please use c# i just want you to modify the code please Download the solution and run it locally. Familiarize yourself with the code, then modify it as follows: 1. Replace the use of depth first search with the breadth first search algorithm. 2. Add at least 5 more employees // The code below was mostly taken directly from: // https://www.csharpstar.com/csharp-depth-first-seach-using-list/ using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace depthFirstSearch { class Program { public class Employee { public Employee(string name) { this.name = name; } public string name { get; set; } public List Employees { get { return EmployeesList; } } public void isEmployeeOf(Employee e) { EmployeesList.Add(e); } List EmployeesList = new List(); public override string ToString() { return name; } } public class DepthFirstAlgorithm { public Employee BuildEmployeeGraph() { Employee Eva = new Employee("Eva"); Employee Sophia = new Employee("Sophia"); Employee Brian = new Employee("Brian"); Eva.isEmployeeOf(Sophia); Eva.isEmployeeOf(Brian); Employee Lisa = new Employee("Lisa"); Employee Tina = new Employee("Tina"); Employee John = new Employee("John"); Employee Mike = new Employee("Mike"); Sophia.isEmployeeOf(Lisa); Sophia.isEmployeeOf(John); Brian.isEmployeeOf(Tina); Brian.isEmployeeOf(Mike); return Eva; } public Employee Search(Employee root, string nameToSearchFor) { if (nameToSearchFor == root.name) return root; Employee personFound = null; for (int i = 0; i < root.Employees.Count; i++) { personFound = Search(root.Employees[i], nameToSearchFor); if (personFound != null) break; } return personFound; } public void Traverse(Employee root) { Console.WriteLine(root.name); for (int i = 0; i < root.Employees.Count; i++) { Traverse(root.Employees[i]); } } } static void Main(string[] args) { DepthFirstAlgorithm b = new DepthFirstAlgorithm(); Employee root = b.BuildEmployeeGraph(); Console.WriteLine("Traverse Graph\n------"); b.Traverse(root); Console.WriteLine("\nSearch in Graph\n------"); Employee e = b.Search(root, "Eva"); Console.WriteLine(e == null ? "Employee not found" : e.name); e = b.Search(root, "Brian"); Console.WriteLine(e == null ? "Employee not found" : e.name); e = b.Search(root, "Soni"); Console.WriteLine(e == null ? "Employee not found" : e.name); } } }
please use c#
i just want you to modify the code please
// The code below was mostly taken directly from:
// https://www.csharpstar.com/csharp-depth-first-seach-using-list/
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace depthFirstSearch
{
class
{
public class Employee
{
public Employee(string name)
{
this.name = name;
}
public string name { get; set; }
public List<Employee> Employees
{
get
{
return EmployeesList;
}
}
public void isEmployeeOf(Employee e)
{
EmployeesList.Add(e);
}
List<Employee> EmployeesList = new List<Employee>();
public override string ToString()
{
return name;
}
}
public class DepthFirstAlgorithm
{
public Employee BuildEmployeeGraph()
{
Employee Eva = new Employee("Eva");
Employee Sophia = new Employee("Sophia");
Employee Brian = new Employee("Brian");
Eva.isEmployeeOf(Sophia);
Eva.isEmployeeOf(Brian);
Employee Lisa = new Employee("Lisa");
Employee Tina = new Employee("Tina");
Employee John = new Employee("John");
Employee Mike = new Employee("Mike");
Sophia.isEmployeeOf(Lisa);
Sophia.isEmployeeOf(John);
Brian.isEmployeeOf(Tina);
Brian.isEmployeeOf(Mike);
return Eva;
}
public Employee Search(Employee root, string nameToSearchFor)
{
if (nameToSearchFor == root.name)
return root;
Employee personFound = null;
for (int i = 0; i < root.Employees.Count; i++)
{
personFound = Search(root.Employees[i], nameToSearchFor);
if (personFound != null)
break;
}
return personFound;
}
public void Traverse(Employee root)
{
Console.WriteLine(root.name);
for (int i = 0; i < root.Employees.Count; i++)
{
Traverse(root.Employees[i]);
}
}
}
static void Main(string[] args)
{
DepthFirstAlgorithm b = new DepthFirstAlgorithm();
Employee root = b.BuildEmployeeGraph();
Console.WriteLine("Traverse Graph\n------");
b.Traverse(root);
Console.WriteLine("\nSearch in Graph\n------");
Employee e = b.Search(root, "Eva");
Console.WriteLine(e == null ? "Employee not found" : e.name);
e = b.Search(root, "Brian");
Console.WriteLine(e == null ? "Employee not found" : e.name);
e = b.Search(root, "Soni");
Console.WriteLine(e == null ? "Employee not found" : e.name);
}
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 6 images

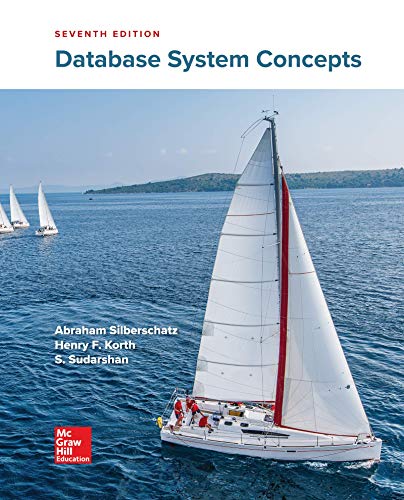
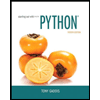
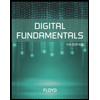
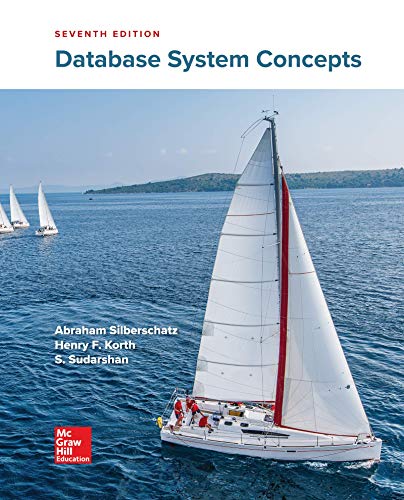
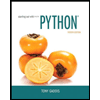
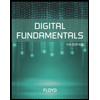
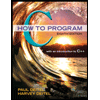
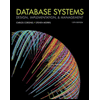
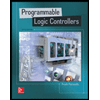