in c++ i need to make it where the the code skips over the leading blanks in the string for example "0077" would mean the value of the BigNumber object will be 46 and the number of digits will be 2 #include
in c++ i need to make it where the the code skips over the leading blanks in the string for example "0077" would mean the value of the BigNumber object will be 46 and the number of digits will be 2
#include <iostream>
#include <string>
using namespace std;
class BigNumber {
friend ostream& operator<<(ostream & out, const BigNumber & c);
private:
int digits[1000];
int numDigits;
public:
BigNumber(const string &s);
};
ostream& operator<<(ostream & out, const BigNumber & c) {
if (c.numDigits == 0) out << "0";
else
for (int i = c.numDigits - 1; i >= 0; i--)
out << c.digits[i];
//out << "<" << c.numDigits << ">";
return out;
}
BigNumber::BigNumber(const string &s)
{
int i = 0;
int j = 0;
string Holder;
while (s[i] != '\0')
{
if(s[i] != '0')
{
numDigits++;
Holder += s[i];
}
i++;
}
while(Holder[j] != '\0')
{
digits[j] = Holder[j];
j++;
}
}
int main() {
string s;
cin >> s;
cout << "Creating a BigNumber object n using the string constructor. " << endl;
cout << "In this test the string's value is " << s << "." << endl;
BigNumber n(s);
cout << "Outputting the value of n. ";
cout << "n = " << n << "." << endl;
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

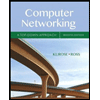
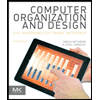
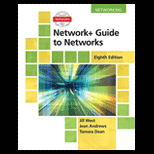
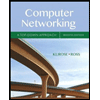
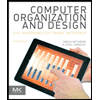
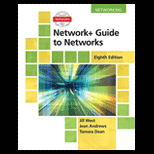
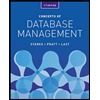
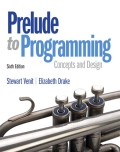
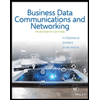