In the code editor, you are provided with an initial code in the main() that asks the user for the elements of a 5x5 2D array and a call to a function called flex2D(). Do not edit anything in the main() function. Your task is to implement the function definition of the flex2D() with the following details: Return type - void Function name - flex2D Parameters - int[][5] Definition - prints the elements of each of the row twice. Input 1. Elements of a 5x5 2D array Output Enter the elements: 1 9 2 3 4 8 2 4 0 1 0 3 1 3 4 9 2 3 1 5 8 8 1 7 6 Flex: 1 9 2 3 4 1 9 2 3 4 8 2 4 0 1 8 2 4 0 1 0 3 1 3 4 0 3 1 3 4 9 2 3 1 5 9 2 3 1 5 8 8 1 7 6 8 8 1 7 6
5. Flexing My 2D
by CodeChum Admin
I'm a pro-grammer and I want to flex to the world my 2D arrays in an extraordinary way! Ha-ha-ha!
Instructions:
In the code editor, you are provided with an initial code in the main() that asks the user for the elements of a 5x5 2D array and a call to a function called flex2D(). Do not edit anything in the main() function.
Your task is to implement the function definition of the flex2D() with the following details:
Return type - void
Function name - flex2D
Parameters - int[][5]
Definition - prints the elements of each of the row twice.
Input
1. Elements of a 5x5 2D array
Output
Enter the elements:
1 9 2 3 4
8 2 4 0 1
0 3 1 3 4
9 2 3 1 5
8 8 1 7 6
Flex:
1 9 2 3 4
1 9 2 3 4
8 2 4 0 1
8 2 4 0 1
0 3 1 3 4
0 3 1 3 4
9 2 3 1 5
9 2 3 1 5
8 8 1 7 6
8 8 1 7 6
![Output
main.c
< >
+ c
Test Cases
1
#include<stdio.h>
Enter the elements:
3
void flex2D(int[][5]);
ÇE Run Tests
1.9-2-3-4
4
8-2-4 0 1
5- int main(void) {
int matrix[5][5];
e- 3-1-3-4
7
Test Case
9-2-3-1.5
printf("Enter the elements:\n");
for(int row = 0; row < 5; row++) {
for(int col = 0; col < 5; col++) {
scanf("%d", &matrix[row][col]);
}
}
8
1
8.8 17 6
9
10
Flex:
11
1.9- 2-3-4
12
Test Case
13
2
1.9 2 3-4
14
8-2-4 0 1
15
printf("\nFlex:\n");
8-2-4 0 1
16
e- 3-1.3-4
17
flex2D(matrix);
Test
a. 3.1.3.4
18
O Case
Hidden
19
return 0;
20 }
3
Score: 0/10
21
22 // TODO: Implement the function definition](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F82304115-cf1d-40b3-864e-0ed64499952f%2Fde140973-51b3-4e58-96a9-a4b113bc448d%2Fqoguld1a_processed.png&w=3840&q=75)
![8-2 4 0 1
main.c
+
Test Cases
e 3 1.3-4
9.2 3 15
4
5- int main(void) {
int matrix[5][5];
8-8 1-7-6
CE Run Tests
6
7
Flex:
printf("Enter the elements:\n");
for(int row = 0; row
for(int col = 0; col < 5; col++) {
scanf("%d", &matrix[row][col]);
}
}
1.9 2 3 4
5; row++) {
Test Case
10
1.9 2-3-4
11
1
8-2-4 0 1
12
8. 2-4 0 1
13
e 3 13-4
14
e- 3-1-3-4
15
printf("\nFlex:\n");
Test Case
16
2
9 2-3-1-5
17
flex2D(matrix);
9 2-3-1-5
18
8.8 1.7-6
19
return 0;
20 }
Test
8-8 1.7-6
21
O Case
Hidden
// TODO: Implement the function definition
23 - void flex2D(int arrO[5]) {
22
3
Score: 0/10
24
25 }
>](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F82304115-cf1d-40b3-864e-0ed64499952f%2Fde140973-51b3-4e58-96a9-a4b113bc448d%2Ffhu15rq_processed.png&w=3840&q=75)

Step by step
Solved in 3 steps with 2 images

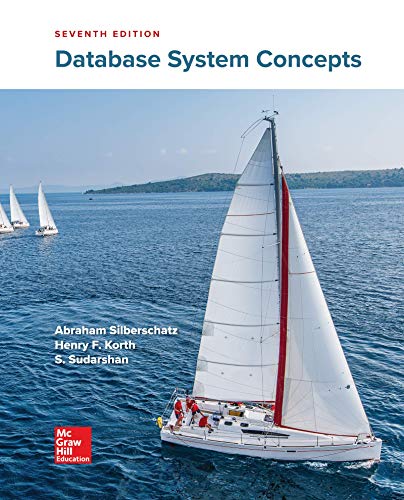
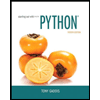
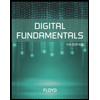
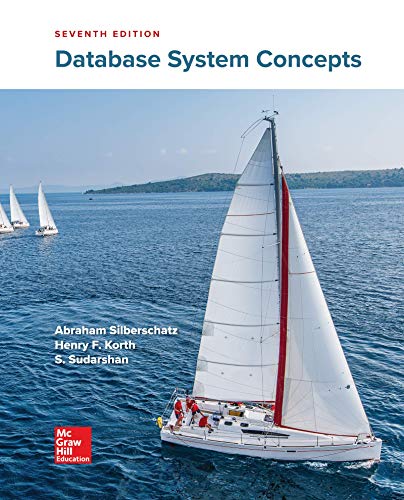
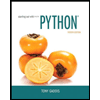
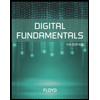
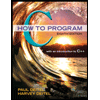
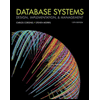
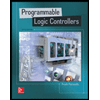