If this code was written in C++, what keyword would need to precede the declaration of the draw() method in the Shape class in order to allow polymorphism?
If this code was written in C++, what keyword would need to precede the declaration of the draw() method in the Shape class in order to allow polymorphism?
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Complete the code to create a polymorphism situation.

Transcribed Image Text:If this code was written in C++, what keyword would need to precede the declaration of the draw() method in the Shape class in order to allow polymorphism?

Transcribed Image Text:**Text Description:**
*Shape* is a superclass with subclasses *Circle, Rect*, etc. Assume that *Shape* has a `draw()` method that does nothing, and that *Circle* and *Rect* each have a `draw()` method that draws a circle and rectangle, respectively.
Consider the following code:
```java
Circle c1 = new Circle(Color.BLUE, 100,100, 50);
Rect r1 = new Rect (Color.GREEN, 200,100, 50,50);
c1.draw();
r1.draw();
Shape s1 = c1, s2 = r1, s3;
s1.draw();
s2.draw();
```
**Explanation:**
This segment of code demonstrates the concepts of inheritance and polymorphism in object-oriented programming. The `Shape` class acts as a base class, while `Circle` and `Rect` are derived classes, each implementing a specific version of the `draw()` method.
1. **Declarations:**
- `Circle c1 = new Circle(Color.BLUE, 100,100, 50);`
- Creates a new `Circle` object `c1` with the color blue and specific dimensions.
- `Rect r1 = new Rect (Color.GREEN, 200,100, 50,50);`
- Creates a new `Rect` object `r1` with the color green and specific dimensions.
2. **Method Invocation:**
- `c1.draw();` and `r1.draw();` call the `draw()` method for each respective object, invoking the action to draw a circle and a rectangle.
3. **Polymorphism:**
- `Shape s1 = c1, s2 = r1, s3;`
- `s1` and `s2` are declared as `Shape` type references that point to `c1` and `r1`, respectively.
- `s1.draw();` and `s2.draw();`
- Although `s1` and `s2` are of type `Shape`, they call the overridden `draw()` methods in `Circle` and `Rect`, demonstrating polymorphism.
This code block illustrates how object-oriented programming leverages class hierarchies and method overriding to achieve flexible design patterns.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
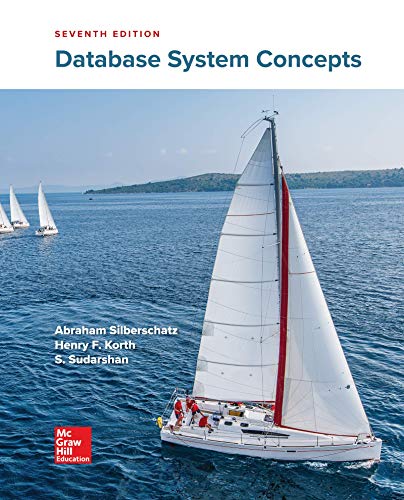
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
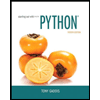
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
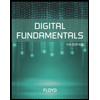
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
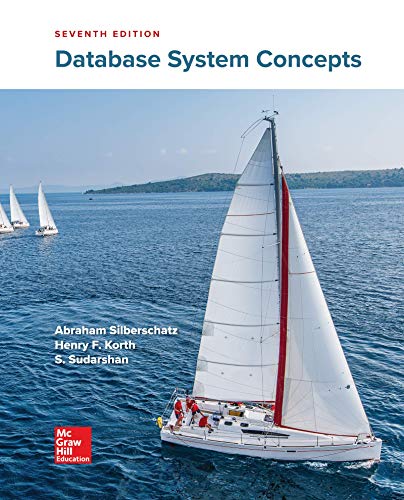
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
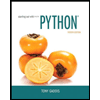
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
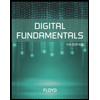
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
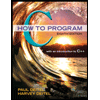
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
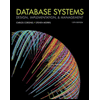
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
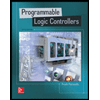
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education