In c++ please Write the RemoveEvens() function, which receives a vector of integers as a parameter and returns a new vector of integers containing only the odd numbers from the original vector. The main program outputs values of the returned vector. Hint: If the original vector has even numbers, then the new vector will be smaller in length than the original vector and should have no blank element. Ex: If the vector passed to the RemoveEvens() function is [1, 2, 3, 4, 5, 6, 7, 8, 9], then the function returns and the program output is: [1, 3, 5, 7, 9] Ex: If the vector passed to the RemoveEvens() function is [1, 9, 3], hen the function returns and the program output is: [1, 9, 3]
In c++ please
Write the RemoveEvens() function, which receives a
Hint: If the original vector has even numbers, then the new vector will be smaller in length than the original vector and should have no blank element.
Ex: If the vector passed to the RemoveEvens() function is [1, 2, 3, 4, 5, 6, 7, 8, 9], then the function returns and the program output is:
[1, 3, 5, 7, 9]
Ex: If the vector passed to the RemoveEvens() function is [1, 9, 3], hen the function returns and the program output is:
[1, 9, 3]
#include <iostream>
#include <vector>
using namespace std;
vector<int> RemoveEvens(vector<int> nums) {
/* Type your code here */
}
int main() {
vector<int> input(9);
input.at(0) = 1;
input.at(1) = 2;
input.at(2) = 3;
input.at(3) = 4;
input.at(4) = 5;
input.at(5) = 6;
input.at(6) = 7;
input.at(7) = 8;
input.at(8) = 9;
vector<int> result = RemoveEvens(input); // Should return [1, 3, 5, 7, 9]
for (size_t i = 0; i < result.size(); ++i) {
cout << result.at(i);
}
cout << endl;
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

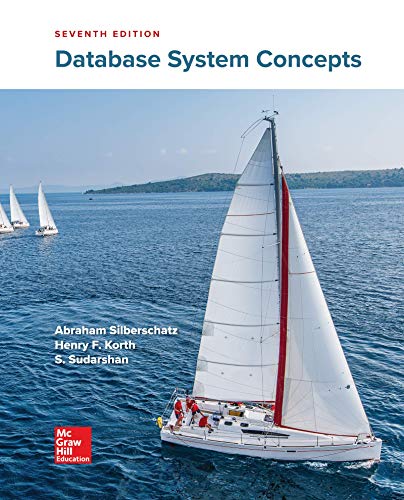
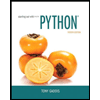
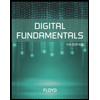
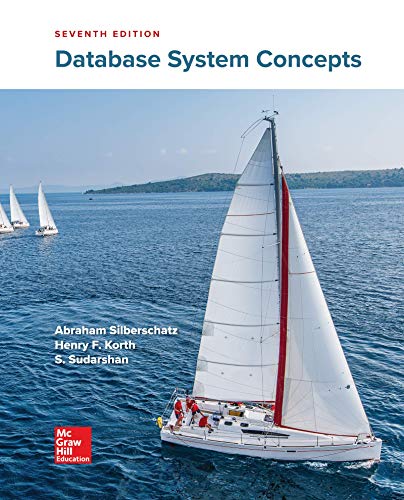
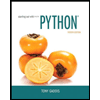
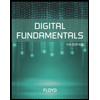
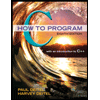
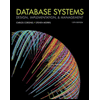
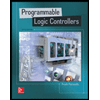