We can create a numpy array from separate lists by specifying a format for each field: roster = np.zeros(4, dtype={'names': ('name', 'age', 'major', 'gpa'), 'formats': ('U50', 'i4', 'U4', 'f8')}) roster['name'] = ['Alice', 'Bob', 'Carol', 'Dennis'] roster['age'] = [21, 25, 18, 29] roster['major'] = ['CS', 'Math', 'Chem', 'Phys'] roster['gpa'] = [3.8, 3.2, 4.0, 3.5] where U50 is a string of max length 50, i4 is a 4-byte integer, U4 is a string of max length 4, and f8 is an 8-byte floating-point number. Then roster has value array([('Alice', 21, 'CS', 3.8), ('Bob', 25, 'Math', 3.2), ('Carol', 18, 'Chem', 4. ), ('Dennis', 29, 'Phys', 3.5)], dtype=[('name', '
We can create a numpy array from separate lists by specifying a format for each field:
roster = np.zeros(4, dtype={'names': ('name', 'age', 'major', 'gpa'), 'formats': ('U50', 'i4', 'U4', 'f8')})
roster['name'] = ['Alice', 'Bob', 'Carol', 'Dennis']
roster['age'] = [21, 25, 18, 29]
roster['major'] = ['CS', 'Math', 'Chem', 'Phys']
roster['gpa'] = [3.8, 3.2, 4.0, 3.5]
where U50 is a string of max length 50, i4 is a 4-byte integer, U4 is a string of max length 4, and f8 is an 8-byte floating-point number. Then roster has value
array([('Alice', 21, 'CS', 3.8), ('Bob', 25, 'Math', 3.2),
('Carol', 18, 'Chem', 4. ), ('Dennis', 29, 'Phys', 3.5)],
dtype=[('name', '<U50'), ('age', '<i4'), ('major', '<U4'), ('gpa', '<f8')])
Then, for example, roster['name'] will give an array of just the student names.
Write a program that reads the numpy array roster we just created that has this format:
name,age,major,gpa
Convert this to a numpy array as shown above. Then use that array to compute and print each of the following:
- The average GPA of all students
- The maximum GPA of students majoring in CS
- The number of students with a GPA over 3.5
- The average GPA of students who are at least 25 years old
- The major that has the highest average GPA among students at most 22 years old
For example, for the four students listed, your program should print:
3.625
3.8
2
3.35
Chem

1. Create the roster array using numpy with student data including name, age, major, and GPA.
2. Define a function, safe_mean(arr), that calculates the mean while handling empty slices:
- If the length of arr is 0, return NaN.
- Otherwise, calculate the mean using np.nanmean.
3. Calculate the average GPA of all students:
- Use safe_mean to compute the mean of roster['gpa'].
4. Calculate the maximum GPA of students majoring in CS:
- Filter students majoring in CS and calculate the maximum GPA.
- Handle empty slices using safe_mean.
5. Calculate the number of students with a GPA over 3.5:
- Use np.sum to count the number of students with GPA > 3.5.
6. Calculate the average GPA of students who are at least 25 years old:
- Filter students with age >= 25, and calculate the average GPA.
- Handle empty slices using safe_mean.
7. Calculate the major with the highest average GPA among students at most 22 years old:
- Iterate through unique majors.
- For each major, filter students in that major and at most 22 years old.
- Calculate the average GPA using,safe_mean.
8. Print the results:
- Print the average GPA of all students.
- Print the maximum GPA of CS students.
- Print the number of students with GPA > 3.5.
- Print the average GPA of students aged 25 or older.
- Print the major with the highest average GPA among students aged 22 or younger.
Step by step
Solved in 4 steps with 2 images

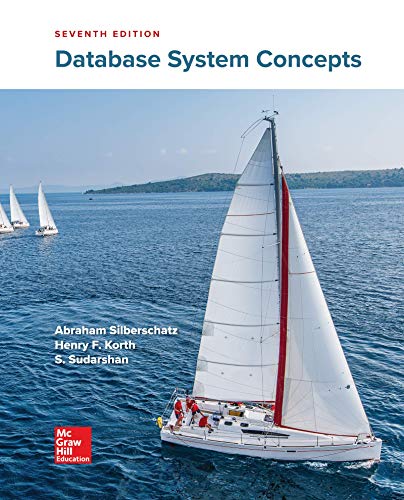
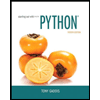
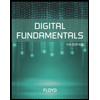
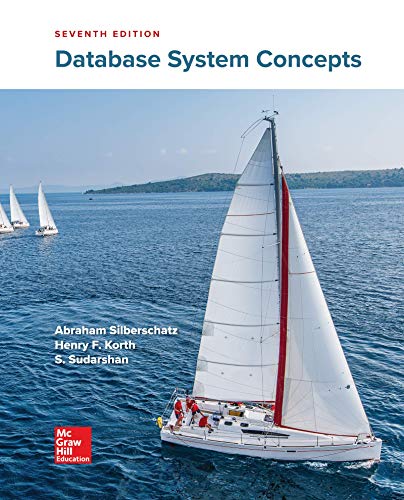
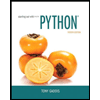
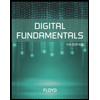
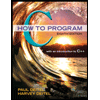
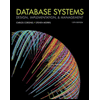
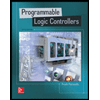