I wrote three different types of code (gate level modeling, dataflow level modeling and behavioral modeling). below I have attached the three codes hoping you could write a comment on each line of code describing what each line does and then explain how each code is different. (Gate level modeling) module project_gate1 (q, qbar, s, r, clk ); //(EXAMPLE COMMENTS WOULD GO HERE FOR EACH LINE) /this is to model a SR Flip-flop in gate level modeling input s, r, clk; output q, qbar; wire nand1_out; wire nand2_out; nand(nand1_out, s, clk); nand(nand2_out, r, clk); nand(q, nand1_out, qbar); nand(qbar, nand2_out, q); endmodule (Data flow modeling) module srff_dataflow(q, qbar, s, r, clk); output q, qbar; input s, r, clk; wire s_bar, r_bar; assign s_bar = ~(s & clk & qbar); assign r_bar = ~(r & clk & q); assign q = s_bar; assign qbar = r_bar; endmodule (Behavioral level modeling) module srff_07 (s, r, clk, q, qbar); input s, r, clk; output q, qbar; reg q, qbar; always@(posedge clk) begin if (s == 1 && r == 0) begin q <= 1; qbar <= 0; end else if (s == 0 && r == 1) begin q <= 0; qbar <= 1; end else if (s==0 && r==0) begin q <= q; qbar <= qbar; end end
I wrote three different types of code (gate level modeling, dataflow level modeling and behavioral modeling).
below I have attached the three codes hoping you could write a comment on each line of code describing what each line does and then explain how each code is different.
(Gate level modeling)
module project_gate1 (q, qbar, s, r, clk ); //(EXAMPLE COMMENTS WOULD GO HERE FOR EACH LINE)
/this is to model a SR Flip-flop in gate level modeling
input s, r, clk;
output q, qbar;
wire nand1_out;
wire nand2_out;
nand(nand1_out, s, clk);
nand(nand2_out, r, clk);
nand(q, nand1_out, qbar);
nand(qbar, nand2_out, q);
endmodule
(Data flow modeling)
module srff_dataflow(q, qbar, s, r, clk);
output q, qbar;
input s, r, clk;
wire s_bar, r_bar;
assign s_bar = ~(s & clk & qbar);
assign r_bar = ~(r & clk & q);
assign q = s_bar;
assign qbar = r_bar;
endmodule
(Behavioral level modeling)
module srff_07 (s, r, clk, q, qbar);
input s, r, clk;
output q, qbar;
reg q, qbar;
always@(posedge clk)
begin
if (s == 1 && r == 0)
begin
q <= 1;
qbar <= 0;
end
else if (s == 0 && r == 1)
begin
q <= 0;
qbar <= 1;
end
else if (s==0 && r==0)
begin
q <= q;
qbar <= qbar;
end
end

Gates can be defined in such a way that they are electronic devices or logical components that perform basic logical functions based on Boolean algebra.
Step by step
Solved in 6 steps

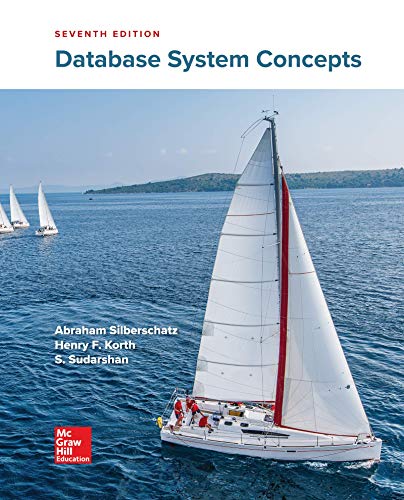
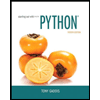
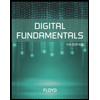
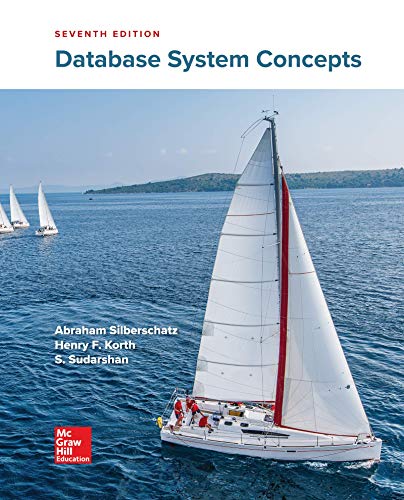
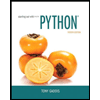
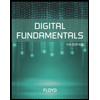
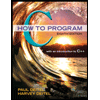
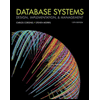
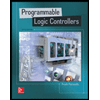