In Python Requirements: In your own words, describe the problem including input and output. In your own words, Describe the major steps for solving the problem. Name and Email Addresses Write a program that keeps names and email addresses in a dictionary as key-value pairs. The program should display a menu that lets the user look up a person’s email address, add a new name and email address, change an existing email address, and delete an existing name and email address. The program should save the data stored in a dictionary to a file when the user exits the program. Each time the program starts, it should retrieve the data from the file and store it in a dictionary. You must create and use at least 5 meaningful functions. A function to display a menu A function to look up a person’s email address A function to add a new name and email address A function to change an email address A function to delete a name and email address. Your program should check user input; for example, if the user wants to delete a name that does not exist in the dictionary, you should print something like: the name is not in the database. A sample one run is shown below. Output Example Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program Enter your choice: 2 Enter name: John Enter email address: John@yahoo.com That name already exists Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program Enter your choice: 2 Enter name: Jack Enter email address: Jack@yahoo.com Name and address have been added Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program Enter your choice: 1 Enter a name: Sam The specified name was not found Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program Enter your choice: 1 Enter a name: Jack Name: Jack Email: Jack@yahoo.com Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program Enter your choice: 3 Enter name: John Enter the new address: John@wayne.edu Information updated Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program Enter your choice: 1 Enter a name: John Name: John Email: John@wayne.edu Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program Enter your choice: 4 Enter name: Sam The specified name was not found Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program Enter your choice: 4 Enter name: Jack Information deleted Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program Enter your choice: 1 Enter a name: Jack The specified name was not found Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program Enter your choice: 5 Information saved >>>
In Python
Requirements:
- In your own words, describe the problem including input and output.
- In your own words, Describe the major steps for solving the problem.
Name and Email Addresses
Write a
The program should display a menu that lets the user look up a person’s email address, add a new name and email address, change an existing email address, and delete an existing name and email address. The program should save the data stored in a dictionary to a file when the user exits the program. Each time the program starts, it should retrieve the data from the file and store it in a dictionary.
You must create and use at least 5 meaningful functions.
- A function to display a menu
- A function to look up a person’s email address
- A function to add a new name and email address
- A function to change an email address
- A function to delete a name and email address.
Your program should check user input; for example, if the user wants to delete a name that does not exist in the dictionary, you should print something like: the name is not in the
Output Example
Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program
Enter your choice: 2 Enter name: John Enter email address: John@yahoo.com That name already exists
Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program
Enter your choice: 2 Enter name: Jack Enter email address: Jack@yahoo.com Name and address have been added
Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program
Enter your choice: 1 Enter a name: Sam The specified name was not found
Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program
Enter your choice: 1 Enter a name: Jack Name: Jack Email: Jack@yahoo.com
Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program
Enter your choice: 3 Enter name: John Enter the new address: John@wayne.edu Information updated
Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program
Enter your choice: 1 Enter a name: John Name: John Email: John@wayne.edu
Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program
Enter your choice: 4 Enter name: Sam The specified name was not found
Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program
Enter your choice: 4 Enter name: Jack Information deleted
Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program
Enter your choice: 1 Enter a name: Jack The specified name was not found
Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program
Enter your choice: 5 Information saved >>> |

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

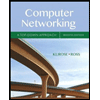
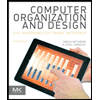
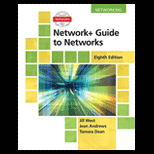
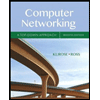
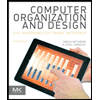
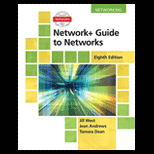
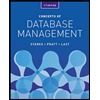
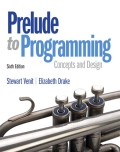
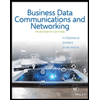