I'm trying to fix a code to give nutritional values of FoodItems but it will do all food items but water properly: class FoodItem: def __init__(self, item_name="None", amount_fat=0.0, amount_carbs=0.0, amount_protein=0.0): self.name = item_name self.fat = amount_fat self.carbs = amount_carbs self.protein = amount_protein def get_calories(self, num_servings): # Calorie formula calories = ((self.fat * 9) + (self.carbs * 4) + (self.protein * 4)) * num_servings; return calories def print_info(self): print(f'Nutritional information per serving of {self.name}:') print(f' Fat: {self.fat:.2f} g') print(f' Carbohydrates: {self.carbs:.2f} g') print(f' Protein: {self.protein:.2f} g') if __name__ == "__main__": item_name = input() if item_name == 'Water' or item_name == 'water': food_item = FoodItem() food_item.print_info() print(f'Number of calories for {1.0:.2f} serving(s): {food_item.get_calories(1.0):.2f}') else: amount_fat = float(input()) amount_carbs = float(input()) amount_protein = float(input()) num_servings = float(input()) food_item = FoodItem(item_name, amount_fat, amount_carbs, amount_protein) food_item.print_info() print(f'Number of calories for {1.0:.2f} serving(s): {food_item.get_calories(1.0):.2f}') print(f'Number of calories for {num_servings:.2f} serving(s): {food_item.get_calories(num_servings):.2f}')
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
I'm trying to fix a code to give nutritional values of FoodItems but it will do all food items but water properly:
class FoodItem:
def __init__(self, item_name="None", amount_fat=0.0, amount_carbs=0.0, amount_protein=0.0):
self.name = item_name
self.fat = amount_fat
self.carbs = amount_carbs
self.protein = amount_protein
def get_calories(self, num_servings):
# Calorie formula
calories = ((self.fat * 9) + (self.carbs * 4) + (self.protein * 4)) * num_servings;
return calories
def print_info(self):
print(f'Nutritional information per serving of {self.name}:')
print(f' Fat: {self.fat:.2f} g')
print(f' Carbohydrates: {self.carbs:.2f} g')
print(f' Protein: {self.protein:.2f} g')
if __name__ == "__main__":
item_name = input()
if item_name == 'Water' or item_name == 'water':
food_item = FoodItem()
food_item.print_info()
print(f'Number of calories for {1.0:.2f} serving(s): {food_item.get_calories(1.0):.2f}')
else:
amount_fat = float(input())
amount_carbs = float(input())
amount_protein = float(input())
num_servings = float(input())
food_item = FoodItem(item_name, amount_fat, amount_carbs, amount_protein)
food_item.print_info()
print(f'Number of calories for {1.0:.2f} serving(s): {food_item.get_calories(1.0):.2f}')
print(f'Number of calories for {num_servings:.2f} serving(s): {food_item.get_calories(num_servings):.2f}')

Step by step
Solved in 4 steps with 3 images

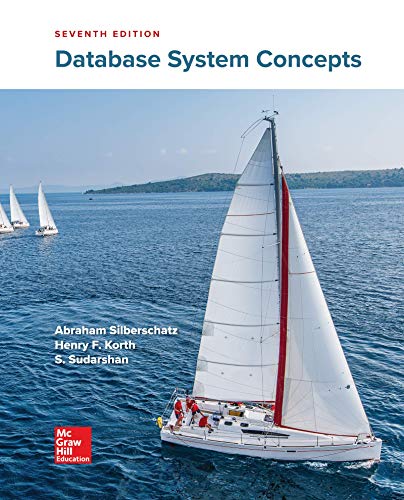
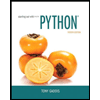
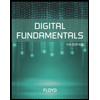
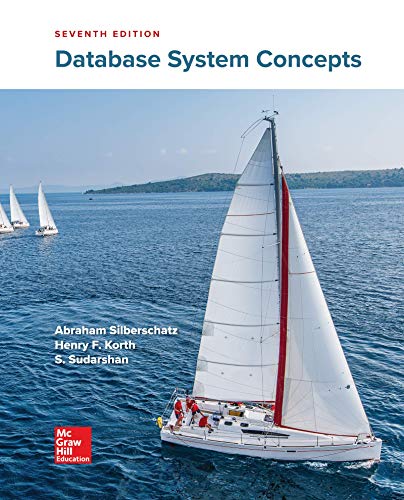
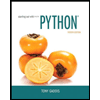
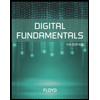
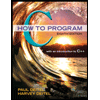
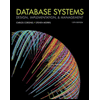
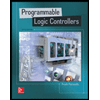