the java file is here. and the question is attached as a photo. i // **************************************************************** // ParseInts.java Authors: Lewis, Loftus & DuVall-Early // Date: 4/17/21 // // Reads a line of text and prints the integers in the line. // // **************************************************************** import java.util.Scanner; public class ParseInts { //--------------------------------------------- // main method //--------------------------------------------- public static void main(String[] args) { int val, sum=0; Scanner scan = new Scanner(System.in); System.out.println("Enter a line of text"); Scanner scanLine = new Scanner(scan.nextLine()); while (scanLine.hasNext()) { val = Integer.parseInt(scanLine.next()); sum += val; } System.out.println("The sum of the integers on this line is " + sum); scan.close(); scanLine.close(); }
the java file is here. and the question is attached as a photo. i // **************************************************************** // ParseInts.java Authors: Lewis, Loftus & DuVall-Early // Date: 4/17/21 // // Reads a line of text and prints the integers in the line. // // **************************************************************** import java.util.Scanner; public class ParseInts { //--------------------------------------------- // main method //--------------------------------------------- public static void main(String[] args) { int val, sum=0; Scanner scan = new Scanner(System.in); System.out.println("Enter a line of text"); Scanner scanLine = new Scanner(scan.nextLine()); while (scanLine.hasNext()) { val = Integer.parseInt(scanLine.next()); sum += val; } System.out.println("The sum of the integers on this line is " + sum); scan.close(); scanLine.close(); }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
the java file is here. and the question is attached as a photo.
i
// ****************************************************************
// ParseInts.java Authors: Lewis, Loftus & DuVall-Early
// Date: 4/17/21
//
// Reads a line of text and prints the integers in the line.
//
// ****************************************************************
import java.util.Scanner;
public class ParseInts
{
//---------------------------------------------
// main method
//---------------------------------------------
public static void main(String[] args)
{
int val, sum=0;
Scanner scan = new Scanner(System.in);
System.out.println("Enter a line of text");
Scanner scanLine = new Scanner(scan.nextLine());
while (scanLine.hasNext())
{
val = Integer.parseInt(scanLine.next());
sum += val;
}
System.out.println("The sum of the integers on this line is " + sum);
scan.close();
scanLine.close();
}
}

Transcribed Image Text:o File Parselnts.java contains a program that does the following:
· Prompts for and reads in a line of input
- Uses a second Scanner to take the input line one token at a time
and parses an integer from each token as it is extracted.
· Sums the integers.
- Prints the sum.
• Save Parselnts to your directory and compile and run it.
If you give it the following input : 10 20 30 40 it should print "The
sum of the integers on the line is 100".
Try some other inputs as well. Now try a line that contains both
integers and other values, e.g., We have 2 dogs and 1 cat. You
should get a NumberFormatException when it tries to call
Integer.parselnt on "We", which is not an integer.
One way around this is to put the loop that reads the input inside a try
and catch the NumberFormatException but not do anything with it. This
way if it's not an integer it doesn't cause an error; it goes to the
exception handler, which does nothing. Do this as follows:
Modify the program to add a try statement that encompasses the
entire while loop. The try and opening { should go before the while,
and the catch after the loop body. Catch a NumberFormatException
and have an empty body for the catch.
· Compile and run the program and enter a line with mixed integers
and other values. You should find that it stops summing at the first
non-integer, so the line above will produce a sum of 0, and the line
"1 fish 2 fish" will produce a sum of 1. This is because the entire
loop is inside the try, so when an exception is thrown the loop is
terminated.
· To make it continue, move the try and catch inside the loop. Now
when an exception is thrown, the next statement is the next
iteration of the loop, so the entire line is processed. The dogs-and-
cats
input should now give a sum of 3, as should the fish input.
Paint X lite
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
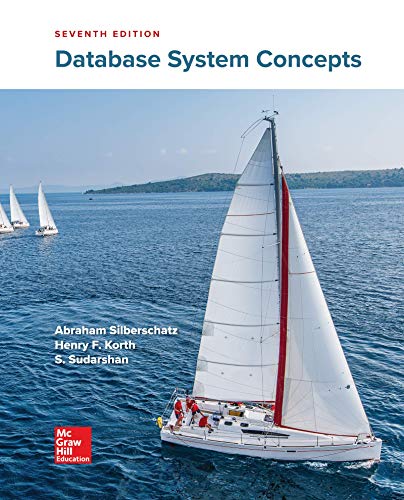
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
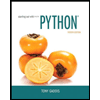
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
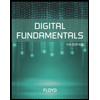
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
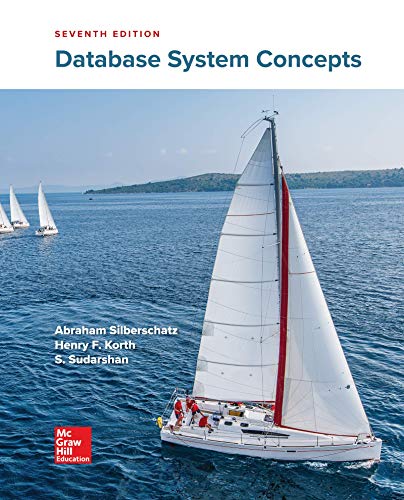
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
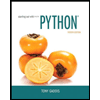
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
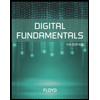
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
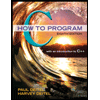
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
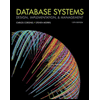
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
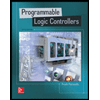
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education