Using C++ add to the below code to pick an operator and define it for your class. Include the header of the operator function like Fraction operator+(Fraction frac) You should include the function header for both member function and (if possible) nonmember function implementation. #include using namespace std; class MobilePhone { private: std::string aabrand; std::string aamodel; int aastorageCapacity; public: MobilePhone(std::string b, std::string m, int s); std::string getBrand(); std::string getModel(); int getStorageCapacity(); }; MobilePhone::MobilePhone(std::string b, std::string m, int s) { aabrand = b; aamodel = m; aastorageCapacity = s; } std::string MobilePhone::getBrand() { return aabrand; } std::string MobilePhone::getModel() { return aamodel; } int MobilePhone::getStorageCapacity() { return aastorageCapacity; } int main() { MobilePhone myPhone("Apple", "iPhone 12 ProMax", 256); std::cout << "Brand: " << myPhone.getBrand() << std::endl; std::cout << "Model: " << myPhone.getModel() << std::endl; std::cout << "Storage capacity: " << myPhone.getStorageCapacity() << "GB" << std::endl; return 0; }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Using C++ add to the below code to pick an operator and define it for your class. Include the header of the operator function like
Fraction operator+(Fraction frac)
You should include the function header for both member function and (if possible) nonmember function implementation.
#include <iostream>
using namespace std;
class MobilePhone {
private:
std::string aabrand;
std::string aamodel;
int aastorageCapacity;
public:
MobilePhone(std::string b, std::string m, int s);
std::string getBrand();
std::string getModel();
int getStorageCapacity();
};
MobilePhone::MobilePhone(std::string b, std::string m, int s) {
aabrand = b;
aamodel = m;
aastorageCapacity = s;
}
std::string MobilePhone::getBrand() {
return aabrand;
}
std::string MobilePhone::getModel() {
return aamodel;
}
int MobilePhone::getStorageCapacity() {
return aastorageCapacity;
}
int main() {
MobilePhone myPhone("Apple", "iPhone 12 ProMax", 256);
std::cout << "Brand: " << myPhone.getBrand() << std::endl;
std::cout << "Model: " << myPhone.getModel() << std::endl;
std::cout << "Storage capacity: " << myPhone.getStorageCapacity() << "GB" << std::endl;
return 0;
}

Step by step
Solved in 4 steps with 2 images

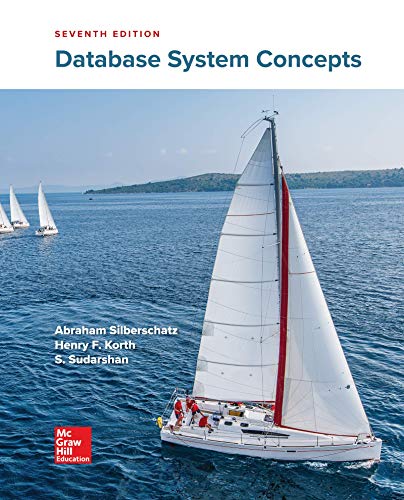
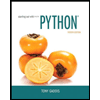
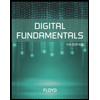
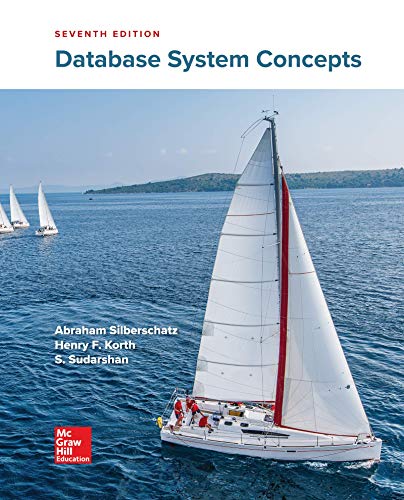
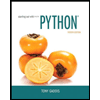
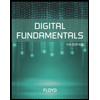
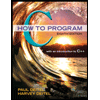
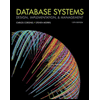
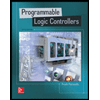