In C programming: Complete the program to compute the number of quarter, dime, nickel, and penny coins that equal total cents, using the fewest coins (i.e., using the most possible of a larger coin first). Please use Structs. #include #include void ComputeChange(int totCents, int *quarters, int *dimes, int*nickles, int*pennies) { printf("%d\n", totCents); int y,x; *quarters=totCents/25; x=pennies-*quarters; *dimes=x/10; y=*dimes - x; *nickles=y/10; *pennies=*nickles - y; } int main(void) { int totalCents; // Total amount of change needed int quartersChange; // Number of quarters used for change int dimesChange; // Number of dimes used for change int nickelsChange; // Number of nickels used for change int penniesChange; // Number of pennies used for change totalCents = 67; ComputeChange(totalCents, &quartersChange, &dimesChange, &nickelsChange, &penniesChange); printf("Change for %d cents is:\n", totalCents); printf("%d quarters\n", quartersChange); printf("%d dimes\n", dimesChange); printf("%d nickels\n", nickelsChange); printf("%d pennies\n", penniesChange); return 0; }
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
In C programming: Complete the program to compute the number of quarter, dime, nickel, and penny coins that equal total cents, using the fewest coins (i.e., using the most possible of a larger coin first). Please use Structs.
#include <stdio.h>
#include <stdlib.h>
void ComputeChange(int totCents, int *quarters, int *dimes, int*nickles, int*pennies) {
printf("%d\n", totCents);
int y,x;
*quarters=totCents/25;
x=pennies-*quarters;
*dimes=x/10;
y=*dimes - x;
*nickles=y/10;
*pennies=*nickles - y;
}
int main(void) {
int totalCents; // Total amount of change needed
int quartersChange; // Number of quarters used for change
int dimesChange; // Number of dimes used for change
int nickelsChange; // Number of nickels used for change
int penniesChange; // Number of pennies used for change
totalCents = 67;
ComputeChange(totalCents, &quartersChange, &dimesChange, &nickelsChange, &penniesChange);
printf("Change for %d cents is:\n", totalCents);
printf("%d quarters\n", quartersChange);
printf("%d dimes\n", dimesChange);
printf("%d nickels\n", nickelsChange);
printf("%d pennies\n", penniesChange);
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

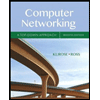
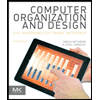
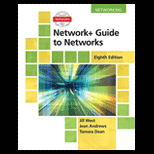
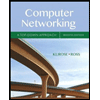
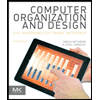
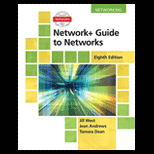
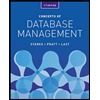
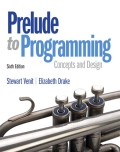
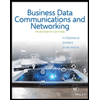