In C++ Plz LAB: Book information (overriding member functions) Given main() and a base Book class, define a derived class called Encyclopedia with member functions to get and set private class data members of the following types: string to store the edition int to store the number of pages Within the derived Encyclopedia class, define a PrintInfo() member function that overrides the Book class' PrintInfo() function by printing the title, author, publisher, publication date, edition, and number of pages. Ex. If the input is: The Hobbit J. R. R. Tolkien George Allen & Unwin 21 September 1937 The Illustrated Encyclopedia of the Universe Ian Ridpath Watson-Guptill 2001 2nd 384 the output is: Book Information: Book Title: The Hobbit Author: J. R. R. Tolkien Publisher: George Allen & Unwin Publication Date: 21 September 1937 Book Information: Book Title: The Illustrated Encyclopedia of the Universe Author: Ian Ridpath Publisher: Watson-Guptill Publication Date: 2001 Edition: 2nd Number of Pages: 384 Note: Indentations use 3 spaces. main.cpp (Unable to edit): #include "Book.h" #include "Encyclopedia.h" #include #include using namespace std; int main() { Book myBook; Encyclopedia myEncyclopedia; string title, author, publisher, publicationDate; string eTitle, eAuthor, ePublisher, ePublicationDate, edition; int numPages; getline(cin, title); getline(cin, author); getline(cin, publisher); getline(cin, publicationDate); getline(cin, eTitle); getline(cin, eAuthor); getline(cin, ePublisher); getline(cin, ePublicationDate); getline(cin, edition); cin >> numPages; myBook.SetTitle(title); myBook.SetAuthor(author); myBook.SetPublisher(publisher); myBook.SetPublicationDate(publicationDate); myBook.PrintInfo(); myEncyclopedia.SetTitle(eTitle); myEncyclopedia.SetAuthor(eAuthor); myEncyclopedia.SetPublisher(ePublisher); myEncyclopedia.SetPublicationDate(ePublicationDate); myEncyclopedia.SetEdition(edition); myEncyclopedia.SetNumPages(numPages); myEncyclopedia.PrintInfo(); return 0; } Book.h (Unable to edit): #ifndef BOOKH #define BOOKH #include using namespace std; class Book { public: void SetTitle(string userTitle); string GetTitle(); void SetAuthor(string userAuthor); string GetAuthor(); void SetPublisher(string userPublisher); string GetPublisher(); void SetPublicationDate(string userPublicationDate); string GetPublicationDate(); void PrintInfo(); protected: string title; string author; string publisher; string publicationDate; }; #endif Book.cpp (Unable to edit): #include "Book.h" #include void Book::SetTitle(string userTitle) { title = userTitle; } string Book::GetTitle() { return title; } void Book::SetAuthor(string userAuthor) { author = userAuthor; } string Book::GetAuthor() { return author; } void Book::SetPublisher(string userPublisher) { publisher = userPublisher; } string Book::GetPublisher() { return publisher; } void Book::SetPublicationDate(string userPublicationDate) { publicationDate = userPublicationDate; } string Book::GetPublicationDate() { return publicationDate; } void Book::PrintInfo() { cout << "Book Information: " << endl; cout << " Book Title: " << title << endl; cout << " Author: " << author << endl; cout << " Publisher: " << publisher << endl; cout << " Publication Date: " << publicationDate << endl; } Encyclopedia.h (Able to edit): #ifndef ENCYCLOPEDIAH #define ENCYCLOPEDIAH #include "Book.h" class Encyclopedia : public Book { // TODO: Declare mutator functions - // SetEdition(), SetNumPages() // TODO: Declare accessor functions - // GetEdition(), GetNumPages() // TODO: Declare a PrintInfo() function that overrides // the PrintInfo() in Book class // TODO: Declare private data members }; #endif Encyclopedia.cpp #include "Encyclopedia.h" #include // Define functions declared in Encyclopedia.h
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
In C++ Plz
LAB: Book information (overriding member functions)
Given main() and a base Book class, define a derived class called Encyclopedia with member functions to get and set private class data members of the following types:
string to store the edition
int to store the number of pages
Within the derived Encyclopedia class, define a PrintInfo() member function that overrides the Book class' PrintInfo() function by printing the title, author, publisher, publication date, edition, and number of pages.
Ex. If the input is:
The Hobbit J. R. R. Tolkien George Allen & Unwin 21 September 1937 The Illustrated Encyclopedia of the Universe Ian Ridpath Watson-Guptill 2001 2nd 384
the output is:
Book Information: Book Title: The Hobbit Author: J. R. R. Tolkien Publisher: George Allen & Unwin Publication Date: 21 September 1937 Book Information: Book Title: The Illustrated Encyclopedia of the Universe Author: Ian Ridpath Publisher: Watson-Guptill Publication Date: 2001 Edition: 2nd Number of Pages: 384
Note: Indentations use 3 spaces.
main.cpp (Unable to edit):
#include "Book.h"
#include "Encyclopedia.h"
#include <string>
#include <iostream>
using namespace std;
int main() {
Book myBook;
Encyclopedia myEncyclopedia;
string title, author, publisher, publicationDate;
string eTitle, eAuthor, ePublisher, ePublicationDate, edition;
int numPages;
getline(cin, title);
getline(cin, author);
getline(cin, publisher);
getline(cin, publicationDate);
getline(cin, eTitle);
getline(cin, eAuthor);
getline(cin, ePublisher);
getline(cin, ePublicationDate);
getline(cin, edition);
cin >> numPages;
myBook.SetTitle(title);
myBook.SetAuthor(author);
myBook.SetPublisher(publisher);
myBook.SetPublicationDate(publicationDate);
myBook.PrintInfo();
myEncyclopedia.SetTitle(eTitle);
myEncyclopedia.SetAuthor(eAuthor);
myEncyclopedia.SetPublisher(ePublisher);
myEncyclopedia.SetPublicationDate(ePublicationDate);
myEncyclopedia.SetEdition(edition);
myEncyclopedia.SetNumPages(numPages);
myEncyclopedia.PrintInfo();
return 0;
}
Book.h (Unable to edit):
#ifndef BOOKH
#define BOOKH
#include <string>
using namespace std;
class Book {
public:
void SetTitle(string userTitle);
string GetTitle();
void SetAuthor(string userAuthor);
string GetAuthor();
void SetPublisher(string userPublisher);
string GetPublisher();
void SetPublicationDate(string userPublicationDate);
string GetPublicationDate();
void PrintInfo();
protected:
string title;
string author;
string publisher;
string publicationDate;
};
#endif
Book.cpp (Unable to edit):
#include "Book.h"
#include <iostream>
void Book::SetTitle(string userTitle) {
title = userTitle;
}
string Book::GetTitle() {
return title;
}
void Book::SetAuthor(string userAuthor) {
author = userAuthor;
}
string Book::GetAuthor() {
return author;
}
void Book::SetPublisher(string userPublisher) {
publisher = userPublisher;
}
string Book::GetPublisher() {
return publisher;
}
void Book::SetPublicationDate(string userPublicationDate) {
publicationDate = userPublicationDate;
}
string Book::GetPublicationDate() {
return publicationDate;
}
void Book::PrintInfo() {
cout << "Book Information: " << endl;
cout << " Book Title: " << title << endl;
cout << " Author: " << author << endl;
cout << " Publisher: " << publisher << endl;
cout << " Publication Date: " << publicationDate << endl;
}
Encyclopedia.h (Able to edit):
#ifndef ENCYCLOPEDIAH
#define ENCYCLOPEDIAH
#include "Book.h"
class Encyclopedia : public Book {
// TODO: Declare mutator functions -
// SetEdition(), SetNumPages()
// TODO: Declare accessor functions -
// GetEdition(), GetNumPages()
// TODO: Declare a PrintInfo() function that overrides
// the PrintInfo() in Book class
// TODO: Declare private data members
};
#endif
Encyclopedia.cpp
#include "Encyclopedia.h"
#include <iostream>
// Define functions declared in Encyclopedia.h

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

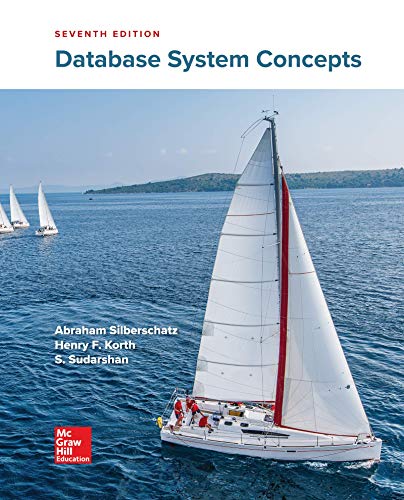
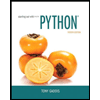
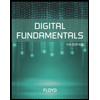
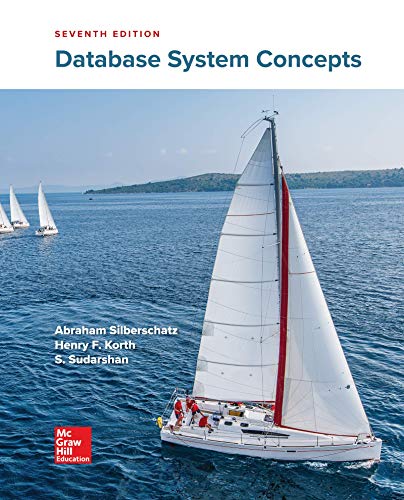
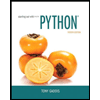
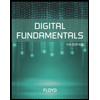
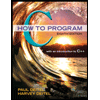
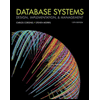
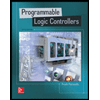