in c++ To gain a better understanding of polymorphic and virtual functions start with the following simple example. Notice we have not defined a virtual function yet. // Part1.h. Note, this header file will have TWO classes declared. #include using namespace std; class Base { public: void testFunction (); }; class Derived : public Base { public: void testFunction (); }; // Part1.cpp. Note this implementation file will have TWO class defined. #include "Part1.h" void Base::testFunction () { cout << "Base class" << endl; } void Derived::testFunction () { cout << "Derived class" << endl; } // main.cpp #include "Part1.h" int main() { Base* ptr = new Base; ptr -> testFunction (); // prints "Base class" delete ptr; ptr = new Derived; ptr -> testFunction (); // prints "Base class" because the base class // function is not virtual delete ptr; return 0; } Now modify the code with the following (all other code should remain the same). class Base { public: virtual void testFunction (); }; Compile and run your program with this modification. You’ll notice the second testFunction() call generates the message “Derived class”. Welcome to polymorphism! No need to submit anything for this part of the lab, just make sure that virtual is clear to you.
in c++
- To gain a better understanding of polymorphic and virtual functions start with the following simple example. Notice we have not defined a virtual function yet.
// Part1.h. Note, this header file will have TWO classes declared.
#include <iostream>
using namespace std;
class Base
{
public:
void testFunction ();
};
class Derived : public Base
{
public:
void testFunction ();
};
// Part1.cpp. Note this implementation file will have TWO class defined.
#include "Part1.h"
void Base::testFunction ()
{
cout << "Base class" << endl;
}
void Derived::testFunction ()
{
cout << "Derived class" << endl;
}
// main.cpp
#include "Part1.h"
int main()
{
Base* ptr = new Base;
ptr -> testFunction (); // prints "Base class"
delete ptr;
ptr = new Derived;
ptr -> testFunction (); // prints "Base class" because the base class
// function is not virtual
delete ptr;
return 0;
}
Now modify the code with the following (all other code should remain the same).
class Base
{
public:
virtual void testFunction ();
};
Compile and run your program with this modification. You’ll notice the second testFunction() call generates the message “Derived class”. Welcome to polymorphism! No need to submit anything for this part of the lab, just make sure that virtual is clear to you.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps

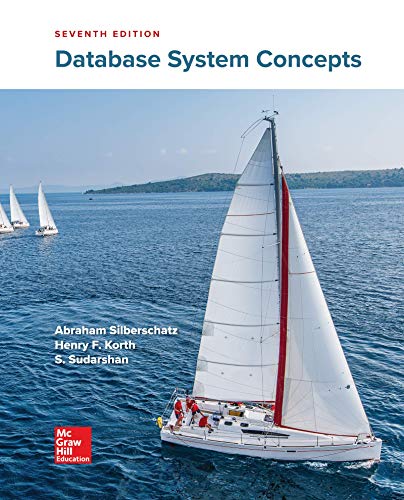
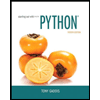
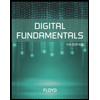
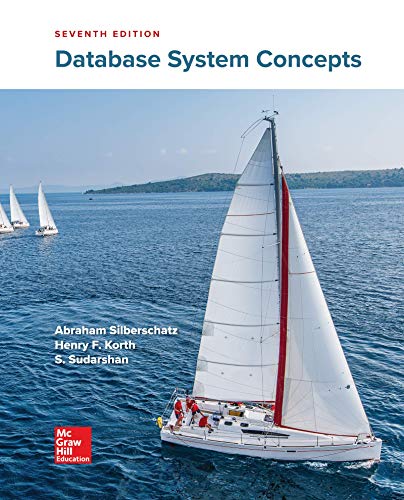
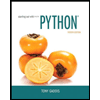
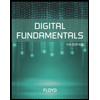
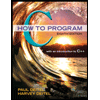
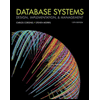
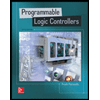