This assignment creates a GradeBook class that manages a list of quiz grades, computes the average and maintains a count of GradeBook objects, one per student. Your program needs to support just two students. Here's how your program should start: $ python3 hwk7.py Please enter the name for Student 1: Mary There are 1 students in the GradeBook. Please enter the name for Student 2: Hadalam There are 2 students in the GradeBook. Grade Book 0: Exit 1: Enter quiz grade for Student 1 2: Enter quiz grade for Student 2 3: Display current grades for all students Please enter a choice: When the program first starts up, the user is queried to enter the names for two students. A count of how many GradeBook objects have been created is maintained in a class variable within GradeBook. This variable is to be updated in the constructor and used for the display of the count. See class vs instance variables for reference. Your class should include the following methods: • a constructor - __init__(self, name) • The construct should two instance variables, self.name and self.grades. The name parameter is stored in self.name and self.grades should be created as an empty list. quizScore(self, score) . This method is called to add the score to self.grades . currentAverage(self) • This method computes the average of the scores in self.grades and returns the result Class variables: • count . this variable is used to count how many GradeBook objects have been created . GradeBook.count should be initialized to 0 outside of any of the methods. GradeBook.count should be incremented by one inside of the constructor Instance variables • self.name the name of the student • self.grades - the list of quiz scores for the student Each GradeBook object (one per student) needs to be created before the while loop. Be sure to include the student's name when creating the object. In order to test your GradeBook class, you'll implement a menu driven interface, providing the choices as shown in the image above. When the user chooses to enter a quiz grade for Student 1, Student 1's quiz list should be updated. When the user chooses to enter a quiz grade for Student 2, Student 2's quiz list should be updated. When the user chooses to display the current grades for all students, the averages for Student 1 is computed via currentAverage method and returned to be printed in the main program (not in currentAverage), and then the same is done with Student 2. The name of each student is available as instance variable in each object, e.g. student 1.name, and should be included in the display. That is, the names should not come from variables outside of the objects. For the purposes of this assignment, the number of students is fixed at two. You do not need to maintain a list of objects. Just use a separate variable for each object- e.g. student1 and student2. If you have implemented the instance and class variables correctly, entering a score for one student should not change the other student's average. If that happens, you are probably using a class variable instead of an instance variable for the list of quiz scores.
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

If I wanted to check scores before they were any value entered how would I construct that? For example, after entering students names in the gradebook, I check their grades and the grades should say 0.0 because no value has been entered yet.
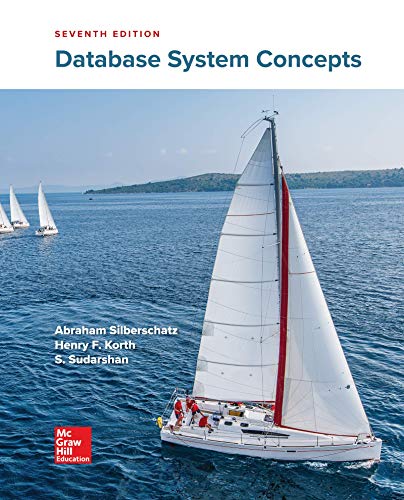
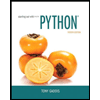
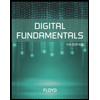
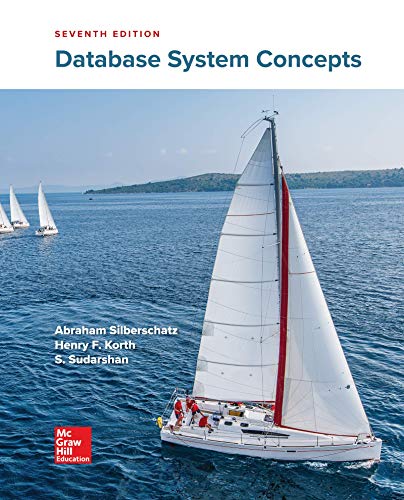
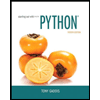
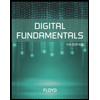
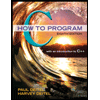
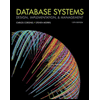
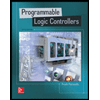