Create a program that dynamically creates a vector whose size depends on the user's preference. Pass the vector to a CalculateAverage function that is responsible for computing the average GPA of the given vector of GPAs.
Class Average GPA
Create a program that dynamically creates a
CalculateAverage
Implement a function called CalculateAverage in calculate_avg.cc that calculates the average of a double vector and returns that average.
CalculateAverage() will have one parameter, a std::vector<double>& referring to the vector.
When the vector size is greater than 0, the function should calculate the average GPA from the given vector of grades.
However, when the size of the vector is 0, then the function should return 0.
main
The main function has mostly been built for you. It is your task to:
- Construct a double vector based on the inputted class size.
- Store each student's GPAs into the vector.
- Pass the vector as input to CalculateAverage to compute the average GPA of the class.
- Display the students' average GPA. Read the instructions in main.cc for more details.
If the user happens to provide a class size of 0, then the program should output "You have no students!" and then end the execution of the program without attempting to calculate the average.
Place the CalculateAverage's function's' implementation in calculate_avg.cc. We have provided the prototype in calculate_avg.h.
Sample Output
How many students are in your class? 5 Enter the GPA for the students in your class (0.0 - 4.0) Enter the GPA for student #1: 3.8 Enter the GPA for student #2: 2.5 Enter the GPA for student #3: 4.0 Enter the GPA for student #4: 1.9 Enter the GPA for student #5: 3.6 Class average: 3.16 How many students are in your class? 0 You have no students!
main.cc file
#include <iomanip> | |
#include <iostream> | |
int main() { | |
int num_students = 0; | |
std::cout << "How many students are in your class? "; | |
std::cin >> num_students; | |
if (num_students <= 0) { | |
std::cout << "You have no students!\n"; | |
} else { | |
// ===================== YOUR CODE HERE ===================== | |
// Construct a vector whose size is the same as the | |
// given number of students in the class, based on the | |
// `num_students` variable. Don't forget to #include <vector>. | |
// ========================================================== | |
std::cout << "Enter the GPA for the students in your class (0.0 - 4.0)\n"; | |
for (int i = 0; i < num_students; i++) { | |
// ============ YOUR CODE HERE ============ | |
// Store the input GPA from cin into | |
// the corresponding index in the vector. | |
// ======================================== | |
std::cout << "Enter the GPA for student #" << i + 1 << ": "; | |
} | |
double average = 0; | |
// ===================== YOUR CODE HERE ===================== | |
// Call your CalculateAverage function and provide any | |
// necessary parameters. Then, store the results in the average | |
// variable. Make sure to #include "calculate_avg.h" before you | |
// use the CalculateAverage function. | |
// ========================================================== | |
std::cout << "Class average: " << std::setprecision(2) << std::fixed | |
<< average << "\n"; | |
} | |
return0; | |
} |
calculate_avg.cc file
#include <vector> | |
double CalculateAverage(const std::vector<double> &student_grades) { | |
// ================= YOUR CODE HERE ================= | |
// Compute and return the average grade | |
// based on the input vector containing student GPAs. | |
// Hint: you may want to use a range based loop! | |
// ================================================== | |
return0.0; | |
} |
calculate_avg.h file
#include <vector> | |
// This is the function declaration for the function you | |
// will implement in the implementation file, calculate_avg.cc | |
// You do not need to edit this file. | |
double CalculateAverage(const std::vector<double> &student_grades); |

Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 2 images

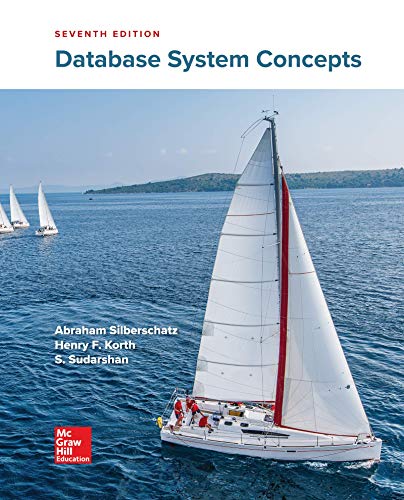
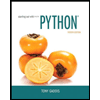
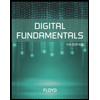
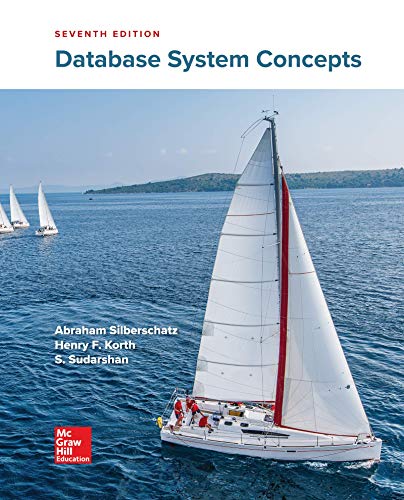
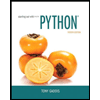
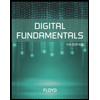
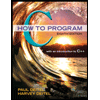
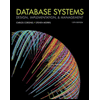
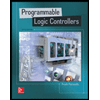