Implement the __getitem__ method so that it returns an element of the sparse array with the same notation as a numpy array. Note that: - if i or j are outside the size of the array you have to issue an AssertionError. - if the element (i,j) exists in the internal dictionary (self.rows) the stored value must be returned. - if it does not exist, the value 0 must be returned. Complete the code: def SparseMatrix4(m=None, **kwargs): import itertools def add_to_dict(d, key1, key2, val): <... YOUR CODE HERE ...> return d class SparseMatrix4_class: def __init__(self, m=m, **kwargs): <... YOUR CODE HERE ...> def __getitem__(self, pos): i,j = pos assert <... YOUR CODE HERE ...> return <... YOUR CODE HERE ...> return SparseMatrix4_class (**kwargs) To check the code manually: def random_sparse_matrix(size): m = np.random.randint(2, size=size) m = m * np.random.randint(10,size=size) return m m = random_sparse_matrix((5,3)) print("original matrix shape", m.shape) print(m) import itertools s = SparseMatrix4(m) print("inner dict", s.rows) print("\ncoord value_in_m value_in_s") for i,j in itertools.product(list(range(m.shape[0])), list(range(m.shape[1]))): print("(%d,%d) %5d %5d"%(i,j, m[i,j], s[i,j]))
Implement the __getitem__ method so that it returns an element of the sparse array with the same notation as a numpy array. Note that:
- if i or j are outside the size of the array you have to issue an AssertionError.
- if the element (i,j) exists in the internal dictionary (self.rows) the stored value must be returned.
- if it does not exist, the value 0 must be returned.
Complete the code:
def SparseMatrix4(m=None, **kwargs):
import itertools
def add_to_dict(d, key1, key2, val):
<... YOUR CODE HERE ...>
return d
class SparseMatrix4_class:
def __init__(self, m=m, **kwargs):
<... YOUR CODE HERE ...>
def __getitem__(self, pos):
i,j = pos
assert <... YOUR CODE HERE ...>
return <... YOUR CODE HERE ...>
return SparseMatrix4_class (**kwargs)
To check the code manually:
def random_sparse_matrix(size):
m = np.random.randint(2, size=size)
m = m * np.random.randint(10,size=size)
return m
m = random_sparse_matrix((5,3))
print("original matrix shape", m.shape)
print(m)
import itertools
s = SparseMatrix4(m)
print("inner dict", s.rows)
print("\ncoord value_in_m value_in_s")
for i,j in itertools.product(list(range(m.shape[0])), list(range(m.shape[1]))):
print("(%d,%d) %5d %5d"%(i,j, m[i,j], s[i,j]))

Step by step
Solved in 4 steps with 2 images

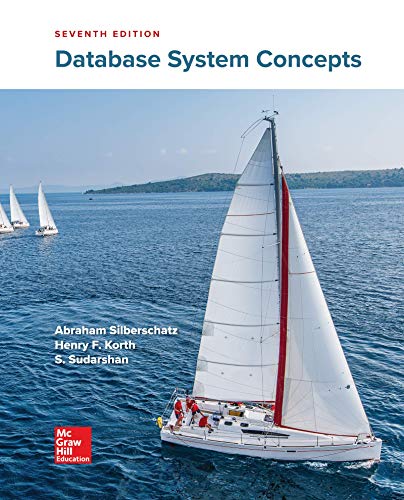
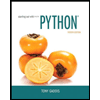
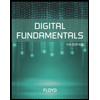
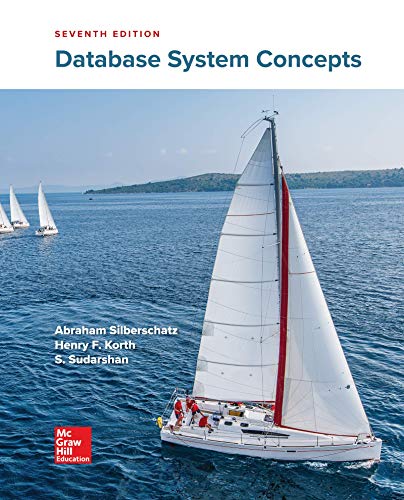
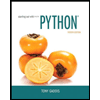
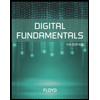
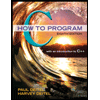
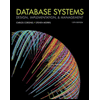
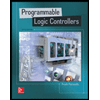