I'm having issues with Python. I need this file to read my text file for the Baseball teams. But I need it to give me a answer of how many times they one by counting and post it 1 time insted of 300. Also I'm pretty new to python so please to not to blow my mind.
I'm having issues with Python. I need this file to read my text file for the Baseball teams. But I need it to give me a answer of how many times they one by counting and post it 1 time insted of 300. Also I'm pretty new to python so please to not to blow my mind.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
I'm having issues with Python. I need this file to read my text file for the Baseball teams. But I need it to give me a answer of how many times they one by counting and post it 1 time insted of 300. Also I'm pretty new to python so please to not to blow my mind.

Transcribed Image Text:à Python 3.8.5 Shell
File Edit Shell Debug Options Window Help
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
Your team the New York Yankees won the world seires 1 times between 1903 and 2000
The New York Yankees didnt win any world series.
>>> |
![Là LaForce page 404 #10.py - D:\Python\LaForce page 404 #10.py (3.8.5)
File Edit
Format Run Options Window Help
1 def main () :
2
3
try:
4
5
file=open ('World champs.txt','r')
6
Winnerstile.readlines ()
8
file.close ()
10
11
for Champs in range (len (Winners)):
13
Winners [Champs]=Winners [Champs].rstrip ('\n')
14
15
Team Name=input ('Enter the team you want to see:
')
16
17
if Team Name in Winners:
18
Win=0
for Name in Winners:
if Name==Team Name:
Win = +1
19
20
21
22
print ('Your team the',Team Name, 'won the world seires' ,Win, 'times between l903 and 2000')
23
else:
24
print ('The',Team Name, 'didnt win any world series.')
25
26
except IOError:
print ('File not found.')
27
28
except IndexError:
print ('Index error')
29
30
31 main ()
32
H H 1 LLR LL LL2 222N 22 222 233 3](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc43e03e1-b353-4d50-a726-bab1a8232fef%2F190b339d-3734-422f-bfe3-28fa81202bae%2Fk4lynkn_processed.png&w=3840&q=75)
Transcribed Image Text:Là LaForce page 404 #10.py - D:\Python\LaForce page 404 #10.py (3.8.5)
File Edit
Format Run Options Window Help
1 def main () :
2
3
try:
4
5
file=open ('World champs.txt','r')
6
Winnerstile.readlines ()
8
file.close ()
10
11
for Champs in range (len (Winners)):
13
Winners [Champs]=Winners [Champs].rstrip ('\n')
14
15
Team Name=input ('Enter the team you want to see:
')
16
17
if Team Name in Winners:
18
Win=0
for Name in Winners:
if Name==Team Name:
Win = +1
19
20
21
22
print ('Your team the',Team Name, 'won the world seires' ,Win, 'times between l903 and 2000')
23
else:
24
print ('The',Team Name, 'didnt win any world series.')
25
26
except IOError:
print ('File not found.')
27
28
except IndexError:
print ('Index error')
29
30
31 main ()
32
H H 1 LLR LL LL2 222N 22 222 233 3
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
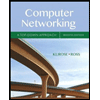
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
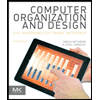
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
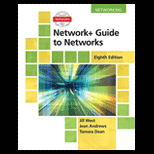
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
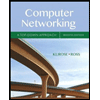
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
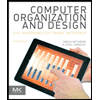
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
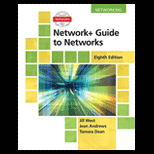
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
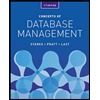
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
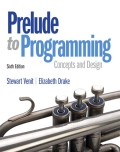
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
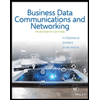
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY