Implement a method concatenate in class LinkedQueue. This method should take all the elements of a queue sourceQ and append them to the end of another queue targetQ. The operation should result in sourceQ being an empty queue. Test this method in the main method of LinkedQueue. package queues; public class LinkedQueue implements Queue { private SinglyLinkedList list = new SinglyLinkedList<>(); // an empty list public LinkedQueue() { } // new queue relies on the initially empty list @Override public int size() { return list.size(); } @Override public boolean isEmpty() { return list.isEmpty(); } @Override public void enqueue(E element) { list.addLast(element); } @Override public E first() { return list.first(); } @Override public E dequeue() { return list.removeFirst(); } public String toString() { return list.toString(); } }
Implement a method concatenate in class LinkedQueue. This method should take all the elements of a queue sourceQ and append them to the end of another queue targetQ. The operation should result in sourceQ being an empty queue. Test this method in the main method of LinkedQueue.
package queues;
public class LinkedQueue<E> implements Queue<E> {
private SinglyLinkedList<E> list = new SinglyLinkedList<>(); // an empty list
public LinkedQueue() { } // new queue relies on the initially empty list
@Override
public int size() { return list.size(); }
@Override
public boolean isEmpty() { return list.isEmpty(); }
@Override
public void enqueue(E element) { list.addLast(element); }
@Override
public E first() { return list.first(); }
@Override
public E dequeue() { return list.removeFirst(); }
public String toString() {
return list.toString();
}
}

Step by step
Solved in 4 steps with 6 images

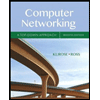
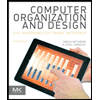
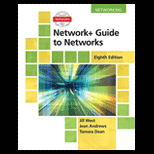
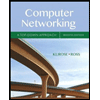
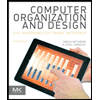
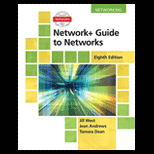
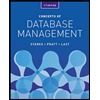
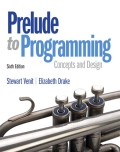
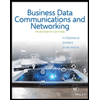