import numpy as npfrom scipy.optimize import minimizeimport matplotlib.pyplot as pltimport pickle #Implement the function calAnaliticalSolution def calAnaliticalSolution(X,y): # Inputs: # X = N x d # y = N x 1 # Output: # w = d x 1 (which is the parameter calculated from data X and label y using alalytical solution) # IMPLEMENT THIS METHOD - REMOVE THE NEXT LINE w = np.zeros((X.shape[0],1)) return w #Implement the function calRegressionError def calRegressionError(w,Xtest,ytest): # Inputs: # w = d x 1 # Xtest = N x d # ytest = N x 1 # Output: # mse = scalar value (which is the error of the regression model) # IMPLEMENT THIS METHOD - REMOVE THE NEXT LINE mse = 0 return mse Xtrain,ytrain,Xtest,ytest = pickle.load(open('diabetes.pickle','rb')) x1 = np.ones((len(Xtrain),1))x2 = np.ones((len(Xtest),1))Xtrain_i = np.concatenate((np.ones((Xtrain.shape[0],1)), Xtrain), axis=1)Xtest_i = np.concatenate((np.ones((Xtest.shape[0],1)), Xtest), axis=1)w = calAnaliticalSolution(Xtrain,ytrain)w_i = calAnaliticalSolution(Xtrain_i,ytrain)mse = calRegressionError(w,Xtrain,ytrain)mse_i = calRegressionError(w_i,Xtrain_i,ytrain)print('MSE without intercept on train data - %.2f'%mse)print('MSE with intercept on train data - %.2f'%mse_i)mse = calRegressionError(w,Xtest,ytest)mse_i = calRegressionError(w_i,Xtest_i,ytest)print('MSE without intercept on test data - %.2f'%mse)print('MSE with intercept on test data - %.2f'%mse_i)
import numpy as np
from scipy.optimize import minimize
import matplotlib.pyplot as plt
import pickle
#Implement the function calAnaliticalSolution
def calAnaliticalSolution(X,y):
# Inputs:
# X = N x d
# y = N x 1
# Output:
# w = d x 1 (which is the parameter calculated from data X and label y using alalytical solution)
# IMPLEMENT THIS METHOD - REMOVE THE NEXT LINE
w = np.zeros((X.shape[0],1))
return w
#Implement the function calRegressionError
def calRegressionError(w,Xtest,ytest):
# Inputs:
# w = d x 1
# Xtest = N x d
# ytest = N x 1
# Output:
# mse = scalar value (which is the error of the regression model)
# IMPLEMENT THIS METHOD - REMOVE THE NEXT LINE
mse = 0
return mse
Xtrain,ytrain,Xtest,ytest = pickle.load(open('diabetes.pickle','rb'))
x1 = np.ones((len(Xtrain),1))
x2 = np.ones((len(Xtest),1))
Xtrain_i = np.concatenate((np.ones((Xtrain.shape[0],1)), Xtrain), axis=1)
Xtest_i = np.concatenate((np.ones((Xtest.shape[0],1)), Xtest), axis=1)
w = calAnaliticalSolution(Xtrain,ytrain)
w_i = calAnaliticalSolution(Xtrain_i,ytrain)
mse = calRegressionError(w,Xtrain,ytrain)
mse_i = calRegressionError(w_i,Xtrain_i,ytrain)
print('MSE without intercept on train data - %.2f'%mse)
print('MSE with intercept on train data - %.2f'%mse_i)
mse = calRegressionError(w,Xtest,ytest)
mse_i = calRegressionError(w_i,Xtest_i,ytest)
print('MSE without intercept on test data - %.2f'%mse)
print('MSE with intercept on test data - %.2f'%mse_i)

Step by step
Solved in 2 steps

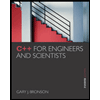
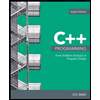
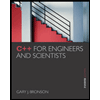
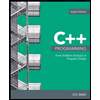