Given this class specification: Class: BankAccount Data members: int balance Write these function definitions: 1. An external definition of a member function that can be a const member function. 2. An external definition for a member function that should not be a const member function.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
paste indedte code....need help with C++


In C++ , if the member function is defined inside the class definition it can be defined directly ,but if its defined outside the class, then we have to use the scope resolution : : operator along with class name and function name.
Here in the following code,getValue has been made a const member function, which means we can call it on any const objects. For member functions defined outside of the class definition, the const keyword must be used on both the function prototype in the definition and on the function definition.
Algorithm:
1,Defining the class name
2.Defining variable name balance of type int
3. Defining constructor
4.Defining constant member function
5.Printing the value in the main function
1.
#include<iostream>
using namespace std;
class BankAccount {
int balance;
public:
BankAccount (int b=0)
{
balance = b;
}
int getValue() const;
};
int BankAccount::getValue() const
{
return cout<<"Balance is:", balance;
}
int main()
{
BankAccount t(20);
cout<<t.getValue();
return 0;
}
Output: 200000
Step by step
Solved in 5 steps with 2 images

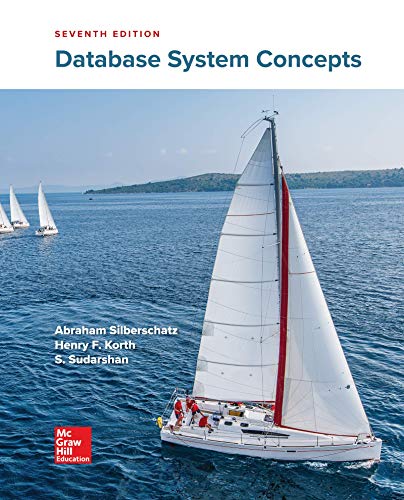
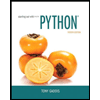
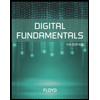
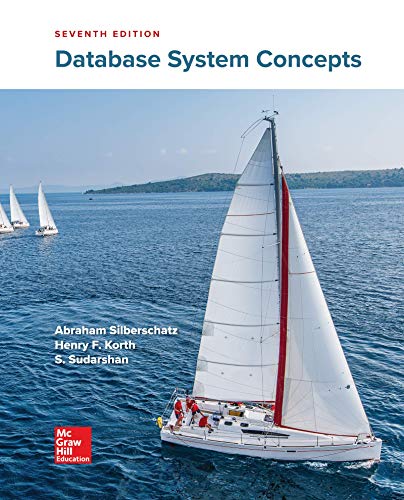
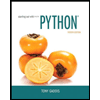
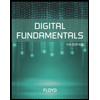
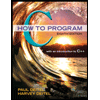
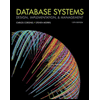
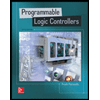