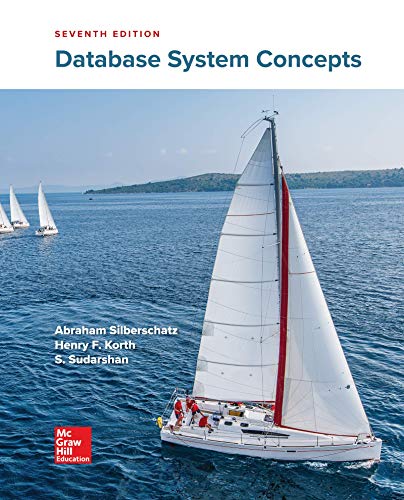
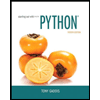
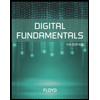
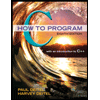
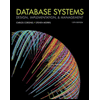
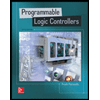