Using HTML and JavaScript do the following: Implement Insertion Sort 1. Non-increasing order, 2. At least an array of 10 elements., 3. You can use a static array.
Using HTML and JavaScript do the following:
Implement Insertion Sort
1. Non-increasing order,
2. At least an array of 10 elements.,
3. You can use a static array.

<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Insertion sort</title>
</head>
<body>
<p>List Before sorting :</p>
<h1 id="beforeArray"></h1>
<p>List After sorting :(Non-increasing order)</p>
<h1 id="afterArray"></h1>
</body>
<script>
//static list
var list = [ 3,54,6,7,9,2,43,23,36,76,87,45,65];
//show data in list
data = "";
for (i = 0; i < list.length; i++) {
if (i == 0) {
data = list[i];
} else {
data += " " + list[i]
}
}
document.getElementById('beforeArray').innerHTML = data;
//Sorting start
//start sorting
var i, key, j;
for (i = 1; i < list.length; i++) {
key = list[i];
j = i - 1;
while (j >= 0 && list[j] < key) {
list[j + 1] = list[j];
j = j - 1;
}
list[j + 1] = key;
}
//Sorting ends
for (i = 0; i < list.length; i++) {
if (i == 0) {
data = list[i];
} else {
data += " " + list[i]
}
}
document.getElementById('afterArray').innerHTML = data;
</script>
</html>
Step by step
Solved in 2 steps with 1 images

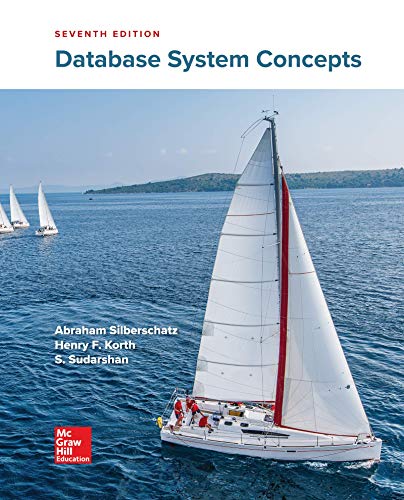
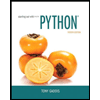
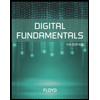
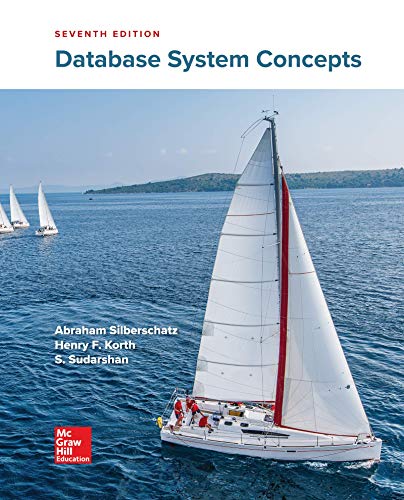
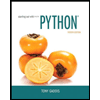
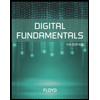
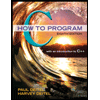
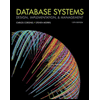
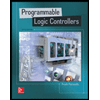