I'm new to Java) and I am really trying to better understand reading code and the logic/ code arrangement. First, I am not completely clear on why I need a seperate Main public class. I'm wondering why I can't include it in the Student class? Also, I made 2 packages that I imported into the CollegeCourse file because my professor wants a 2 java files- CollegeCourse and Student. Now when I compile my CollegeCourse program it compiles with no errors but when I try to run it I get this message- No main methods, JavaFX applications, applets, or MIDIets found in file. I looked online and it says- The java program needs the specific main() method defined in the class that is passed to it. I have this in my public class Main. Does it need to be somewhere else? Please advise and thanks in advance for helping me learn Java. Here are my 3 code pieces- import stud.*; import mai.*; import java.util.*; class CollegeCourse { private String courseID; private int creditHours; private char letterGrade; public String getCourseID() { return courseID; } public int getCreditHours() { return creditHours; } public char getLetterGrade() { return letterGrade; } public void setCourseID(String idNum) { courseID=idNum; } public void setCreditHrs(int ch) { creditHours=ch; } public void setLetterGrade(char gd) { letterGrade=gd; } } ______________________________________________________________________________ package stud; class Student { private int sID; CollegeCourse[]obj = new CollegeCourse[5]; public int getSID() { return sID; } public void setSID(int SIDNum) { sID=SIDNum; } public CollegeCourse getCollegeCourse(int i) { return obj[i]; } public void setCollegeCourse(CollegeCourse c, int i) { obj[i]=c; } } _______________________________________________________________________ //This is where I get confused on what each line is saying package mai; public class Main { public static void main(String args[]) { //create an object of class CollegeCourse but why is it now CollegeCourse c1 = CollegeCourse c1();? CollegeCourse c1 = new CollegeCourse(); c1.setCourseID("CIS 210"); c1.setCreditHrs(3); c1.setLetterGrade('A'); //create object of class Student- same question as above Student s1 = new Student(); s1.setSID(1); //set method that sets the value of one of the Student's CollegeCourses s1.setCollegeCourse(c1,0); CollegeCourse c2 = new CollegeCourse(); //get method that returns one of the Student's CollegeCourses c2 = s1.getCollegeCourse(0); System.out.println("Returned Object Details"); System.out.println("Course ID " + c2.getCourseID()); System.out.println("Credit Hours " + c2.getCreditHours()); System.out.println("Letter Grade " +c2.getLetterGrade()); } }
Control structures
Control structures are block of statements that analyze the value of variables and determine the flow of execution based on those values. When a program is running, the CPU executes the code line by line. After sometime, the program reaches the point where it has to make a decision on whether it has to go to another part of the code or repeat execution of certain part of the code. These results affect the flow of the program's code and these are called control structures.
Switch Statement
The switch statement is a key feature that is used by the programmers a lot in the world of programming and coding, as well as in information technology in general. The switch statement is a selection control mechanism that allows the variable value to change the order of the individual statements in the software execution via search.
( I'm new to Java) and I am really trying to better understand reading code and the logic/ code arrangement.
First, I am not completely clear on why I need a seperate Main public class. I'm wondering why I can't include it in the Student class?
Also, I made 2 packages that I imported into the CollegeCourse file because my professor wants a 2 java files- CollegeCourse and Student.
Now when I compile my CollegeCourse
Please advise and thanks in advance for helping me learn Java.
Here are my 3 code pieces-
import stud.*;
import mai.*;
import java.util.*;
class CollegeCourse
{
private String courseID;
private int creditHours;
private char letterGrade;
public String getCourseID()
{
return courseID;
}
public int getCreditHours()
{
return creditHours;
}
public char getLetterGrade()
{
return letterGrade;
}
public void setCourseID(String idNum)
{
courseID=idNum;
}
public void setCreditHrs(int ch)
{
creditHours=ch;
}
public void setLetterGrade(char gd)
{
letterGrade=gd;
}
}
______________________________________________________________________________
package stud;
class Student
{
private int sID;
CollegeCourse[]obj = new CollegeCourse[5];
public int getSID()
{
return sID;
}
public void setSID(int SIDNum)
{
sID=SIDNum;
}
public CollegeCourse getCollegeCourse(int i)
{
return obj[i];
}
public void setCollegeCourse(CollegeCourse c, int i)
{
obj[i]=c;
}
}
_______________________________________________________________________
//This is where I get confused on what each line is saying
package mai;
public class Main
{
public static void main(String args[])
{
//create an object of class CollegeCourse but why is it now CollegeCourse c1 = CollegeCourse c1();?
CollegeCourse c1 = new CollegeCourse();
c1.setCourseID("CIS 210");
c1.setCreditHrs(3);
c1.setLetterGrade('A');
//create object of class Student- same question as above
Student s1 = new Student();
s1.setSID(1);
//set method that sets the value of one of the Student's CollegeCourses
s1.setCollegeCourse(c1,0);
CollegeCourse c2 = new CollegeCourse();
//get method that returns one of the Student's CollegeCourses
c2 = s1.getCollegeCourse(0);
System.out.println("Returned Object Details");
System.out.println("Course ID " + c2.getCourseID());
System.out.println("Credit Hours " + c2.getCreditHours());
System.out.println("Letter Grade " +c2.getLetterGrade());
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

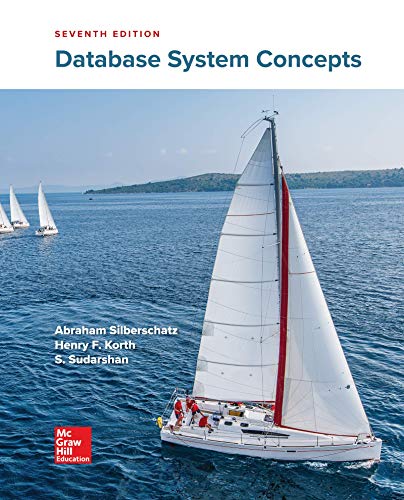
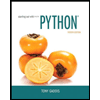
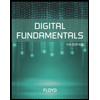
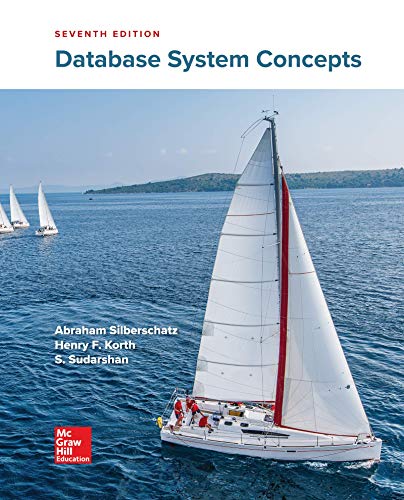
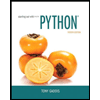
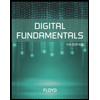
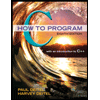
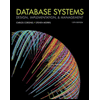
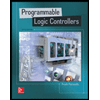